npx create-next-app@latest my-ecommerce-app
This command sets up a brand-new Next.js project with the latest features included, giving you a strong foundation to build your recommendation engine on.
Step 2: Choose Your Recommendation Algorithm
This is where the magic truly begins! You’ll want to choose an algorithm that fits your e-commerce goals. Here are a couple of approaches to consider:
-
Collaborative Filtering: This technique analyzes user behaviors and similarities to recommend products based on what others with similar patterns liked. It’s like being in a book club and discovering new reads based on your friends’ tastes.
-
Content-Based Filtering: Here, the focus is on the attributes of the items themselves. If a user likes running shoes, the engine might suggest other shoes with similar features (like cushioning or support).
-
Hybrid Methods: Why not have a blend? Combining both approaches can provide a robust recommendation system that covers all bases.
Step 3: Gathering Data
Next, you need data! This might involve collecting user behavior data, product descriptions, reviews, and sales history. Having a thorough dataset is like gathering enchanting ingredients for your magical potion; the better the ingredients, the better the results. You can use tools like Google Analytics or your own database to collect this data.
Step 4: Integrating Machine Learning
Once you have gathered enough data, it's time to employ some machine learning magic. You can use libraries like TensorFlow.js or directly integrate APIs such as Amazon Personalize to enhance your recommendation engine. Here’s a simple snippet on how you might fetch recommendations using an API:
const getRecommendations = async (userId) => {
const res = await fetch(`/api/recommendations?userId=${userId}`);
const recommendations = await res.json();
return recommendations;
};
Step 5: Crafting the Front-End
Now, let's create an engaging and visually appealing User Interface (UI) to showcase the recommendations. You’ll want to display these suggestions prominently, perhaps as a carousel or grid. Consider using components from libraries like Material-UI or TailwindCSS to achieve a polished look.
const Recommendations = ({ items }) => {
return (
<div className="recommendations-grid">
{items.map(item => (
<div className="recommendation-item" key={item.id}>
<img src={item.image} alt={item.name} />
<h3>{item.name}</h3>
<p>${item.price}</p>
</div>
))}
</div>
);
};
Step 6: Testing & Iterating
The key to any successful product recommendation engine lies in constant testing and iteration. Monitor how the recommendations perform, gather user feedback, and continue refining your algorithms accordingly. Utilize A/B testing to try out different approaches, providing you insights into what resonates best with your users.
Wrapping It Up
Congratulations! You’re well on your way to unlocking the magic of AI-powered e-commerce with your Next.js recommendation engine. Personalization is not merely a trend; it’s the future of the shopping experience. By investing in this technology, you not only set your brand apart but also create valued and loyal customers—those who will return time and again, eager to discover what you have in store for them.
By marrying the power of AI with a strong framework like Next.js, you’re setting the stage for a delightful and engaging shopping experience. So grab your coding wand, and let the magic unfold! 🌟
Additional Resources
For those ready to dive deeper, consider exploring:
- Next.js Documentation: Get familiar with the capabilities of this amazing framework.
- Machine Learning Basics: Understanding the fundamentals will help you choose the best recommendation strategy for your business.
- E-commerce UX Best Practices: Enhancing user experience will make your site not just functional, but enjoyable!
Happy Coding! 🎉
This comprehensive guide will empower you to build a personalized and AI-driven shopping experience that delights your customers and elevates your e-commerce game!
Expanding, explaining, and refining your content can provide clearer guidance and offer more comprehensive instructions for users. Here's the revised version:
---
## Getting Started with Your E-Commerce Site Using Next.js
We're on a noteworthy journey to build an e-commerce platform powered by Next.js! Below are the detailed steps to create your app, understand customer behaviors through data collection, and implement a recommendation system that enhances user experience.
### Step 1: Initialize Your Next.js Application
To kick off your journey, you need to set up your Next.js environment. This is a straightforward process that lays the groundwork for your e-commerce application.
Open your terminal and input the following command:
```bash
npx create-next-app your-ecommerce-site
This command utilizes npx
to run create-next-app, a tool that initializes a new Next.js application in a folder named "your-ecommerce-site." It automatically installs all necessary dependencies and creates a structure with essential files and folders.
Next, navigate into your project directory:
cd your-ecommerce-site
Now, install any additional packages required:
npm install
This command installs all your app dependencies based on the configuration files created earlier. With these commands executed, your application is ready, similar to the way ordering a pizza sets you up for a delicious meal—without any need to share!
Step 2: Data Collection and Understanding Customer Behavior
Before diving into building your recommendation engine, it’s crucial to gather and analyze user data. Effective data collection should include:
- User Activity: Track user interactions with your site.
- Product Views: Record which items customers are browsing.
- Purchases: Log completed transactions for generating insights.
- Abandoned Carts: Keep track of products that users added to their carts but didn’t purchase.
These data points are vital to understanding customer preferences and behavior.
Analyzing User Data with Machine Learning
To identify patterns and trends within the collected data, utilize machine learning models. Don't worry if you're unfamiliar with this concept; think of it as deciphering a mystery novel. The goal is to look for clues—this time, trends and correlations among user behaviors.
Example: Collaborative Filtering
One common and effective recommendation technique is collaborative filtering. This method relies on analyzing user interactions to find similarities between different customers.
Consider this scenario:
- Customer A enjoys products X and Y.
- Customer B prefers products Y and Z.
Given such data, it’s reasonable to predict that Customer A might also enjoy product Z, based on their shared interest in product Y. This approach builds a strong recommendation engine by leveraging the purchasing behaviors of numerous users.
Step 3: Creating the Recommendation Component
Now it’s time to implement your findings into a practical feature! Begin by creating a new component in your Next.js application dedicated to product recommendations. Let’s name this component ProductRecommendations.js
. This component will display the recommended products to users based on the insights you've gathered.
Here’s a simplified example of how this component might look:
const ProductRecommendations = ({ products }) => {
return (
<div>
<h2>Recommended For You</h2>
<ul>
{products.map(product => (
<li key={product.id}>{product.name}</li>
))}
</ul>
</div>
);
};
export default ProductRecommendations;
At this point, take a moment to appreciate your work! You’re now on your way to creating a personalized shopping experience that can make users feel valued and understood. 🎉
Step 4: Fetching and Displaying Recommendations
Next, you’ll need to connect your recommendation logic with your backend to fetch product data dynamically. Choose any method that fits your project needs, such as the native Fetch API or libraries like Axios.
Here's a sample of how you may implement fetching data using Axios:
import axios from 'axios';
import React, { useEffect, useState } from 'react';
import ProductRecommendations from './ProductRecommendations';
const RecommendedProductsContainer = () => {
const [recommendedProducts, setRecommendedProducts] = useState([]);
useEffect(() => {
const fetchRecommendedProducts = async () => {
try {
const response = await axios.get('/api/recommendations'); // Your API endpoint
setRecommendedProducts(response.data.products);
} catch (error) {
console.error("Error fetching recommended products:", error);
}
};
fetchRecommendedProducts();
}, []);
return <ProductRecommendations products={recommendedProducts} />;
};
export default RecommendedProductsContainer;
In this example, the RecommendedProductsContainer
component fetches product recommendations when it loads and passes them to the ProductRecommendations
component for rendering. Ensure you replace /api/recommendations
with the actual endpoint you will use to get the data.
Conclusion
You've taken significant steps to set up your Next.js e-commerce site. From initializing your app to gathering insightful data and building a personalized recommendation feature, these steps bring your project closer to completion. Embrace this journey—your users will appreciate the tailored shopping experience, and you'll have built something truly remarkable!
Let's expand upon, explain in detail, and refine the provided JavaScript code and subsequent content related to building an e-commerce recommendation engine. We'll break down each section methodically for clarity and depth.
JavaScript Code Explanation and Refinement
import axios from 'axios';
/**
* Fetches personalized recommendations for a user based on their unique user ID.
*
* @param {string} userId - The unique identifier for the user seeking recommendations.
* @returns {Promise<object>} - A Promise that resolves to an object containing recommendation data.
*/
const fetchRecommendations = async (userId) => {
try {
// Make a GET request to the recommendations API endpoint using the userId
const response = await axios.get(`/api/recommendations/${userId}`);
// Return the data received in response, which contains personalized recommendations
return response.data;
} catch (error) {
// Log error message for debugging and throw an error for further handling
console.error(`Error fetching recommendations for user ID ${userId}:`, error);
throw new Error('Could not fetch recommendations, please try again later.');
}
};
Explanation:
-
Importing Axios: The code starts with importing the
axios
library, which is widely used for making HTTP requests. It simplifies the process and handles bothPromise
and callback functionality effectively. -
Function Definition: The
fetchRecommendations
function is an asynchronous function designed to retrieve personalized recommendations for a user. -
Parameter and Return Type:
- The input parameter
userId
is crucial since it uniquely identifies the user whose recommendations are being requested. - The function is expected to return a Promise that resolves to an object containing the user's recommendations.
- The input parameter
-
Error Handling: We've added a
try...catch
block to handle potential errors gracefully:- If the API call fails (due to network issues, an invalid user ID, etc.), it logs the error to the console for debugging.
- It throws a new error message to inform the caller that something went wrong, making it easier to manage failures.
Step 5: Testing & Iteration
After the recommendation engine is operational, it’s essential to implement a robust testing strategy:
-
Gathering User Feedback: Engaging real users to provide feedback is akin to having trusted friends sample your culinary creation. They can offer valuable insights on what could be improved.
-
A/B Testing: This method allows you to compare two variations (A and B) of your recommendation engine. For example:
- Version A could display recommendations based on user behavior.
- Version B might utilize demographic data.
Analyzing which version performs better can guide further optimizations and refinements.
Step 6: Deploying Your Creation
Once you’ve thoroughly tested the recommendation engine and feel it’s ready to launch, deployment becomes the next exciting step:
-
Choosing a Deployment Platform: For those using Next.js, a popular framework for React applications, platforms like Vercel provide a seamless experience. They handle the complexities of scaling and deploying your application, allowing you to focus on development.
-
Monitoring Post-Deployment: After deployment, monitor user interactions with the recommendation engine closely. Tools like analytics can help capture user behavior and engagement metrics.
The Bang for Your Buck: Design and User Experience
While the recommendation engine enhances functionality, the visual aesthetics and user experience (UX) of your e-commerce platform are just as critical:
-
Engagement through Design: An eye-catching design keeps users on your site longer. Think of it as curating an art gallery—each product must invite exploration.
-
Strengthening Brand Identity: The design of your e-commerce site should resonate with your brand's ethos and message. Every visual element must feel intentional and aligned.
-
Building Trust and Credibility: A professional design fosters trust. If users perceive your site as credible and user-friendly, they’re more likely to return.
Partnering with Experts
If you’re searching for expertise in crafting a stunning storefront, look no further than Prateeksha Web Design, hailed as one of the best web design agencies in Mumbai. Their proficiency spans across various services, ensuring your e-commerce experience is visually compelling and functionally robust.
Wrapping It All Up: Take the Next Step
You've traversed the e-commerce landscape, equipped with a framework for developing a Next.js recommendation engine.
-
Emphasize Data-Driven AI Recommendations: Remember that the strength of AI-powered recommendations is rooted in data. Continuously adapt your offerings based on user interactions and preferences.
-
Engage with Stakeholders: This journey doesn’t have to be solitary. Collaborate with a trustworthy ecommerce website development company in Mumbai, like Prateeksha Web Design, to realize your vision fully.
Now, as you embark on this digital entrepreneurial endeavor, channel your creativity into building a platform that not only meets user needs but exceeds expectations.
Embrace the adventure ahead! Each successful e-commerce platform started as a vision—yours is about to ignite. Happy coding! 💥
Tip:
Leverage Personalization Algorithms: Utilize machine learning models like collaborative filtering or content-based filtering to offer personalized recommendations. Incorporate user behavior data, such as clicks and purchase history, to enhance the relevance of your recommendations, leading to improved user engagement and conversion rates.
Fact:
Real-Time Data Processing: Next.js supports server-side rendering, which is beneficial for delivering real-time, personalized content. By integrating APIs that handle user interactions and behavior analytics, you can ensure that your e-commerce site presents timely product recommendations, creating a dynamic shopping experience that adapts to users' preferences.
Warning:
Data Privacy Compliance is Crucial: When implementing AI-driven recommendations, ensure you adhere to data privacy regulations like GDPR and CCPA. Collecting and processing user data without consent can lead to severe legal consequences and damage your brand's reputation. Always inform users about data usage and provide clear opt-in/opt-out options.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services for building AI-powered e-commerce solutions using Next.js. Their expertise includes customized web design, seamless integration of AI algorithms for personalized recommendations, and responsive user interfaces. They provide end-to-end development support, ensuring optimal performance and user engagement. Additionally, Prateeksha focuses on enhancing customer experience through data-driven insights and innovative design strategies. With a team of skilled developers, they ensure your e-commerce platform stands out in the competitive digital landscape.
Interested in learning more? Contact us today.
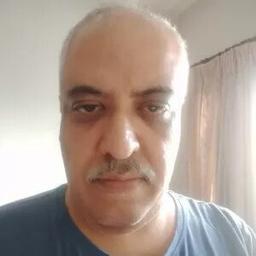