In the fast-paced digital age, the demand for efficiency has skyrocketed. Whether you're filling out job applications, submitting client data, or performing daily repetitive tasks, online form filling can be a time-consuming chore. Imagine how much easier life would be if you could automate these tasks using Python—a versatile and beginner-friendly programming language. This blog dives into the exciting world of Python for automated data entry, exploring how to automate web forms, simplify workflows, and save valuable time.
If you're a curious programming geek, this guide is tailored to you—engaging, detailed, and beginner-friendly, with just the right balance of depth and accessibility.
What is Online Form Automation?
Online form automation refers to the process of using technology—specifically, scripts or tools—to automatically input data into web-based forms. Instead of manually typing out information into each field, automation handles these repetitive tasks for you. It’s like having a digital assistant that does the tedious work while you focus on more important things.
How Online Form Automation Works
At a high level, online form automation involves:
- Accessing the Webpage: Navigating to the web page that contains the form.
- Identifying Form Elements: Detecting input fields like text boxes, checkboxes, or dropdowns using their HTML attributes (like
id
orname
). - Filling the Form: Inputting predefined data into these fields using a script.
- Submitting the Form: Clicking the "Submit" button or triggering the form submission through code.
Examples of Online Forms You Can Automate
- Login Forms: Automating username and password entry.
- Survey Submissions: Automatically inputting data into long surveys.
- Job Applications: Quickly applying to multiple job postings with consistent information.
- Registration Forms: Filling out user details for event sign-ups or account creations.
- E-commerce Tasks: Automating product uploads or order form submissions.
Benefits of Online Form Automation
- Speeds Up Repetitive Tasks: Manual form filling is time-consuming. Automating the process can save hours, especially for bulk submissions.
- Minimizes Errors: Humans can make typos or inconsistencies. Scripts ensure data is entered accurately every time.
- Improves Productivity: By handling the repetitive tasks, you free up mental bandwidth for creative or strategic work.
- Reduces Frustration: Long, tedious forms can be a pain. Automation makes them a breeze.
- Increases Scalability: Whether it’s 10 forms or 1,000, automation handles them with consistent speed and precision.
Real-Life Scenario
Imagine you’re a student applying for internships. Each application form asks for the same basic details—name, address, phone number, etc. Instead of filling out these forms 50 times, a Python script can input the data automatically for each application.
Or consider a small business owner managing e-commerce. Updating prices or adding new products to an online store can be tedious. Python form automation can handle such tasks efficiently, leaving you more time to focus on growing your business.
Why Python for Online Form Automation?
Python is the ideal choice for online form automation for several reasons. Let’s break it down in detail:
1. Beginner-Friendly
Python’s syntax is intuitive and reads like plain English. Even if you’ve never coded before, Python is a great starting point. For example, instead of complex code, you can interact with a web form using straightforward commands like find_element_by_id()
or send_keys()
.
Here’s a quick example of Python's simplicity:
name_field = driver.find_element_by_name("name")
name_field.send_keys("John Doe")
This code snippet tells Python to find the "Name" field on a form and input "John Doe." It’s simple, readable, and beginner-friendly.
2. Powerful Libraries
Python offers a treasure trove of libraries that make automation seamless. Let’s take a closer look at a few key ones:
- Selenium: Ideal for web browser automation. Selenium can handle dynamic websites that rely on JavaScript. Learn more about Selenium here.
- Requests: Perfect for sending HTTP requests directly to web pages. It’s lightweight and efficient for static forms.
- Beautiful Soup: Great for parsing HTML and extracting form data, helping you understand the structure of a webpage.
- PyAutoGUI: Helps automate GUI interactions, like clicking buttons or typing.
Each library comes with robust documentation and tutorials, making it easy for beginners and advanced users alike.
3. Cross-Platform Compatibility
Python scripts work on all major operating systems—Windows, macOS, and Linux. This versatility means you can automate tasks regardless of your setup. Plus, Python libraries often have platform-specific drivers (like ChromeDriver or GeckoDriver) to ensure smooth execution.
For example:
- On Windows, you can install ChromeDriver for Selenium and start automating forms on Google Chrome.
- On macOS, the same script will work with minimal changes, provided you’ve installed the correct driver.
4. Community Support
Python has one of the largest and most active programming communities in the world. If you’re ever stuck, forums like ProgramGeeks, Stack Overflow, or the Python subreddit are filled with experienced developers ready to help.
Resources like:
- Tutorials on YouTube.
- Beginner-friendly guides on blogs like Geeks Program or Programming Geeks.
- Free courses on platforms like Coursera and edX.
Whether you're debugging a tricky issue or learning a new automation technique, you'll never feel alone.
5. Cost-Effective
Python is open-source and free to use. Most of its libraries are also free, meaning you don’t need to invest in expensive software to automate tasks.
For small businesses or freelancers, this makes Python a practical solution for reducing manual effort without stretching your budget.
Tools and Libraries for Automating Online Forms With Python
To master Python for automated data entry, you’ll need to get familiar with some essential tools and libraries. These libraries simplify the process of automating online forms, making it possible to interact with web pages like a human or through direct HTTP requests. Below, we’ll explore each tool in detail.
1. Selenium
What It Is
Selenium is one of the most popular tools for browser automation. It allows Python scripts to control a web browser, interact with elements on a web page, and perform actions such as clicking buttons, entering text, and submitting forms. It’s particularly effective for dynamic websites that use JavaScript.
Why It’s Useful
- Handles Dynamic Content: Websites today often rely on JavaScript for loading elements dynamically. Selenium can wait for these elements to load and interact with them seamlessly.
- Mimics Human Interaction: Selenium can perform tasks like scrolling, hovering, or dragging elements, making it feel like a real user is interacting with the page.
- Cross-Browser Compatibility: Works with popular browsers like Chrome, Firefox, Safari, and Edge.
How to Install
To get started with Selenium, install it using pip:
pip install selenium
Example Usage
Here’s a simple script to log in to a website using Selenium:
from selenium import webdriver
from selenium.webdriver.common.by import By
# Set up WebDriver
driver = webdriver.Chrome(executable_path='path_to_chromedriver')
# Open the webpage
driver.get('https://example.com/login')
# Find elements and fill the form
username = driver.find_element(By.ID, 'username')
password = driver.find_element(By.ID, 'password')
username.send_keys('your_username')
password.send_keys('your_password')
# Submit the form
login_button = driver.find_element(By.ID, 'login-button')
login_button.click()
driver.quit()
Browser Drivers
To use Selenium, you’ll need a browser-specific driver, such as:
- ChromeDriver: Download ChromeDriver
- Firefox: Download GeckoDriver from Mozilla’s official site
2. Requests
What It Is
The Requests
library is used for sending HTTP/HTTPS requests to web servers. Unlike Selenium, which automates browsers, Requests interacts directly with web servers, making it lightweight and fast for simple tasks.
Why It’s Useful
- Form Submissions: Ideal for submitting forms where fields can be sent using POST or GET methods.
- Efficient: Perfect for static forms that don’t require a browser to load content.
- Handles Authentication: Easily send credentials for forms that require login.
How to Install
Install Requests using pip:
pip install requests
Example Usage
Submitting a form with POST data:
import requests
# Define the URL and form data
url = 'https://example.com/form'
data = {
'username': 'your_username',
'password': 'your_password'
}
# Send the POST request
response = requests.post(url, data=data)
# Check the response
print(response.status_code)
print(response.text)
Limitations
Requests cannot handle JavaScript-based forms. For such cases, you’ll need a tool like Selenium.
3. Beautiful Soup
What It Is
Beautiful Soup is a library used for parsing HTML and XML documents. It’s particularly helpful for web scraping and analyzing form structures to determine how to interact with them programmatically.
Why It’s Useful
- HTML Parsing: Extract form elements, such as input fields or buttons, from the HTML.
- Supports Complex Structures: Handles nested tags and poorly structured HTML with ease.
- Perfect for Preprocessing: Use it to analyze forms before automating their submission.
How to Install
Install Beautiful Soup using pip:
pip install beautifulsoup4
Example Usage
Extracting form fields:
from bs4 import BeautifulSoup
import requests
# Fetch the HTML content
response = requests.get('https://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
# Find all input fields
input_fields = soup.find_all('input')
for field in input_fields:
print(f"Name: {field.get('name')}, Type: {field.get('type')}")
Best Use Case
Use Beautiful Soup alongside Requests to analyze and scrape static web pages before submitting forms programmatically.
4. PyAutoGUI
What It Is
PyAutoGUI is a library for automating GUI (Graphical User Interface) tasks. It’s perfect for scenarios where you need to automate desktop-based interactions, such as typing into a browser or clicking buttons.
Why It’s Useful
- Browser Interaction: Automates tasks like opening a browser, clicking fields, or typing text.
- Custom Applications: Works well for non-web forms, such as desktop software or virtual environments.
- User-Friendly: Offers simple commands like
click()
,write()
, andmoveTo()
.
How to Install
Install PyAutoGUI using pip:
pip install pyautogui
Example Usage
Automating mouse and keyboard inputs:
import pyautogui
# Move the mouse to coordinates
pyautogui.moveTo(100, 200)
# Click the mouse
pyautogui.click()
# Type text
pyautogui.write('Hello, World!', interval=0.1)
Limitations
PyAutoGUI lacks web-specific functionality and should be used only when browser-based libraries like Selenium are insufficient.
5. Browser-Specific Drivers
To use Selenium, you’ll need a driver compatible with your browser. These drivers act as a bridge between your Selenium script and the browser.
Examples of Drivers
- ChromeDriver: Works with Google Chrome. Download it here.
- GeckoDriver: Works with Mozilla Firefox. Download it from Mozilla's GitHub.
- Edge WebDriver: Compatible with Microsoft Edge. Available here.
How to Set Up a Driver
- Download the driver for your browser.
- Add the driver path to your Python script:
driver = webdriver.Chrome(executable_path='path_to_chromedriver')
Pro Tip
Keep your browser and driver versions in sync to avoid compatibility issues.
Step-by-Step Guide to Automate Form Filling With Python
Step 1: Setting Up Your Environment
Before diving in, ensure you have Python installed. Download it from the official Python website. Install your chosen libraries using pip
.
pip install selenium requests beautifulsoup4 pyautogui
Step 2: Analyze the Form
Inspect the web form you want to automate:
- Right-click on the form and select Inspect.
- Identify the input fields (e.g., username, password) and their attributes (
id
,name
, orclass
).
Step 3: Write the Python Script
Here’s an example script using Selenium to log in to a website:
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
# Initialize WebDriver
driver = webdriver.Chrome(executable_path='path_to_chromedriver')
# Open the website
driver.get('https://example.com/login')
# Fill out the form
username = driver.find_element(By.ID, 'username')
password = driver.find_element(By.ID, 'password')
username.send_keys('your_username')
password.send_keys('your_password')
# Submit the form
login_button = driver.find_element(By.ID, 'login-button')
login_button.click()
# Wait and close the browser
time.sleep(5)
driver.quit()
Step 4: Handle Dynamic Forms
Dynamic forms may require handling JavaScript or waiting for elements to load. Use Selenium’s WebDriverWait feature to tackle this.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Wait for the element to appear
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'dynamic-field'))
)
element.send_keys('Dynamic Input')
Advanced Techniques in Python Form Automation
1. Handling CAPTCHA
CAPTCHAs are designed to block bots. While bypassing CAPTCHAs may violate terms of service, you can use tools like anti-captcha services if you have explicit permission.
2. Automating Multi-Step Forms
For forms spanning multiple pages, ensure you automate navigation between pages using Selenium.
3. Using Python Scripts for Web Forms in Bulk
If you’re dealing with bulk data, store it in a CSV file and let Python iterate through each entry. Example:
import csv
with open('data.csv', mode='r') as file:
reader = csv.DictReader(file)
for row in reader:
# Use Selenium to fill the form for each row
pass
Real-World Applications of Python Form Automation
Automating online forms with Python has practical applications across various industries and tasks. By eliminating repetitive manual effort, Python form automation enhances productivity, reduces errors, and simplifies workflows. Let’s dive deeper into these real-world applications and how they can revolutionize your daily tasks or business operations.
1. Job Applications: Fill Out Multiple Job Applications with Consistent Data
Searching for a job often means filling out the same personal information across dozens—or even hundreds—of applications. This repetitive process can be time-consuming and error-prone. Python form automation solves this problem by enabling you to automatically:
- Input your name, email, phone number, and address across multiple forms.
- Attach your resume or cover letter to each application.
- Answer commonly asked questions, such as availability or desired salary.
How It Works:
- Use Selenium to navigate job portals, locate form fields, and input predefined data.
- Store your application data in a CSV file, and let Python iterate through each entry.
Example Use Case:
A university graduate applying to internships on a platform like LinkedIn or Indeed can create a Python script to automatically submit applications, saving hours of repetitive work.
2. E-commerce: Automate Product Listings or Price Updates
In e-commerce, managing product catalogs can be a daunting task, especially if you’re running a store with hundreds of SKUs. Tasks such as adding new products, updating prices, or modifying descriptions can take up a significant amount of time. Python automation streamlines these operations by:
- Uploading product details like names, prices, images, and descriptions in bulk.
- Automatically updating pricing information during sales or promotions.
- Managing inventory by removing out-of-stock items.
How It Works:
- Use Requests or Selenium to interact with your e-commerce platform’s backend.
- Combine Python with APIs provided by platforms like Shopify or WooCommerce for efficient product management.
Example Use Case:
A small business owner using Shopify can automate daily price adjustments or bulk product uploads, freeing up time to focus on marketing and customer engagement.
At Prateeksha Web Design, we specialize in Python automation for online tasks like managing Shopify stores. Our clients have seen faster workflows and reduced manual effort with our tailored solutions. Find out more at Prateeksha Web Design.
3. Data Entry: Submit Surveys or Forms in Bulk
Survey collection and submission can involve repetitive tasks, such as entering standardized responses into forms or submitting data for research purposes. Python scripts can automate these actions, enabling you to:
- Populate survey fields with predefined answers.
- Submit large batches of forms without manual intervention.
- Extract results from surveys for analysis.
How It Works:
- Use Selenium to locate and fill form fields for surveys hosted on platforms like Google Forms or Typeform.
- Store survey responses in a CSV or database and programmatically input them into forms.
Example Use Case:
A research assistant conducting a large-scale survey can use Python to submit multiple responses, ensuring consistent data entry and saving hours of manual work.
4. Small Business: Streamline Client Onboarding Processes
For small businesses, onboarding new clients often involves collecting repetitive information such as contact details, preferences, and business requirements. Automating this process can drastically improve efficiency. Python automation can:
- Automatically fill client details into CRM systems or spreadsheets.
- Generate and email personalized onboarding forms.
- Integrate with third-party tools to sync data seamlessly.
How It Works:
- Combine Python with tools like Zapier or APIs of CRM platforms (e.g., HubSpot or Salesforce).
- Use Requests to submit client information to online forms or databases.
Example Use Case:
A small marketing agency onboarding multiple clients can use Python scripts to populate intake forms and sync client data across tools, eliminating manual input.
5. Other Notable Applications
- Education: Automate submission of student grades or registration forms.
- Healthcare: Populate patient intake forms with repetitive data to save time during appointments.
- Legal Services: Pre-fill contract templates or legal forms for clients.
- Government Services: Automatically submit tax forms, business permits, or grant applications.
Prateeksha Web Design’s Role in Form Automation
At Prateeksha Web Design, we understand the challenges businesses face in managing repetitive tasks. Our team has extensive experience in developing Python scripts for web forms, helping businesses integrate automation into their workflows. Here’s how we help:
- Customized Solutions: We create tailored automation tools for specific industries, ensuring seamless integration into existing systems.
- Browser Automation: With our expertise in Python scripts for online data submission, we handle complex, dynamic forms requiring browser interactions.
- Error Reduction: By automating data entry, we minimize the risk of manual errors, ensuring higher accuracy and consistency.
- Scalable Processes: Our solutions are designed to grow with your business, handling increasing workloads effortlessly.
Whether you’re a freelancer looking to automate job applications or a business managing bulk data entry, Prateeksha Web Design can craft automation solutions that save time, reduce effort, and enhance productivity.
Best Practices for Online Form Automation
- Test Thoroughly: Run your script multiple times to ensure reliability.
- Respect Privacy and Ethics: Always obtain permission before automating interactions with websites.
- Optimize Performance: Use efficient code and libraries like Selenium Grid for scalability.
Conclusion
Automating web forms with Python is a powerful skill that can simplify repetitive tasks, save time, and boost productivity. With tools like Selenium and Requests, even a 20-year-old newbie can create scripts that handle tasks traditionally reserved for tech experts.
Whether you’re a programming geek, a small business owner, or just someone looking to make life easier, learning how to automate repetitive tasks in Python opens up a world of possibilities. And if you need expert guidance, Prateeksha Web Design is here to help you integrate automation seamlessly into your workflow.
Are you ready to take the plunge into Python-powered productivity? Let’s simplify your world, one script at a time!
Interested in learning more? Contact us today!
FAQs
FAQs
1. What is online form automation?
Online form automation refers to the use of technology and scripts to automatically input data into web-based forms, eliminating the need for manual data entry.
2. Why is Python a good choice for automating online forms?
Python is beginner-friendly with an intuitive syntax, offers powerful libraries like Selenium and Requests for web automation, is cross-platform compatible, and has a strong community support system.
3. What libraries do I need to get started with Python form automation?
Essential libraries include Selenium for browser automation, Requests for sending HTTP requests, Beautiful Soup for parsing HTML, and PyAutoGUI for GUI automation tasks.
4. Can I automate the submission of sensitive information, like passwords?
While it is possible to automate sensitive information submission, it is vital to approach this with caution to avoid security risks and data breaches. Always ensure that your scripts handle such data safely.
5. What are some practical applications of Python form automation?
Python form automation can be used in various scenarios, such as automating job applications, updating e-commerce product information, conducting bulk survey submissions, and streamlining client onboarding processes.
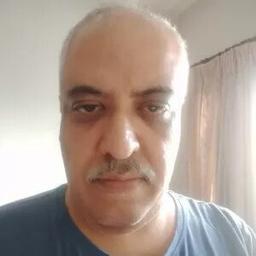