Table of Contents
- Introduction to Next.js and CMS Integration
- Choosing the Right CMS for Your Next.js Project
- Best Practices for API Integration Between CMS and Next.js
- Managing Content Efficiently with Next.js and CMS
- Enhancing Scalability in Next.js CMS Integration
- Security Best Practices for Next.js and CMS Integrations
- Improving SEO and Performance with Next.js and CMS
- Testing and Deployment Strategies
- Conclusion
Introduction to Next.js and CMS Integration
Next.js has rapidly emerged as a popular framework for building React-based web applications due to its server-side rendering (SSR), static site generation (SSG), and exceptional performance capabilities. Meanwhile, Content Management Systems (CMS) serve as a back-end system for managing content like blogs, landing pages, and product catalogs. When you combine Next.js with a CMS, it enables dynamic content management with a scalable, efficient workflow. In this blog, we’ll explore best practices for integrating a CMS with Next.js to ensure a seamless workflow that balances performance, scalability, and user-friendliness.
Why Next.js for CMS Integration?
- Versatility in rendering: It offers both SSR and SSG, which means your content can be rendered dynamically or at build time, offering flexibility depending on your needs.
- SEO-friendly: The framework is optimized for search engines, especially when combined with SSR.
- Scalability: Its modular structure makes it easier to integrate with any CMS while keeping performance intact.
Next, let’s dive into the key aspects that make this integration smooth and scalable.
Choosing the Right CMS for Your Next.js Project
Choosing the right CMS is pivotal to the success of your integration with Next.js. With a variety of headless CMSs available today, it’s essential to evaluate each CMS based on your project’s requirements, scalability, and ease of use.
Types of CMSs
- Headless CMS: A headless CMS decouples the content management from the presentation layer, giving developers more freedom to deliver content to different platforms. Popular choices include Strapi, Contentful, Sanity, and Prismic. Headless CMSs typically use an API-based approach to deliver content, which integrates well with Next.js.
- Traditional CMS: A traditional CMS like WordPress or Drupal includes both the content management and front-end layer. These can be integrated with Next.js using APIs, but headless CMSs generally offer better flexibility for developers.
Key Considerations for CMS Selection
- API flexibility: Ensure that the CMS provides RESTful or GraphQL APIs for fetching content easily. Next.js works seamlessly with both but GraphQL often offers more efficient data querying.
- Content modeling: The CMS should allow for robust content models that can scale with your project. Modular content types help you manage a variety of content formats.
- Editorial experience: Choose a CMS that provides an easy-to-use interface for non-technical editors to update and publish content.
Best Practices for API Integration Between CMS and Next.js
API integration is the backbone of any Next.js and CMS workflow, enabling communication between the CMS and the front-end application. Let’s look at best practices for setting up this integration:
1. Use Environment Variables
When connecting to a CMS API, you’ll need to secure sensitive data like API keys or tokens. Use environment variables to store this information securely and avoid hardcoding them directly into your project. In Next.js, you can manage environment variables through a .env
file.
NEXT_PUBLIC_API_URL=https://yourcmsapi.com
NEXT_PUBLIC_API_TOKEN=yourapitoken
2. Optimize API Requests
- Cache API responses: Caching API responses where possible can drastically reduce the number of calls made to the CMS, thus improving performance.
- Batch API requests: Instead of making multiple small requests, try to combine them into a single request to reduce latency and speed up the build process.
3. Use Incremental Static Regeneration (ISR)
Next.js supports Incremental Static Regeneration (ISR), which allows you to update static pages without rebuilding the entire site. This is useful for sites that need regular content updates but also require high performance.
export async function getStaticProps() {
const data = await fetchYourCMSContent();
return {
props: { data },
revalidate: 10, // Revalidate the page every 10 seconds
};
}
4. Choose Between REST and GraphQL
Both REST and GraphQL can be used to integrate your CMS with Next.js. However, GraphQL often provides more granular control over data fetching, allowing you to request only the fields you need. For larger projects, GraphQL can lead to more optimized and cleaner integrations.
Managing Content Efficiently with Next.js and CMS
Managing content efficiently is essential for long-term scalability and editor-friendliness. A well-structured CMS workflow with Next.js ensures that content updates are reflected seamlessly without developer intervention.
1. Modular Content Types
Set up modular content types in your CMS, allowing content to be easily reused across multiple pages or components. This approach saves time and ensures consistency.
For instance, in Contentful, you can create modules like Hero Banners, Call-to-Actions, and Blog Post Previews that can be mixed and matched across pages.
2. Draft Mode and Preview Feature
Enable draft mode and content previews within your Next.js site to allow editors to see unpublished content. Most CMS platforms provide preview APIs that integrate with Next.js.
export async function getServerSideProps(context) {
const preview = context.preview || false;
const data = await fetchCMSContent(preview);
return { props: { data, preview } };
}
3. Role-based Access Control
Ensure your CMS has role-based access control (RBAC) to allow different team members (e.g., editors, developers) to have appropriate permissions. This improves workflow efficiency by limiting access to only those who need it.
Enhancing Scalability in Next.js CMS Integration
Scalability is a major concern for large projects, especially as the content grows. Here’s how to design your CMS integration for scalability:
1. CDN for Content Delivery
Use a Content Delivery Network (CDN) to cache and deliver content faster. Most CMS platforms like Contentful and Sanity have built-in CDN support, which works well with Next.js's static site generation.
2. Pagination and Infinite Scroll
For large datasets, implement pagination or infinite scroll to improve load times and performance. Avoid loading large amounts of data on a single page.
export async function getServerSideProps({ query }) {
const page = query.page || 1;
const data = await fetchCMSContent({ page });
return { props: { data } };
}
3. Dynamic Imports
Leverage dynamic imports in Next.js to load components only when they’re needed, reducing the initial page load time.
const DynamicComponent = dynamic(() => import("../components/HeavyComponent"));
Security Best Practices for Next.js and CMS Integrations
Security is crucial when dealing with CMS integration, especially when sensitive content is involved. Follow these best practices to ensure your setup is secure:
1. API Authentication
Use OAuth, JWT, or API tokens to authenticate requests from Next.js to your CMS. Ensure that these tokens are securely stored and refreshed periodically.
2. HTTPS and Secure Headers
Ensure your Next.js application uses HTTPS to encrypt data. Set secure headers like Content Security Policy (CSP) and Strict-Transport-Security (HSTS) to mitigate potential security threats.
3. User Authentication for Admin Panels
When your Next.js application includes an admin panel for content management, ensure proper user authentication and role management to prevent unauthorized access.
Improving SEO and Performance with Next.js and CMS
One of the biggest advantages of using Next.js is its SEO optimization. Here’s how you can take it a step further when integrating with a CMS:
1. Server-Side Rendering for Better SEO
Next.js's server-side rendering (SSR) allows your content to be rendered on the server, improving its visibility to search engines.
2. Meta Tags and Open Graph Tags
Ensure that your pages include meta tags for SEO and Open Graph tags for social sharing. Use dynamic data from your CMS to populate these tags.
import Head from "next/head";
export default function BlogPost({ post }) {
return (
<Head>
<title>{post.title}</title>
<meta name="description" content={post.description} />
<meta property="og:title" content={post.title} />
<meta property="og:description" content={post.description} />
</Head>
);
}
3. Image Optimization
Next.js provides a built-in Image component that automatically optimizes images. Ensure that images from your CMS are properly formatted and sized to enhance load times.
Testing and Deployment Strategies
Proper testing and deployment strategies ensure that your Next.js and CMS integration is smooth and bug-free. Here’s what to keep in mind:
1. End-to-End Testing
Use tools like Cypress or Puppeteer to run end-to-end tests, ensuring that content is rendered correctly and APIs are functioning as expected.
2. Automated Deployment
Leverage platforms like Vercel (Next.js’s hosting provider) for automated deployment. Set up CI/CD pipelines to automatically deploy changes when content is updated in the CMS.
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Dependencies
run: npm install
- name: Build
run: npm run build
- name: Deploy
run: vercel --prod
Conclusion
Integrating a CMS with Next.js can provide a powerful, scalable, and user-friendly workflow for managing and delivering content. By following the best practices outlined in this guide, from selecting the right CMS to ensuring secure API integration and optimizing for SEO, you can create a seamless workflow that benefits both developers and content managers. Next.js’s flexibility combined with a robust CMS enables organizations to scale content efficiently while maintaining performance and security.
About Prateeksha Web Design
Prateeksha Web Design is a professional web design company that specializes in utilizing Next.js and CMS Integration to create smooth, seamless workflows for businesses. They excel in implementing best practices for efficient and effective web development and design.
Interested in learning more? Contact us today.
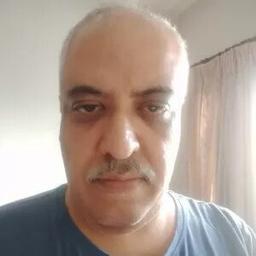