Unlocking the Power of Next.js API Routes: Building Custom Backends with Program Geek
Building a custom backend can seem intimidating, especially when working with complex applications. However, Next.js API Routes simplify this process, making it accessible even for developers who are just starting out. This comprehensive guide will delve deep into how to use Next.js API Routes to build robust custom backends, optimized for Program Geek backend solutions. Along the way, we'll showcase practical examples, best practices, and cutting-edge insights while demonstrating how Prateeksha Web Design can help small businesses leverage this technology effectively.
What Are API Routes in Next.js?
API Routes in Next.js are a feature that lets you create backend logic directly within your Next.js application. They allow developers to set up endpoints for server-side operations without needing to configure or manage a separate backend server. These routes are implemented as serverless functions, which means they are deployed and executed only when needed, providing scalability and efficiency.
This capability makes Next.js not just a frontend framework but also a backend tool, enabling full-stack development with ease. For small businesses and startups, this is especially beneficial because it reduces the technical overhead and costs of maintaining a standalone backend infrastructure.
How API Routes Differ from Traditional Backends
In a traditional backend setup, you might need to:
- Set up a server with Node.js, Django, or another backend framework.
- Configure the server to handle routes and APIs.
- Maintain the server infrastructure, including hosting, scalability, and uptime.
With API Routes in Next.js, all of this is simplified:
- No Dedicated Server: You don’t need a constantly running server. The serverless functions execute on-demand, triggered by user requests.
- Integrated Development: Backend logic resides within the same codebase as your frontend, eliminating context-switching between different projects.
- Platform Scalability: Platforms like Vercel and AWS Lambda handle deployment and scaling automatically.
Key Features of Next.js API Routes
1. Serverless by Default
When you use API Routes, each route is deployed as a serverless function. Serverless computing means:
- Pay-as-you-go: You’re billed only for the execution time of your API endpoints, not for idle server time.
- Automatic Scalability: The function automatically scales to handle varying numbers of requests. Whether it’s one request per day or thousands per second, the infrastructure adjusts dynamically.
This is particularly useful for small businesses that may experience unpredictable traffic patterns but don’t want to overpay for underutilized servers.
2. File-based Routing
Next.js simplifies routing by using its file system as the routing mechanism. Here's how it works:
- Every file in the
/pages/api/
directory is automatically treated as an API route. - The file name corresponds to the endpoint URL. For instance:
- A file named
hello.js
in/pages/api/
corresponds to the endpoint/api/hello
. - Subfolders within
/api/
allow for more structured routes, like/api/user/profile
.
- A file named
This eliminates the need for manually defining and mapping routes, making development faster and less error-prone.
3. Built-in Middleware
Middleware is essential for tasks like:
- Cross-Origin Resource Sharing (CORS): Ensures your API can be accessed from different domains.
- Authentication: Validates user credentials before processing a request.
- Session Management: Maintains user state across requests.
Next.js API Routes make it easy to integrate middleware directly into your routes. You can also use third-party libraries like cors
or jsonwebtoken
for enhanced functionality.
4. Flexible Data Handling
API Routes can:
- Fetch data from external APIs.
- Interact with databases like MongoDB, PostgreSQL, or Firebase.
- Process incoming data from forms or frontend requests.
This flexibility enables developers to build anything from simple contact forms to complex e-commerce backends directly within a Next.js application.
Setting Up Your First API Route
Creating an API Route in Next.js is straightforward and intuitive. Here's a step-by-step explanation of the example provided:
Step 1: Create the File
In your project directory, navigate to /pages/api/
and create a new file, hello.js
. This file will automatically become an API endpoint available at /api/hello
.
Step 2: Define the Handler Function
Inside hello.js
, define the handler
function. This function processes the request (req
) and sends a response (res
).
export default function handler(req, res) {
res.status(200).json({ message: 'Hello, world!' });
}
req
: This object contains details about the HTTP request, such as:- The request method (
GET
,POST
, etc.). - Query parameters (
req.query
). - Request body data (
req.body
). - Headers (
req.headers
).
- The request method (
res
: This object is used to send a response back to the client. You can:- Set the status code using
res.status(code)
. - Send JSON data using
res.json(data)
or plain text withres.send(data)
.
- Set the status code using
Step 3: Access the Endpoint
Run your Next.js application locally and visit the URL:
http://localhost:3000/api/hello
You’ll see this JSON response in your browser or API testing tool:
{ "message": "Hello, world!" }
Why This Example Is Important
This simple "Hello, World!" example might seem basic, but it lays the foundation for much more powerful functionality. Here's why it's significant:
- Proof of Concept: It demonstrates how easy it is to set up a working API endpoint.
- Serverless Execution: The example shows how serverless functions work in practice.
- Scalability: This endpoint can handle anything from a single request to thousands without additional configuration.
Next Steps: Expanding Your API Routes
Once you’ve set up your first route, you can start adding complexity:
- Dynamic Routes: Create endpoints that accept parameters, like
/api/user/[id]
. - Middleware Integration: Add security or preprocessing logic.
- Database Connectivity: Fetch or store data using databases.
For instance, you can build a contact form backend where user submissions are saved to a database, or you can set up an API that interacts with third-party services like Stripe or Twilio.
Advanced Use Cases for API Routes
While a basic route is great for static responses, real-world applications demand more complexity. Here's how you can expand your API Routes to handle various backend needs:
1. Dynamic Routing for CRUD Operations
Dynamic API routes empower you to build endpoints that accept parameters directly in the URL.
// pages/api/user/[id].js
export default function handler(req, res) {
const { id } = req.query;
if (req.method === 'GET') {
res.status(200).json({ message: `Fetching user with ID: ${id}` });
} else if (req.method === 'POST') {
res.status(200).json({ message: `Updating user with ID: ${id}` });
} else {
res.status(405).json({ error: 'Method Not Allowed' });
}
}
This route can handle different HTTP methods (GET, POST, etc.), making it a versatile tool for any application.
2. Integrating Databases
Next.js API Routes can interact with databases like MongoDB, PostgreSQL, or even Firebase. Let’s look at a basic example with MongoDB:
import { MongoClient } from 'mongodb';
const uri = process.env.MONGO_URI; // Add your MongoDB URI here
export default async function handler(req, res) {
const client = new MongoClient(uri);
try {
await client.connect();
const database = client.db('my_database');
const collection = database.collection('users');
if (req.method === 'GET') {
const users = await collection.find().toArray();
res.status(200).json(users);
} else if (req.method === 'POST') {
const newUser = req.body;
const result = await collection.insertOne(newUser);
res.status(201).json(result);
}
} catch (error) {
res.status(500).json({ error: 'Failed to connect to database' });
} finally {
await client.close();
}
}
This example shows how to:
- Connect to a MongoDB database.
- Perform CRUD operations using Next.js API Routes.
3. Handling Authentication
Secure your endpoints with authentication middleware. For example, using JSON Web Tokens (JWT):
import jwt from 'jsonwebtoken';
export default function handler(req, res) {
const token = req.headers.authorization?.split(' ')[1];
try {
const decoded = jwt.verify(token, process.env.JWT_SECRET);
res.status(200).json({ message: 'Authorized', user: decoded });
} catch (error) {
res.status(401).json({ error: 'Unauthorized' });
}
}
This approach ensures that sensitive data is only accessible to authorized users.
Benefits of Using Next.js API Routes for Program Geek Backend Solutions (Detailed Explanation)
Building a backend for a web application often involves juggling multiple frameworks, tools, and configurations. However, Next.js API Routes streamline this process, making it more accessible and efficient for developers and businesses. Below, we break down the specific benefits of using Next.js API Routes for Program Geek backend solutions, explaining why they are a game-changer for modern web development.
1. Simplified Development
With Next.js, developers can write both frontend and backend logic in the same project, creating a seamless full-stack development experience.
- Unified Codebase: Traditionally, developers manage separate repositories for frontend and backend, requiring time-consuming context switching. With Next.js API Routes, the backend logic resides within the same Next.js project, reducing complexity.
- File-based Routing: Instead of setting up routes manually (as in Express.js or other backend frameworks), you define a file under
/pages/api/
to automatically create an API endpoint. For example:- File:
/pages/api/data.js
- Endpoint:
/api/data
- File:
This simplicity speeds up development, making it easier for small teams or solo developers to build complete applications.
- Tool Integration: Next.js integrates seamlessly with modern tools like TypeScript, ESLint, and Prettier, ensuring clean and maintainable code for both frontend and backend.
Real-world Example: For Program Geek, if you’re building a directory of AI tools, you can fetch and display tool data within the same project, significantly reducing development time and effort.
2. Cost Efficiency
Managing traditional server infrastructure can be expensive and resource-intensive. Next.js API Routes leverage serverless computing, offering significant cost savings.
- No Dedicated Servers: With serverless functions, there’s no need to maintain a dedicated server that runs 24/7. The function only executes when requested, eliminating costs for idle server time.
- Pay-as-you-go Pricing: Platforms like Vercel (where Next.js was developed) or AWS Lambda charge only for the duration of function execution. This model is especially advantageous for applications with fluctuating traffic.
- Scalability: Serverless functions scale automatically based on demand, so businesses don’t need to invest in expensive infrastructure to handle occasional traffic spikes.
Real-world Example: A small business using Program Geek's backend solutions might only need 100 API calls per day. With serverless functions, they pay only for those requests, making it a budget-friendly choice for startups or growing businesses.
3. SEO Advantages
Search Engine Optimization (SEO) plays a critical role in attracting organic traffic, especially for web applications and e-commerce platforms. Next.js API Routes contribute to better SEO performance in several ways:
- Fast Page Loads: Slow-loading pages can hurt your SEO rankings. By directly fetching required data via API Routes and delivering it to the frontend, you reduce latency and enhance page speed.
- Server-side Rendering (SSR): Next.js enables SSR, meaning pages are pre-rendered on the server before being sent to the user. API Routes can be integrated into SSR processes to fetch dynamic data at runtime, ensuring search engines index fully rendered content.
- Reduced Dependencies: Instead of relying on external APIs (which might introduce latency), you can create custom API endpoints that are optimized for your application's specific needs.
Real-world Example: Imagine a blog section on Program Geek that highlights trending AI tools. By using API Routes, you can fetch the latest data dynamically and serve SEO-optimized pages with minimal delay, helping your site rank higher on search engines.
4. Customizable Middleware
Middleware refers to code that executes between receiving a client’s request and sending a response. Next.js API Routes make middleware integration straightforward, enabling powerful configurations tailored to your application’s needs.
Key Middleware Features:
-
Caching:
- Cache responses to reduce the number of calls to a database or third-party service.
- Improves performance and reduces API request costs.
-
Rate Limiting:
- Protect your API from being overwhelmed by limiting the number of requests a client can make within a specific timeframe.
- Prevents abuse and ensures fair usage.
-
Logging:
- Log every API request for debugging, performance monitoring, or auditing purposes.
- Helps identify bottlenecks and optimize endpoint performance.
-
Authentication and Authorization:
- Implement user authentication (e.g., with JSON Web Tokens or OAuth).
- Control access to specific endpoints based on user roles.
Implementation Example:
Here’s how you might add rate limiting to a Next.js API Route:
let requestCounts = {};
export default function handler(req, res) {
const ip = req.headers['x-forwarded-for'] || req.connection.remoteAddress;
if (!requestCounts[ip]) {
requestCounts[ip] = 1;
} else {
requestCounts[ip]++;
}
if (requestCounts[ip] > 10) {
return res.status(429).json({ error: 'Too many requests' });
}
res.status(200).json({ message: 'Request successful!' });
}
In this example, a client is limited to 10 requests. If they exceed that limit, they receive a 429 Too Many Requests error.
Best Practices for Building with Next.js API Routes
-
Structure Your Routes Intuitively: Keep your API routes modular by separating concerns. For example, group similar routes (e.g.,
/api/users
,/api/products
). -
Error Handling: Always include meaningful error messages for better debugging and user experience.
res.status(400).json({ error: 'Invalid input' });
-
Environment Variables: Use
.env
files to secure sensitive information like API keys and database credentials. -
Testing and Debugging: Use tools like Postman or Insomnia to test your routes and ensure they behave as expected under different conditions.
Showcasing Prateeksha Web Design’s Expertise
At Prateeksha Web Design, we specialize in creating tailored solutions for businesses using technologies like Next.js API Routes. Whether you're building a custom backend or looking for a complete e-commerce solution, our team ensures that your application is optimized, secure, and scalable.
Why Choose Prateeksha Web Design?
- Expertise in building full-stack applications.
- Affordable solutions tailored to small businesses.
- Proven track record of successful backend implementations.
Let us handle your backend challenges so you can focus on growing your business.
Encouraging Small Businesses to Leverage Next.js API Routes
For small businesses, managing a backend often feels like an overwhelming challenge, especially if you're juggling limited technical expertise and budget constraints. However, Next.js API Routes eliminate many of the traditional complexities, offering a streamlined, cost-effective, and scalable solution to backend management. When paired with the expertise of Prateeksha Web Design, small businesses can unlock powerful features tailored to their specific needs, enhancing their online presence and operational efficiency.
Why Next.js API Routes are Perfect for Small Businesses
- Simplicity: Next.js API Routes integrate seamlessly into your existing website setup, allowing you to manage backend functionality without additional server infrastructure or complex configurations.
- Affordability: As a serverless solution, Next.js minimizes hosting costs by charging only for the resources you use. This is particularly beneficial for businesses with fluctuating traffic or seasonal demands.
- Flexibility: API Routes can be customized to suit various business needs, from handling customer orders to integrating third-party services.
Whether you run an e-commerce store, a consulting agency, or a creative studio, Next.js API Routes empower you to build advanced features quickly and efficiently.
Real-Life Use Cases for Small Businesses
To showcase the versatility of Next.js API Routes, here are three practical examples where small businesses can benefit:
1. E-commerce Integration
For e-commerce businesses, managing backend operations such as inventory, order processing, and customer data is critical. With Next.js API Routes, these tasks become much simpler.
- Inventory Management: Create API endpoints to fetch, update, and monitor inventory levels in real-time. This ensures your online store always displays accurate stock availability.
- Order Processing: Use API Routes to handle customer orders. For example, when a user places an order, an endpoint can process the request, update inventory, and send an email confirmation.
- Secure Payments: Integrate payment gateways like Stripe or PayPal directly through API Routes to manage transactions securely.
Example:
// pages/api/order.js
import {
processPayment,
updateInventory,
sendConfirmationEmail,
} from '../../utils';
export default async function handler(req, res) {
if (req.method === 'POST') {
const { orderDetails } = req.body;
try {
const paymentResult = await processPayment(orderDetails.paymentInfo);
if (paymentResult.success) {
await updateInventory(orderDetails.items);
await sendConfirmationEmail(orderDetails.customerEmail);
res.status(200).json({ message: 'Order processed successfully!' });
} else {
res.status(400).json({ error: 'Payment failed.' });
}
} catch (error) {
res.status(500).json({ error: 'Order processing failed.' });
}
} else {
res.status(405).json({ error: 'Method not allowed' });
}
}
This example demonstrates how a single API Route can handle the complete order lifecycle, reducing the need for external services.
2. Custom Dashboards
Small businesses often need custom dashboards for their customers or internal teams. With Next.js API Routes, you can build dashboards that fetch and display user-specific data securely.
- Personalized User Experience: Create API endpoints that deliver tailored content, such as order histories, subscription details, or loyalty points.
- Admin Tools: Build admin dashboards that allow you to manage content, view analytics, or handle customer support requests.
Example:
// pages/api/dashboard.js
import { getUserData } from '../../utils';
export default async function handler(req, res) {
const { userId } = req.query;
try {
const userData = await getUserData(userId);
res.status(200).json(userData);
} catch (error) {
res.status(500).json({ error: 'Failed to fetch user data.' });
}
}
This API Route fetches user-specific data securely, ensuring sensitive information is handled responsibly.
3. Third-Party Integrations
Many small businesses rely on third-party tools to enhance their services. Next.js API Routes make it easy to integrate these services into your website.
- Stripe for Payments: Manage recurring subscriptions, invoices, and one-time payments.
- Twilio for SMS Notifications: Send order confirmations, delivery updates, or promotional messages via SMS.
- HubSpot for CRM: Sync customer data with HubSpot to streamline marketing and sales workflows.
Example: Integrating Stripe
// pages/api/payment.js
import Stripe from 'stripe';
const stripe = new Stripe(process.env.STRIPE_SECRET_KEY);
export default async function handler(req, res) {
if (req.method === 'POST') {
try {
const { amount, paymentMethodId } = req.body;
const paymentIntent = await stripe.paymentIntents.create({
amount,
currency: 'usd',
payment_method: paymentMethodId,
confirm: true,
});
res.status(200).json({ success: true, paymentIntent });
} catch (error) {
res.status(400).json({ error: 'Payment failed.' });
}
} else {
res.status(405).json({ error: 'Method not allowed' });
}
}
This example demonstrates how to handle secure payment processing via Stripe using API Routes, providing a seamless checkout experience for your customers.
How Prateeksha Web Design Can Help
Prateeksha Web Design specializes in helping small businesses harness the power of modern technologies like Next.js API Routes. Here’s how we can assist:
- Customized Solutions: Whether you need an e-commerce backend, a custom dashboard, or third-party integrations, we build solutions tailored to your unique needs.
- Cost-Effective Development: We focus on serverless and scalable architectures, ensuring you get high-performance results without overspending.
- Expert Guidance: From initial strategy to implementation and maintenance, our team ensures a smooth development process, so you can focus on growing your business.
Conclusion: Empower Your Small Business with Next.js API Routes
For small businesses, adopting Next.js API Routes can be a transformative step toward building a powerful, scalable, and cost-effective online presence. Whether you need advanced e-commerce features, user-specific dashboards, or seamless integrations with third-party services, API Routes offer the flexibility and efficiency to get the job done.
With the expert guidance of Prateeksha Web Design, you can confidently leverage this technology to enhance your business operations, attract more customers, and stay ahead in today’s competitive digital landscape. Let us help you unlock the full potential of Next.js API Routes for your small business!
About Prateeksha Web Design
Prateeksha Web Design offers Next.js Image Component services that focus on optimizing images for improved website performance. Our team of program geeks ensures that images are resized, compressed, and served in the most efficient format. We utilize advanced techniques such as lazy loading and responsive design to enhance user experience. Let us help you make your website faster and more visually engaging with our image optimization services.
Interested in learning more? Contact us today.
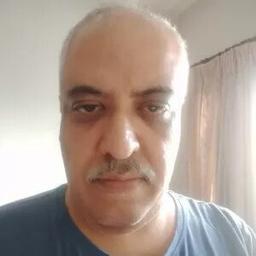