// next-sitemap.js
siteUrl: 'https://www.yourwebsite.com',
generateRobotsTxt: true, // (optional)
}
- Run the command to generate your sitemap:
npm run build
By incorporating a dynamic sitemap into your site, you'll provide search engines with a clear view of your website’s structure, making it easier for them to crawl and index your pages effectively. Plus, having a sitemap can boost your site’s authority and visibility in search engine results.
3. Leveraging Image Optimization
In the realm of Next.js SEO, optimizing images can improve your page load speeds, which is a critical ranking factor. The Next.js next/image
component automatically optimizes images for performance, providing various sizes based on the user’s device.
Implementation Steps:
import Image from 'next/image';
const MyImageComponent = () => (
<Image
src="/path/to/image.jpg"
alt="Description of image"
width={500}
height={300}
quality={75}
/>
);
By using the next/image
component, you ensure that your images are served in the most efficient format and size, enhancing user experience and helping reduce bounce rates.
4. Structuring Your Content with Semantic HTML
Search engines love content that is well-structured and easy to read. Using semantic HTML tags (like <article>
, <section>
, <header>
, <footer>
, etc.) helps convey the meaning of your content more clearly.
Example:
const MyArticle = () => (
<article>
<header>
<h1>The Benefits of Next.js for SEO</h1>
<p>By Digital Dynamo | Date: 2023-10-25</p>
</header>
<section>
<h2>Enhanced Performance</h2>
<p>Next.js excels in performance, which is crucial for SEO...</p>
</section>
<footer>
<p>Related Articles: <a href="/seo-tips">5 SEO Tips</a></p>
</footer>
</article>
);
This not only helps search engines understand your content better but also enhances accessibility for users who rely on screen readers.
5. Implementing Structured Data
Structured data is a game-changer for enhancing SEO. It provides search engines with explicit clues about the meaning of your content, which can lead to rich snippets in search results—like the star ratings or FAQ sections that grab attention.
Setting Up:
You can create structured data in JSON-LD format within the <Head>
component of your Next.js page:
const MyPage = () => (
<>
<Head>
<script
type="application/ld+json"
dangerouslySetInnerHTML={{
__html: JSON.stringify({
"@context": "https://schema.org",
"@type": "WebSite",
"name": "My Amazing Next.js Site",
"url": "https://www.yourwebsite.com",
}),
}}
/>
</Head>
<h1>Welcome to My Amazing Site!</h1>
</>
);
This added information can help your page stand out in search engine results, thereby increasing click-through rates.
Conclusion: The Continuous Journey of SEO Mastery
As we wrap up our exploration of SEO optimization techniques for Next.js, it's important to remember that SEO is an evolving discipline. What works today may change tomorrow, so staying updated with best practices and continuously testing and iterating your strategies is essential.
Harness the power of Next.js, implement these techniques, and watch your website thrive in search engine rankings. Remember, the digital landscape is your oyster—go forth, optimize, and keep swimming towards success!
Additional Tools and Resources
- Google Search Console: Monitor your site's performance in Google Search and troubleshoot issues.
- Lighthouse: Utilize Chrome's built-in performance auditing tool for actionable insights to improve your site.
- Ahrefs or SEMrush: Use these for competitive analysis and keyword research to optimize your content strategy further as you grow.
Happy optimizing! 🌟
Certainly! Below is an expanded, detailed, and refined version of your content, providing a more comprehensive understanding of the topics presented:
siteUrl: 'https://yourwebsite.com',
generateRobotsTxt: true,
}
When search engine bots, which you can envision as diligent mail carriers delivering your site's content to the digital world, arrive at your website, they rely on a sitemap to understand the structure of your content. This sitemap serves as a roadmap, allowing bots to navigate through your pages effortlessly and quickly identify key information. This systematic organization can significantly enhance your site's visibility, potentially leading to improved search engine rankings.
Pro Tip: Just as an e-commerce website in Mumbai needs to keep its inventory fresh and aligned with the latest trends to attract customers, it's crucial to maintain your sitemap consistently updated to reflect changes. Regular updates ensure search engine bots have access to the most relevant and timely content, optimizing your indexing and potentially boosting traffic.
3. Understanding Next.js Structured Data
Now let’s delve deeper into structured data—often referred to as the "secret sauce" for enhancing your website's SEO. Structured data is a standardized format that helps search engines understand the context of your content better. By implementing structured data, you can provide specific details about your pages, such as the type of content they contain, which helps search engines serve relevant information to users.
In the context of a Next.js application, integrating structured data is straightforward with the use of JSON-LD (JavaScript Object Notation for Linked Data). JSON-LD allows you to embed structured data directly within your HTML, without affecting the visible content of your site.
Here’s an example:
import Head from 'next/head';
const MyPage = () => (
<>
<Head>
<script type="application/ld+json">
{`
{
"@context": "https://schema.org",
"@type": "Website",
"name": "My Amazing Site",
"url": "https://yourwebsite.com",
"potentialAction": {
"@type": "SearchAction",
"target": "https://yourwebsite.com/?s={search_term_string}",
"query-input": "required name=search_term_string"
}
}
`}
</script>
</Head>
<h1>Welcome to My Amazing Site!</h1>
</>
);
export default MyPage;
In the above example, we are defining our website as a structured entity with specific attributes such as its name and URL. Moreover, we include a potential action, allowing search engines to understand that users can search content directly from this site.
Integrating structured data can dramatically enhance how your page appears in search results. You may gain attractive metadata displays known as rich snippets, which can boost click-through rates by making your listing more engaging. When your site stands out visually, it’s akin to being the belle of the ball amidst a crowd of competitors, significantly improving user interaction and engagement.
4. Fine-Tuning for Next.js Lighthouse Optimization
Think of Lighthouse as your website's comprehensive fitness tracker—it evaluates the performance, accessibility, and SEO of your site, spotlighting areas for enhancement. This powerful tool, developed by Google, operates as a built-in feature in Chrome DevTools, providing a thorough analysis of your Next.js application.
Key aspects Lighthouse analyzes include:
-
Performance: Measurements like loading speed, interactivity, and the visual stability of your site are crucial. A fast-loading site not only improves user experience but positively influences rankings.
-
Accessibility: Lighthouse checks whether your site can be easily navigated by all users, including those relying on assistive technologies. It emphasizes the importance of usability in reaching a wider audience.
-
SEO: This audit ensures fundamental SEO practices are in place, such as proper use of headings, image alt texts, and metadata. A solid SEO foundation is vital for maximizing visibility on search engines.
After running a Lighthouse report, you’ll receive actionable feedback, including scores for each category and specific recommendations for improvement. By continuously optimizing based on Lighthouse insights, you can ensure that your Next.js site remains not only user-friendly but also competitive in the ever-changing digital landscape.
By exploring these key aspects of site optimization in Next.js, you equip yourself with the knowledge to enhance your web presence, leading to increased traffic and better engagement from your audience. Embrace these practices to turbocharge your site's performance and ensure it stands out in the crowded online marketplace.
Comprehensive Tips for Website Optimization
In today's fast-paced digital environment, the speed and efficiency of your website can greatly affect user experience and search engine rankings. Here are some detailed strategies for optimizing your website effectively:
-
Compress Images to Reduce Load Time:
- High-resolution images can significantly slow down page loading times. Use tools like TinyPNG or ImageOptim to compress images without noticeably sacrificing quality. Aim to use formats like WebP or SVG for better performance.
- Use responsive images with the
<picture>
element orsrcset
attribute in<img>
tags to serve different image sizes based on the user’s device.
-
Optimize JavaScript Bundles:
- Reduce the size of your JavaScript bundles by eliminating unnecessary libraries. Use tree-shaking to remove dead code and ensure that only the necessary functions are included.
- Consider using code-splitting to load only the JavaScript required for the initial load of the page. This can significantly enhance rendering speed and improve performance metrics like First Contentful Paint (FCP).
-
Leverage a Content Delivery Network (CDN):
- A CDN distributes your content geographically across multiple servers, ensuring faster load times for users, regardless of their location. By caching static assets like images, scripts, and stylesheets, CDNs reduce the distance between your users and the server that delivers the content, minimizing latency.
- Popular CDN services like Cloudflare, AWS CloudFront, and Akamai can enhance your site’s performance and provide additional security features, such as DDoS protection.
Just as a well-balanced diet keeps your body fit, regular maintenance and attention to your website keep visitors happy and engaged. A sluggish website can drive users away, harming both engagement and conversions.
Section 5: Focus on Next.js SEO Best Practices
Putting these optimization theories into practice is essential. Follow this handy checklist to help enhance your visibility and user experience in the vast digital landscape:
-
Descriptive URLs:
- Ensure your URLs are clean, descriptive, and structured logically. They should accurately reflect the content on the page (e.g.,
www.example.com/seo-best-practices
).
- Ensure your URLs are clean, descriptive, and structured logically. They should accurately reflect the content on the page (e.g.,
-
Performance Matters:
- Speed is paramount. Utilize Next.js features like Static Site Generation (SSG) and Server-Side Rendering (SSR) to render pages faster. Remember to measure and optimize performance using tools such as Google PageSpeed Insights or GTmetrix.
-
Responsive Design:
- Implement a flexible, responsive design that looks great on all devices. Use media queries in CSS and ensure your layout adjusts seamlessly across various screen sizes.
-
Quality Content:
- Prioritize valuable content over keyword stuffing. Original, engaging, and relevant content not only satisfies your audience but also improves your search rankings. Regularly update your content to ensure it remains fresh and useful.
-
Link Up:
- Build a robust internal linking strategy. Internally linking relevant pages enhances navigation for users and aids search engines in understanding your site structure. Increased dwell time on your site often correlates with improved SEO.
Section 6: The Importance of Next.js Indexing
For your pages to be discovered by users, they must be properly indexed by search engines. Efficient Next.js indexing is crucial for visibility. After launching your content:
- Use Google Search Console: This tool can monitor how your website is being indexed and alert you to any potential issues affecting visibility.
Implementation Tip:
- Submit your sitemap to Google Search Console to expedite the indexing process. Just like giving your friends a heads-up about an upcoming event brings them in faster, a sitemap directs search engines straight to your important content!
Conclusion: Time to Shine!
Congratulations on reaching the end of this SEO guide! The world of Next.js SEO is vast and multi-faceted, but you are now equipped with actionable strategies. Remember, you don't have to navigate this journey alone; expert partners like Prateeksha Web Design can assist you, especially if you're searching for the best web design company in Mumbai.
Take your newfound knowledge and apply it. Experiment with these techniques, keep a close watch on your website's performance metrics, and don’t hesitate to refine your approach as necessary. Whether you are focusing on ecommerce website design in Mumbai or any other type of website, these strategies can elevate your site's performance.
Call to Action: Let's Get Started!
Are you ready to implement these invaluable tips on your Next.js website? Roll up your sleeves, dive into the intricacies of optimization, and start enhancing your site's performance today! If you feel overwhelmed at any point, don't hesitate to engage experienced web developers in Mumbai who can provide you with the support you need.
Your path to growth and success in digital visibility awaits! Are you excited? Because I am! 🌟
In Summary:
- Utilize meta tags effectively for clarity and to enhance SEO benefits.
- Generate and submit sitemaps to guide search engines.
- Implement structured data (schema markup) to improve search visibility.
- Optimize your website with performance tools like Lighthouse.
- Adhere to Next.js SEO best practices to maximize potential.
- Regularly monitor your site’s indexing to ensure all pages are easily discoverable.
Tip: Utilize Next.js's built-in support for server-side rendering (SSR) and static site generation (SSG) to create pages that are crawlable and indexable by search engines, improving your website's visibility and performance in search results.
Fact: Next.js automatically optimizes your JavaScript bundle size and reduces load time by only sending necessary code to the browser, which can lead to better user experience metrics (like reduced bounce rates), contributing positively to SEO rankings.
Warning: Ensure that your Next.js application correctly implements meta tags, structured data, and sitemap generation. Neglecting these can result in poor search engine indexing and visibility, making your site less competitive in search results.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services tailored to enhance SEO for Next.js websites, as outlined in Program Geeks' Ultimate Guide. Their team implements advanced optimization techniques, ensuring fast loading times and improved site architecture. They conduct comprehensive SEO audits, focusing on metadata and structured data integration. Continuous performance monitoring and strategic content recommendations further boost search visibility. Partnering with Prateeksha guarantees a customized approach to elevate your Next.js site's search engine ranking.
Interested in learning more? Contact us today.
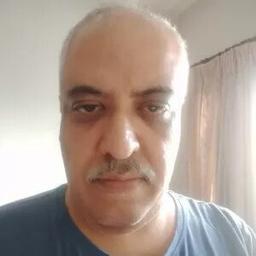