protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'log' => \App\Http\Middleware\LogRequests::class,
'cors' => \App\Http\Middleware\Cors::class,
];
With this setup, you can easily apply middleware to your routes, ensuring that only authenticated and authorized requests make it to your application logic.
Pro Tip:
Develop custom middleware tailored to your application's needs. For example, you might want to create middleware for user role checks, ensuring only certain roles can access specific endpoints.
3. Implement Rate Limiting
In the exhilarating world of APIs, you can't have everyone diving in headfirst at once—you need to control the flow! Enter rate limiting. This practice helps you safeguard your resources by limiting the number of requests a user can make to your API in a given timeframe.
Why is this important?
-
Prevent Denial of Service (DoS) attacks: By restricting the number of requests, you protect your API from overloading.
-
Fair Usage: It ensures all users get a fair share of the service without unfair burdening by a few requests-happy users.
How to implement?
You can easily set up rate limiting in Laravel's api.php
routes file using:
Route::middleware('throttle:60,1')->group(function () {
Route::get('/user', function () {
// fetch and return user data
});
});
This setup would allow 60 requests per minute—they'll have to wait if they try to exceed that!
Pro Tip:
Monitor your rate limiting metrics to adjust thresholds and improve user experience based on real usage trends.
4. Fortify API Token Security
Your API tokens are your security badges; losing them can turn your concert into complete chaos. It’s essential to manage these tokens wisely to keep threats at bay.
Best practices include:
-
Use HTTPS: Always ensure your API communicates over HTTPS to keep tokens and data encrypted during transit. Anyone sniffing the network will be left empty-handed.
-
Short-lived Tokens: Create tokens that expire after a set period—not only does this improve security, but it also minimizes the risk of token theft.
-
Revocation: Plan for instances where a token needs to be invalidated, like when a user logs out or changes their password.
Example:
Think of it as having a temporary wristband for an event—once you're done, it gets cut off!
Pro Tip:
Regularly review and roll your authentication keys and secrets in line with security best practices.
5. Protect Your Data
Just like you wouldn’t want unruly guests spilling drinks on your event area, you need to protect your API from data breaches. Proper data handling and storage are crucial.
Strategies include:
-
Sanitize Input: Always validate and sanitize user input to prevent SQL injection attacks and XSS vulnerabilities.
-
Use Eloquent's Built-in Protection: Make use of Eloquent ORM's built-in protections against mass assignment vulnerabilities, ensuring only the intended attributes are assigned.
-
Data Encryption: Encrypt sensitive information before storage. Laravel provides built-in features to easily encrypt and decrypt data.
Example:
Similar to keeping valuables locked away during the party—you want to ensure only authorized individuals have access!
Pro Tip:
Consider falling back on hashing mechanisms for passwords, utilizing Laravel's built-in Hash facade.
6. Enhance Endpoint Security
Your endpoints showcase your talent, but they also invite scrutiny. It's essential that they’re fortified against unwanted guests.
Recommendations include:
-
Limit Data Exposure: Only expose what’s necessary. For instance, return a minimal subset of user information; don’t offer the entire dataset.
-
Validate Payloads: Thoroughly check incoming data formats. Laravel's validation rules can prevent numerous common pitfalls—make use of them!
-
Use versioning: By versioning your API (e.g.,
/v1/user
), you maintain backward compatibility and allow for iterative improvements without breaking existing applications.
Example:
Think of it like having a VIP section at a club—invitation-only for specified guests!
Pro Tip:
Document your API endpoints using Swagger or Postman to ensure that all potential endpoints are well-defined and can be easily tested.
7. Embrace Continuous Learning & Improvement
In this fast-paced digital universe, you can't rest on your laurels. The landscape of cybersecurity is continuously evolving, and so should your knowledge and practices.
Tips for continuous improvement:
-
Stay Updated: Regularly track Laravel’s updates and security bulletins. Follow key Laravel contributors and cyber security experts on social media.
-
Participate in the Community: Engage in community forums like Laravel’s official forum, Stack Overflow, or Reddit to exchange knowledge and strategies.
-
Perform Regular Audits: Conduct regular security audits and penetration tests on your APIs to identify and rectify vulnerabilities.
Example:
Think of this as constantly fine-tuning your DJ mix—always improving to ensure a fantastic show for your audience!
Pro Tip:
Consider attending workshops and conferences on API security and Laravel development to keep your skills sharp.
Conclusion
Securing your Laravel APIs is not just a one-time setup; it’s an ongoing commitment to ensuring your digital space remains safe from cyber threats. Enjoy the thrills of API development, but never forget to buckle up with solid security practices. With the insights from this guide, you’re now armed with tools and strategies to transform your Laravel APIs into a fortress against cyber wolves. Happy coding—stay safe out there! 🎉
This expanded and refined content enhances the structure, provides in-depth explanations, and clarifies various technical aspects. It also includes practical examples and precautionary tips, making it more useful for readers looking to understand and implement Laravel API security.
Certainly! Let's expand and clarify the provided content, emphasizing the importance of each concept while maintaining a friendly tone.
---
### Understanding Middleware in Laravel
In Laravel, the `protected $routeMiddleware` array is a crucial component for defining middleware that can be applied to routes. Middleware acts like a layer of filtering, processing HTTP requests entering your application.
Here’s a closer look at our middleware configuration:
```php
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'log' => \App\Http\Middleware\LogRequests::class,
];
Key Middleware Definitions:
-
Auth Middleware (
\App\Http\Middleware\Authenticate::class
):- This middleware is responsible for verifying whether a user is authenticated before they can access certain routes. If a user attempts to access a protected route without being logged in, they will be redirected to the login page. This layer ensures that only authorized users can interact with sensitive parts of your application.
-
Log Requests Middleware (
\App\Http\Middleware\LogRequests::class
):- This middleware logs incoming requests to your application. By tracking requests, you can gather intelligence on user behavior, spot potential issues, or analyze patterns for improved service delivery. This is like having a security camera that keeps an eye on who’s entering and exiting.
Pro Tip:
Instead of trying to reinvent the wheel by creating your own middleware for common tasks, leverage the robust collection of existing middleware that Laravel offers. It’s akin to enjoying your go-to pizza from your favorite pizzeria instead of investing time in baking one at home! Why not make your life easier and utilize what’s readily available?
Implementing Rate Limiting
What is Rate Limiting?
Imagine you're at your favorite café where the barista manages the flow of customers to ensure everyone gets served efficiently. Laravel rate limiting acts similarly by controlling how many requests a user can make to your application within a specific timeframe—this is crucial for maintaining performance and protecting your server from potential overloads.
By implementing rate limiting, you can easily prevent DDoS (Distributed Denial of Service) attacks, ensuring smooth operation even under significant traffic.
How it Works
You can define a restriction such as allowing a user to make 100 requests per minute. This setting ensures that while users can interact with your API, there is a safeguard against excessive requests that could hamper performance.
Example in Action:
Think of a café that only accepts a limited number of customer orders at once. If they allow too many people to place orders simultaneously, chaos ensues. Rate limiting ensures that your application runs much like that well-organized café.
Implementation in Laravel:
You can easily set up rate limiting using middleware in your route definitions:
Route::middleware('throttle:100,1')->group(function () {
Route::get('/api/resource', 'ApiController@index');
});
This code snippet means that a user can make up to 100 requests to the /api/resource
endpoint every 1 minute.
Pro Tip:
When implementing rate limiting, don't forget to provide clear and friendly error messages. If a user exceeds their limit, instead of a generic error, try sending them something like, “Hey, ease up there, partner! You’ve hit the limit for now. Come back soon!” A little humor goes a long way in enhancing user experience.
Fortifying API Token Security
When we discuss API token security, think of tokens as the golden tickets that grant access to your application's exclusive features. Just like you wouldn’t want to give away your concert ticket, it’s vital to protect these tokens from falling into the wrong hands.
Best Practices for Token Security
-
Short-lived Tokens: Just like a party needs to end eventually, so should your tokens. Use short-lived tokens that expire after a certain period. This minimizes the risk in case they are compromised.
-
Secure Storage: Ensure that your tokens are stored in environments that are secure, such as environment variables or encrypted storage solutions. Keeping tokens hidden and safe is paramount—imagine leaving your prized possessions out in the open!
Real-world Analogy:
Picture an exclusive club that gives wristbands to members at the door. If you lose your wristband (token), you’re not getting back in without verification! This highlights the importance of secure token management.
Protecting Your Data in Laravel
Just like you wouldn’t leave your front door wide open, protecting your application's data is non-negotiable. Laravel data protection measures ensure that your sensitive information remains safe from unauthorized access.
Strategies for Data Protection:
- Encryption: Leverage Laravel’s built-in encryption services to safeguard sensitive data. This means even if a malicious actor gains access to your database, any data they retrieve will be securely encoded, much like trying to interpret hieroglyphics without a Rosetta Stone.
Implementation:
Laravel provides simple methods to encrypt data, which you can utilize throughout your application. The encryption is seamless and helps maintain the confidentiality and integrity of sensitive information.
Conclusion
By integrating these best practices into your Laravel application, you strengthen its security and ensure a good user experience. From utilizing middleware to securing tokens and encrypting data, each step plays a significant role in contributing to a robust application architecture. So, gear up to make your application not just functional but secure and efficient!
Validation: Ensuring Data Integrity
In web application development, validation is a critical practice that ensures the integrity and security of incoming data. Proper validation verifies that the data received conforms to expected formats and standards before it is processed further. This is essential because relying on invalid or unexpected data can lead to a range of issues—from application errors to security vulnerabilities.
A common practice in Laravel (a popular PHP framework) is to implement validation rules to enforce data integrity. The following code snippet illustrates how to validate an email and password during a request:
$request->validate([
'email' => 'required|email',
'password' => 'required|string|min:8',
]);
In this example:
- The
email
field is mandated to be present (required
) and must adhere to valid email format (email
). This ensures that only well-formed email addresses can enter the system. - The
password
field requires not only its presence (required
) but also ensures that it is a string of at least eight characters (string|min:8
). This eliminates the possibility of weak passwords that can be easily cracked.
Pro Tip:
Keep your dependencies updated! Consider it akin to hiring a personal trainer for your application. Regular updates enhance security, introduce new features, and prevent bugs from accumulating over time.
6. Enhance Endpoint Security
When it comes to managing Laravel endpoint security, one cannot underestimate the importance of protecting API endpoints. These endpoints serve as pathways that allow client applications to interact with server-side resources. If improperly managed, they can expose sensitive data and lead to detrimental security breaches.
Limit Exposed Endpoints:
- Exercise caution in exposing endpoints—only make those necessary for your application's functionality accessible to the public.
- Implement scope-based permissions to limit access according to user roles. This is akin to creating ‘VIP zones’ in an event, where only selected individuals have access to exclusive areas, thereby enhancing security.
Example:
If your API were likened to a concert, think of it this way: ensure that only the appropriate audience gains access to backstage passes. This kind of restriction not only safeguards sensitive information but also maintains a controlled environment for operations.
7. Embrace Continuous Learning & Improvement
In the ever-evolving sphere of Laravel API security, continuous education is paramount. The security landscape constantly changes as new threats emerge and new defenses are created. Therefore, it is vital to stay updated with current trends and practices, not just for theoretical knowledge but for practical application as well. Don’t hesitate to experiment with your applications to identify and rectify vulnerabilities.
Resources to Explore:
-
Laravel Documentation: This comprehensive guide serves as an essential reference for developers at all levels, offering insights into the framework’s features and capabilities.
-
Online Courses: Platforms such as Udemy or Coursera provide rich repositories of courses focused on Laravel and its security measures, catering to various skill levels.
Pro Tip:
Engage with online communities like Stack Overflow or Reddit. Interacting with fellow Laravel enthusiasts provides invaluable insights and potential solutions to common challenges. Sharing knowledge fosters growth and can lead to innovative ideas and methodologies.
Wrapping It Up
In conclusion, this guide serves as a blueprint for securing Laravel APIs against potential cyber threats. 🛡️ Your overarching strategy should consist of layered Laravel security practices. This approach incorporates robust authentication, effective middleware application, intelligent rate limiting, reliable token management, and stringent data protection measures. Together, they form an impenetrable fortress against cyber intrusions.
Once you have fortified your API, an exciting realm of web design possibilities unfolds before you. If you’re eager to enhance your online presence with an eye-catching ecommerce website design in Mumbai, consider engaging the expertise of Prateeksha Web Design. They are renowned in Mumbai for delivering stunning, functional web design solutions that will enable your API to shine on a grand platform.
Call to Action
Now that you're equipped with these valuable insights, what will your next move be? Take a moment to review your current Laravel API setup. Are there adjustments you could make? Why not start implementing one best practice at a time? Remember, incremental changes can lead to significant enhancements over time. Happy coding, and may your APIs remain secure!
And don't forget, if you’re aiming to elevate your web design, consider reaching out to skilled mumbai website designers or a reputable web design agency in Mumbai. Whether you seek to build a sophisticated site or require expertise in ecommerce website development, the options available are vast!
Stay curious, stay proactive, and keep building! ✨
Tip:
Implement API Authentication: Always use robust authentication mechanisms like Laravel Sanctum or Passport for securing your APIs. This adds a layer of protection, ensuring that only authorized users can access your application's endpoints.
Fact:
Rate Limiting: Laravel provides built-in rate limiting features through middleware. By setting appropriate limits on the number of requests a user can make in a given timeframe, you can effectively mitigate the risks of brute-force attacks and denial-of-service threats.
Warning:
Avoid Exposing Sensitive Data: Be cautious when returning data in API responses. Never expose sensitive information such as user passwords, API keys, or any personal data. Always filter and validate the data to minimize the risk of leaking critical information.
About Prateeksha Web Design
Prateeksha Web Design specializes in implementing robust security measures for Laravel APIs, guided by Program Geeks' best practices. We focus on authentication protocols, encrypted data transfers, and regular security audits to safeguard against cyber threats. Our team ensures proper validation and sanitization of user inputs to prevent vulnerabilities like SQL injection and XSS attacks. We also provide ongoing support and training for developers to maintain security awareness. Trust us to enhance your API security and protect your digital assets effectively.
Interested in learning more? Contact us today.
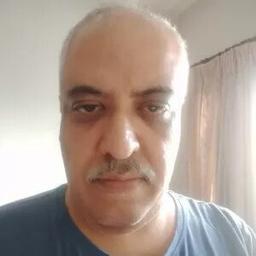