In the bustling world of digital marketing, automation is no longer a luxury—it's a necessity. Promotional campaigns, whether for email marketing, customer outreach, or internal updates, need to be timely, relevant, and consistent. Manually managing these campaigns can be time-consuming and prone to errors. Enter Python—a versatile programming language that empowers businesses to streamline email workflows, enhance outreach, and automate promotional campaigns.
This guide is tailored for programming enthusiasts, small business owners, and budding tech-savvy marketers looking to leverage Python for marketing automation. Whether you're a programming geek or just dipping your toes into coding, this blog will provide you with a practical roadmap to automating your promotional campaigns with Python scripts. Let’s dive in!
Why Python for Automating Promotional Campaigns?
Python has gained immense popularity among programming geeks (programgeeks) and marketers alike due to its simplicity, robust libraries, and ease of integration with various platforms. Here’s why Python stands out:
- Ease of Learning: Python’s syntax is intuitive, making it an excellent choice for beginners and seasoned programmers alike.
- Rich Libraries: Python offers libraries like smtplib, email, and schedule for automation and integration with email services.
- Scalability: Whether you're managing small outreach campaigns or large-scale email blasts, Python scales effortlessly.
- Community Support: Python boasts a massive community of geeks who contribute to its ecosystem, ensuring a wealth of resources and support.
Setting Up Your Environment
Before diving into automation, you need to set up your development environment. Here’s how:
Step 1: Install Python
Download and install Python from the official Python website. During installation, check the box to add Python to your system’s PATH.
Step 2: Install Necessary Libraries
Use pip
, Python’s package manager, to install essential libraries:
pip install smtplib email schedule pandas
- smtplib: For sending emails via SMTP.
- email: For constructing and handling email messages.
- schedule: For scheduling tasks.
- pandas: For managing and processing email lists.
Step 3: Choose an IDE
Popular choices include Visual Studio Code, PyCharm, or even Jupyter Notebook for experimentation.
Step-by-Step: Automating Promotional Email Campaigns
Automating promotional email campaigns involves several steps, from managing email lists to crafting personalized messages. Let’s break it down:
Step 1: Managing Your Email List
Before sending out emails, organize your recipient list using a .csv
file. Python’s pandas library makes it easy to read and process data.
Example CSV structure:
Name | Last Purchase | Offer | |
---|---|---|---|
John Doe | john@example.com | 2023-12-15 | 20% Off |
Jane Smith | jane@example.com | 2023-12-10 | Free Gift |
Load and process the data with Python:
import pandas as pd
# Load the email list
email_data = pd.read_csv('email_list.csv')
# Preview the data
print(email_data.head())
Step 2: Crafting Personalized Emails
Personalization increases engagement. Use Python's email.mime modules to create dynamic emails.
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def create_email(recipient_name, recipient_email, offer):
msg = MIMEMultipart()
msg['From'] = 'your_email@example.com'
msg['To'] = recipient_email
msg['Subject'] = 'Exclusive Offer Just for You!'
body = f"""
Hi {recipient_name},
Thank you for being a valued customer. We're excited to offer you a special promotion: {offer}.
Click here to claim your offer: [Insert Link]
Best Regards,
Your Company
"""
msg.attach(MIMEText(body, 'plain'))
return msg.as_string()
Step 3: Sending Emails with SMTP
Python’s smtplib library connects to SMTP servers to send emails.
import smtplib
def send_email(message):
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login('your_email@example.com', 'your_password')
server.sendmail('your_email@example.com', message['To'], message)
server.quit()
Step 4: Automating the Process
Use the schedule library to automate sending emails at specific intervals.
import schedule
import time
def job():
for index, row in email_data.iterrows():
email = create_email(row['Name'], row['Email'], row['Offer'])
send_email(email)
print(f"Email sent to {row['Email']}")
# Schedule the job
schedule.every().day.at("10:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
Advanced Use Cases of Python for Promotional Campaigns
Python is not just for basic email automation—it can drive advanced, high-impact workflows. Below are some detailed use cases where Python helps businesses supercharge their operations, providing both technical insights and actionable steps for each scenario.
1. Customer Outreach Automation with Python
Goal: Re-engage dormant customers by analyzing their purchase behavior and sending personalized campaigns to draw them back.
Why It Matters:
Dormant customers represent untapped potential. By analyzing their purchase history and crafting targeted messages, you can rekindle interest and increase conversions.
Implementation Steps:
-
Analyze Purchase History:
- Use transaction logs to identify inactive customers.
- Filter based on inactivity period (e.g., no purchases in 3+ months).
- Tools like pandas can help process purchase history in bulk.
import pandas as pd # Load purchase data data = pd.read_csv('purchase_history.csv') # Filter dormant customers dormant_customers = data[data['LastPurchaseDate'] < '2023-09-01'] print(dormant_customers)
-
Personalized Messaging: Craft custom email templates with exclusive offers, such as discounts or loyalty points.
from email.mime.text import MIMEText def create_outreach_email(name, email, last_purchase): body = f""" Hi {name}, We noticed you haven't shopped with us since {last_purchase}. To welcome you back, here's a special offer: 20% OFF your next purchase! Use the code WELCOME20 at checkout. Looking forward to seeing you again! - Your Brand Team """ msg = MIMEText(body, 'plain') msg['Subject'] = "We Miss You! Here's 20% Off" msg['To'] = email return msg
-
Sending the Campaign: Automate sending emails to this segment using smtplib.
for index, row in dormant_customers.iterrows(): email = create_outreach_email(row['Name'], row['Email'], row['LastPurchaseDate']) send_email(email)
-
Track Results: Use email tracking tools to monitor open rates, click-through rates, and conversions. Adjust your strategy based on the feedback.
2. Automate Internal Updates
Goal: Keep your team informed by sending daily or weekly reports automatically, pulling real-time data from spreadsheets or APIs.
Why It Matters:
Manual reporting can be error-prone and time-intensive. Automating internal updates ensures timely, accurate, and consistent information delivery.
Implementation Steps:
-
Data Gathering:
- Pull data from spreadsheets using Python's
pandas
library. - Integrate with APIs (e.g., Google Analytics API, HubSpot API) for real-time metrics.
import pandas as pd # Load data from a spreadsheet report_data = pd.read_excel('team_report.xlsx') # Summarize key metrics summary = report_data.describe()
- Pull data from spreadsheets using Python's
-
Generate a Report: Use Python to compile a formatted report, either as plain text or HTML.
from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText def create_report_email(metrics_summary, recipient_email): msg = MIMEMultipart() msg['From'] = 'your_email@example.com' msg['To'] = recipient_email msg['Subject'] = "Weekly Performance Report" body = f""" Hello Team, Here's this week's performance summary: {metrics_summary} Let’s aim for even better results next week! Regards, Automation Bot """ msg.attach(MIMEText(body, 'plain')) return msg
-
Automate Delivery: Schedule the script to run at specific intervals using the schedule library or cron jobs.
import schedule import time def send_reports(): email = create_report_email(summary, 'team@example.com') send_email(email) print("Report sent!") schedule.every().monday.at("09:00").do(send_reports) while True: schedule.run_pending() time.sleep(1)
-
Enhancements:
- Add visualizations to the report using matplotlib.
- Send reports via Slack or Microsoft Teams using their respective APIs for easier team consumption.
3. Create Outreach Automation Tools with Python
Goal: Build custom tools to streamline complex workflows, such as lead generation, follow-ups, and campaign tracking.
Why It Matters:
Out-of-the-box solutions often lack flexibility for unique business needs. Custom tools built with Python allow you to design processes tailored to your goals.
Implementation Steps:
-
Lead Generation Automation:
- Scrape data from social media or business directories using tools like BeautifulSoup or Selenium.
- Save leads in a structured format for further processing.
from bs4 import BeautifulSoup import requests # Scrape leads from a website url = 'https://example.com/leads' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') leads = [] for contact in soup.find_all('div', class_='contact-card'): name = contact.find('h3').text email = contact.find('a', class_='email').text leads.append({'Name': name, 'Email': email}) print(leads)
-
Automated Follow-Ups:
- Use Python to track whether a lead has responded.
- Automate reminders or second-touch campaigns for non-responders.
# Track responses lead_status = {'john@example.com': 'Pending', 'jane@example.com': 'Responded'} for email, status in lead_status.items(): if status == 'Pending': follow_up_email = create_outreach_email('John', email, 'Special Offer') send_email(follow_up_email)
-
Campaign Tracking:
- Integrate with Google Sheets API or Airtable API to log campaign performance (e.g., opens, clicks).
- Automate periodic updates for review.
import gspread from oauth2client.service_account import ServiceAccountCredentials # Authenticate with Google Sheets scope = ['https://spreadsheets.google.com/feeds'] creds = ServiceAccountCredentials.from_json_keyfile_name('credentials.json', scope) client = gspread.authorize(creds) # Update campaign data sheet = client.open("Campaign Report").sheet1 sheet.update('A2', 'Email Sent')
-
Scalability:
- Host the tool on a cloud platform (e.g., AWS, Heroku) for reliability and availability.
- Add a user interface using frameworks like Flask or Django to make the tool accessible to non-technical users.
Advanced use cases like customer outreach automation, internal updates, and custom outreach tools unlock the full potential of Python for marketing and operational excellence. By leveraging Python’s versatility and rich ecosystem, you can enhance customer engagement, improve team communication, and scale your business operations seamlessly.
At Prateeksha Web Design, we specialize in crafting automated solutions tailored to your business needs. Whether it’s creating outreach automation tools or streamlining your workflows, our expertise ensures you stay ahead of the curve. Let’s build the future of your business together!
Best Practices for Python Email Marketing Automation
- Use a Professional Email Service: Integrate with platforms like SendGrid or Mailgun for higher deliverability.
- Test Your Campaigns: Always test your scripts with dummy data before a full-scale rollout.
- Respect Privacy: Comply with GDPR and CAN-SPAM regulations to maintain customer trust.
- Monitor Performance: Use tools to track email open rates and optimize campaigns based on data.
Why Choose Prateeksha Web Design?
At Prateeksha Web Design, we specialize in crafting automated solutions for businesses, helping you leverage technologies like Python to streamline your marketing efforts. Whether it’s building custom scripts or integrating email automation tools, our expertise ensures that your promotional campaigns are efficient, effective, and engaging.
Conclusion
By automating promotional campaigns with Python scripts, businesses can save time, reduce errors, and enhance customer engagement. Whether you're a geek program enthusiast, a small business owner, or a marketer, Python offers endless possibilities for email campaign automation and beyond.
Ready to transform your marketing strategy? Reach out to Prateeksha Web Design to discover how our expertise can take your campaigns to the next level.
Interested in learning more? Contact us today. For more insights on email marketing, check out Mailchimp's Guide to Email Marketing and to stay updated on Python tools, visit the Real Python website. For advanced automation techniques, explore Zapier's Automation Resources.
FAQs
FAQs
1. What is the purpose of using Python for marketing automation? Python is used for marketing automation due to its simplicity, robust libraries, and ability to integrate with various platforms. It allows businesses to streamline email workflows, enhance customer outreach, and automate promotional campaigns efficiently.
2. What libraries do I need to install to get started with Python email automation?
To start with Python email automation, you need to install libraries such as smtplib
, email
, schedule
, and pandas
using Python's package manager, pip
.
3. How can I ensure my automated emails are compliant with regulations? To ensure compliance with regulations like GDPR and CAN-SPAM, always include a clear opt-out option in your emails, avoid misleading subject lines, and maintain honesty in your content. Regularly update your email list to remove inactive subscribers.
4. What are some advanced use cases for Python in promotional campaigns? Advanced use cases include automating customer outreach to re-engage dormant customers, automating internal updates and reports for team communication, and creating custom outreach tools for lead generation and follow-ups.
5. How can I track the performance of my automated email campaigns? You can track the performance of your automated email campaigns by using email tracking tools to monitor open rates, click-through rates, and conversions. Analyzing this data helps refine your strategies and improve future campaigns.
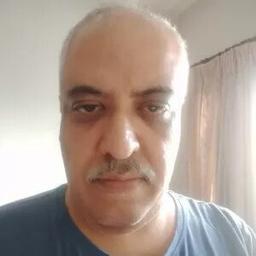