Mastering the Next.js Image Component: How to Optimize Your Web Visuals Effectively
Let’s face it: nothing kills a website vibe faster than slow-loading images. Imagine walking into a cafe and waiting an hour for a cup of coffee. Frustrating, right? The same goes for users visiting your website. They expect stunning visuals delivered at lightning speed. And guess what? With the Next.js image component, you can give them exactly that.
In this blog, we’ll take you through the nitty-gritty of web image optimization using the Next.js image component. We'll show you how to deliver jaw-dropping visuals without sacrificing performance. Whether you’re a rookie or someone dabbling in web design, this guide has you covered.
Oh, and before we dive in, a quick shoutout to Prateeksha Web Design! We’ve spent years mastering web design strategies, helping businesses deliver fast, responsive, and visually appealing websites. Let’s dive in, shall we?
What is the Next.js Image Component, and Why Should You Care?
When it comes to web development, visuals are non-negotiable. They’re the first thing your users notice, and they play a huge role in how engaging and professional your website feels. But here’s the catch: images are also one of the biggest culprits for slow-loading websites. Enter the Next.js Image Component, your secret weapon for optimizing visuals like a pro.
The Next.js image component isn’t just another way to display images. It’s a full-fledged toolset designed to optimize, deliver, and display images in the most efficient way possible. Imagine it as the Swiss Army knife of image optimization—it handles multiple tasks seamlessly, making your life easier and your website faster.
Key Features That Make the Next.js Image Component Awesome
-
Automatic Optimization
- No more fiddling with third-party tools to compress images manually.
- Next.js automatically compresses and resizes your images on the fly, ensuring they’re as small as possible without compromising quality.
- For example, if you upload a 5MB photo, the component shrinks it to a fraction of the size while maintaining its sharpness.
-
Responsive Design
- Your website visitors use a variety of devices—smartphones, tablets, laptops, and desktops.
- The image component generates and serves different versions of your image, optimized for the user’s screen size and resolution.
- Think of it as a tailor who creates the perfect fit for every client, whether they’re 5’2” or 6’5”.
-
Lazy Loading
- Traditional websites load all images upfront, even if the user never scrolls down to see them.
- Lazy loading ensures images are only loaded when they come into view. This speeds up initial page load times and improves user experience.
- It’s like having a buffet where food is served only when a diner arrives at the table—it keeps things efficient and prevents waste.
-
Cache-Friendly
- Next.js stores optimized images in a cache so that repeat visitors (or users navigating between pages) don’t need to re-download them. This speeds up the site even further.
- Imagine your favorite coffee shop remembering your order—it saves time and keeps things running smoothly.
-
Built-In Security
- Serving images from external sources? No problem. Next.js validates and optimizes them while ensuring they don’t pose security risks to your website.
Why Should You Care About All This?
Here’s why the Next.js image component matters for you, your users, and your business:
-
Speed Equals Retention
- A slow website is like a long queue—no one sticks around for it. Optimized images mean faster loading times, keeping users engaged and reducing bounce rates.
-
SEO Boost
- Search engines love fast websites. By using the image component, you’re indirectly improving your SEO and making it easier for your target audience to find you.
-
Mobile-Friendly
- With over half of web traffic coming from mobile devices, responsive and optimized images ensure your site looks stunning on any screen.
-
Improved Core Web Vitals
- Google measures your website’s performance with metrics like Largest Contentful Paint (LCP) and Cumulative Layout Shift (CLS). The Next.js image component helps you ace these scores.
-
Cost-Effective
- Hosting large, unoptimized images eats up server resources and bandwidth, potentially increasing your hosting costs. Efficient image delivery saves both time and money.
In short, the Next.js image component doesn’t just make your website look good—it makes it work brilliantly. It’s your all-in-one solution for delivering high-quality visuals without sacrificing performance, user experience, or SEO rankings.
With this tool, you don’t just display images; you optimize them in ways that keep users happy, search engines impressed, and your site lightning-fast.
Step 1: Setting Up the Next.js Image Component
Ready to get started? Let’s set up the foundation so you can start optimizing those visuals.
1. Install Next.js
First, create a Next.js project. If you’re new to this, don’t worry—it’s just a couple of commands.
npx create-next-app@latest my-nextjs-app
cd my-nextjs-app
npm run dev
2. Import the Image Component
Once your project is set up and running, you can start using the Image
component.
Here’s a simple import statement:
import Image from 'next/image';
3. Add an Image
Now let’s display an optimized image using the component:
<Image
src="/example.jpg"
alt="Example description"
width={800}
height={600}
/>
src
: Points to your image file. It can be a local file or an external URL.alt
: Describes the image for accessibility and SEO purposes.width
&height
: Define the dimensions of your image. Always include these to prevent layout shifts.
💡 Pro Tip: Store your images in the /public
folder of your project for easy access via relative paths like /example.jpg
.
Step 2: Basic Usage of the Next.js Image Component
The Next.js Image component is all about making your life easier while ensuring your visuals look and perform their best. Let’s walk through a basic example and break down how it works.
Here’s a simple code snippet:
<Image
src="/my-image.jpg"
alt="A stunning sunset"
width={800}
height={600}
/>
Breaking It Down
-
src
:- This is the source of your image.
- If your image is stored locally, use a relative path (e.g.,
/my-image.jpg
). Place the file in thepublic
folder of your project for easy access. - For external URLs, you’ll need to whitelist the domain in your configuration (more on that later).
-
alt
:- The
alt
attribute is essential for accessibility—it describes the image to screen readers for visually impaired users. - It also boosts your SEO. Search engines use
alt
text to understand the content of your images. - Example: Instead of a generic "sunset," use something descriptive like, "A stunning sunset over the mountains."
- The
-
width
&height
:- These define the dimensions of your image.
- Always specify them to avoid layout shifts, a common issue where page elements jump around while the image loads.
Why It Matters
Using exact dimensions isn’t just a "nice-to-have" feature. It directly impacts Cumulative Layout Shift (CLS), one of Google’s Core Web Vitals metrics. Preventing layout shifts improves both user experience and SEO performance.
💡 Pro Tip: If your image dimensions vary based on screen size, use CSS or dynamic properties to control them for responsiveness.
Step 3: Leverage Image Optimization Features
Once you’ve nailed the basics, it’s time to unlock the advanced features that make the Next.js Image component stand out.
1. Lazy Loading
By default, the Next.js Image component enables lazy loading. This means images are only fetched when they’re about to appear in the user’s viewport.
Imagine a dinner party where the butler serves appetizers just as guests are seated. It’s efficient and ensures nothing goes to waste. Similarly, lazy loading helps:
- Improve initial page load time.
- Save bandwidth by only loading images the user needs.
- Boost user engagement, especially for mobile users with slower connections.
Example of Lazy Loading in Action:
No extra configuration is needed! Lazy loading is baked into the component by default:
<Image
src="/banner.jpg"
alt="Hero banner"
width={1920}
height={1080}
/>
2. Adaptive Resizing
Next.js dynamically creates multiple versions of your image to cater to various screen sizes and device resolutions.
For example:
- A desktop user on a 4K monitor might receive a high-resolution image.
- A mobile user with a smaller screen and slower connection will get a lightweight version.
How It Works:
The Image component automatically handles this for you—no need for manual intervention. The magic happens through Next.js's Image Optimization API, which ensures every user gets the best possible version of your image.
3. External Image Support
Not all images live in your project’s public
folder. Sometimes, you need to fetch images from external sources, like a CMS or third-party server.
To enable optimization for external images, whitelist the domain in your next.config.js
file:
module.exports = {
images: {
domains: ['example.com', 'cdn.myimages.com'],
},
};
Why It’s Important
This step ensures that Next.js can optimize and securely deliver images from these sources, applying all the same benefits—compression, resizing, and caching—as it would for local images.
Example with an External Image:
<Image
src="https://example.com/external-image.jpg"
alt="External image example"
width={800}
height={600}
/>
💡 Pro Tip: If you’re working with a CMS like WordPress, Shopify, or Contentful, this feature is especially useful for fetching and optimizing images stored on their servers.
Step 4: Optimize Images Like a Pro
Now that you’ve got the basics down, let’s take it up a notch. Advanced image optimization can dramatically improve your site’s performance and user experience. These techniques ensure your visuals look fantastic while loading as efficiently as possible.
1. Use WebP Format
Think of WebP as the superhero of image formats. It provides:
- Smaller File Sizes: Compared to JPEG or PNG, WebP images are 25-35% smaller, reducing bandwidth usage.
- High Quality: Despite being smaller, WebP maintains excellent image quality.
How It Works in Next.js:
Next.js automatically detects if a browser supports WebP and serves it instead of the original format. If the browser doesn’t support WebP, it gracefully falls back to other formats like JPEG or PNG.
💡 Pro Tip: Use tools like Squoosh to manually convert images to WebP before uploading for even better control.
2. Preload Key Images
First impressions matter. Images above the fold (the part of the page users see immediately without scrolling) should load instantly. Preloading ensures these images are prioritized by the browser.
How to Preload in Next.js:
Use the priority
attribute with your Image component to tell the browser to fetch the image ASAP.
<Image
src="/hero-banner.jpg"
alt="Hero banner"
width={1920}
height={1080}
priority
/>
- When to Use: For hero images, logos, or any other visuals critical to the first screen your users see.
- Impact: This improves Largest Contentful Paint (LCP), a key performance metric in Google’s Core Web Vitals.
3. Avoid Oversized Images
Even the most powerful optimization tools can’t fix overly large source images. Always upload images that are close to their intended display size.
Best Practices:
- Use tools like TinyPNG, ImageOptim, or Kraken.io to compress images before uploading.
- If you’re exporting images from design tools like Photoshop or Figma, ensure the dimensions match your website’s needs.
- For vector images (like icons or logos), use the SVG format instead of PNG or JPEG for better scalability and performance.
💡 Pro Tip: A 10MB image file is like bringing a sledgehammer to hang a picture frame—overkill. Stick to optimized sizes for the best results.
Step 5: Combine Image Optimization with SEO Strategies
Optimizing images isn’t just about speed; it’s also about ensuring your site is discoverable by search engines. A well-optimized image can enhance your SEO, bringing in more organic traffic.
1. Descriptive Alt Text
Alt text is your chance to describe the image for both accessibility and SEO purposes. Include keywords naturally to boost your search rankings.
- Bad Example:
alt="Image1"
- Good Example:
alt="Next.js image optimization tutorial with a responsive design example"
Why It Matters:
Alt text helps screen readers describe images to visually impaired users and provides context for search engines to index the content.
2. Image File Names
Your image’s file name is another opportunity to include relevant keywords. A descriptive file name helps search engines understand the image’s content.
Examples:
- Bad:
IMG1234.jpg
- Good:
nextjs-image-optimization-example.jpg
💡 Pro Tip: Use hyphens to separate words in file names. Search engines treat hyphens as word separators but don’t recognize underscores.
3. Structured Data for Images
Structured data provides search engines with additional context about your images. It’s especially useful if your images are critical to your content, like product photos or infographics.
Example Schema Markup for an Image:
{
"@context": "https://schema.org/",
"@type": "ImageObject",
"contentUrl": "https://example.com/images/nextjs-image-optimization.jpg",
"creator": {
"@type": "Person",
"name": "John Doe"
},
"datePublished": "2025-01-01",
"description": "An optimized image showing Next.js responsive design in action."
}
Step 6: Test and Monitor Performance
Congratulations! Your website is now using optimized images. But the journey doesn’t end here—now it’s time to make sure everything runs as smoothly as a well-oiled machine. Testing and monitoring performance is critical to ensure your optimizations deliver real-world results.
Tools for Testing Your Website's Performance
-
Google Lighthouse
- Available in Chrome DevTools, Lighthouse provides detailed reports on performance, accessibility, SEO, and best practices.
- Use it to identify any bottlenecks caused by large images or slow loading times.
- Access it by opening Chrome DevTools (right-click > Inspect > Lighthouse tab) and running a test.
-
Google PageSpeed Insights
- This tool analyzes your website’s speed and provides actionable recommendations.
- It highlights key metrics like Largest Contentful Paint (LCP) and Cumulative Layout Shift (CLS), which are directly influenced by image optimization.
-
WebPageTest
- A more advanced testing tool that provides insights into image loading sequences, CDN effectiveness, and resource priorities.
-
Next.js Built-In Analytics
- Next.js offers its own analytics tools to monitor performance over time. Use them to identify areas where image optimization might be falling short.
Key Metrics to Watch
-
Largest Contentful Paint (LCP)
- Measures the time it takes for the largest visible element (often an image) to load.
- Goal: Aim for an LCP score of less than 2.5 seconds.
- Improvement Tips: Preload above-the-fold images using the
priority
attribute, use WebP formats, and optimize server response times.
-
Cumulative Layout Shift (CLS)
- Measures how much your page layout shifts as it loads. Images without defined dimensions are a major culprit.
- Goal: Keep your CLS score below 0.1.
- Improvement Tips: Always define
width
andheight
for images in the Next.js Image component.
-
First Contentful Paint (FCP)
- Measures how quickly the first piece of content appears on the screen. While not specific to images, optimizing critical visuals can improve this metric.
Common Mistakes to Avoid
Even with all these tools and tips, it’s easy to slip up. Here are some common pitfalls and how to steer clear of them:
1. Skipping Alt Tags
- Why It’s a Problem: Alt tags are vital for accessibility and SEO. They allow screen readers to describe images to visually impaired users and help search engines understand the content.
- Fix: Always add descriptive, keyword-rich alt text for every image.
2. Using Uncompressed Images
- Why It’s a Problem: Large, uncompressed images increase page load times, impacting user experience and SEO.
- Fix: Compress your images before uploading. Tools like TinyPNG, ImageOptim, or the Next.js Image Optimization API can help.
3. Overloading the Page
- Why It’s a Problem: Loading too many high-resolution images at once can overwhelm your server and slow down your website.
- Fix:
- Use lazy loading for images that aren’t immediately visible.
- Optimize your image selection—don’t use high-res images for tiny thumbnails.
- Consider a content delivery network (CDN) to distribute the load.
4. Forgetting to Test on Mobile
- Why It’s a Problem: Mobile users account for a significant portion of web traffic, and their connections may not be as fast as desktop users’.
- Fix: Always test your site’s performance on mobile devices using tools like Lighthouse or PageSpeed Insights.
5. Ignoring Third-Party Hosted Images
- Why It’s a Problem: If your site relies on external image sources (e.g., a CMS or API), unoptimized images can bottleneck your performance.
- Fix: Add the domain to your
next.config.js
file so Next.js can optimize those images too.
Why Prateeksha Web Design is Your Next.js Ally
Building a visually stunning, lightning-fast website takes expertise. At Prateeksha Web Design, we’ve perfected the art of crafting websites that blend performance with aesthetics. Whether it’s mastering Next.js visuals or optimizing every pixel, we’ve got you covered.
Our team specializes in:
- Seamless Next.js integrations
- Advanced image optimization strategies
- Custom solutions tailored to your business
Wrapping It Up
Optimizing web visuals with the Next.js image component doesn’t have to be daunting. With a few tweaks and some smart strategies, you can elevate your website to pro-level performance.
So, whether you’re running an e-commerce store, a portfolio site, or a blog, start optimizing those images today. Your users—and your Google rankings—will thank you.
Ready to take your website to the next level? Contact Prateeksha Web Design and let’s create something extraordinary together.
About Prateeksha Web Design
Prateeksha Web Design specializes in leveraging the Next.js Image Component to enhance web visuals with optimal performance. Their services include efficient image loading, automatic resizing, and format selection for improved speed and SEO. They focus on delivering a seamless user experience by utilizing responsive images that adapt to various devices. In addition, they implement lazy loading to minimize initial load time, ensuring a faster, more engaging website. Trust Prateeksha to transform your web visuals into a dynamic asset with cutting-edge optimization techniques.
Interested in learning more? Contact us today.
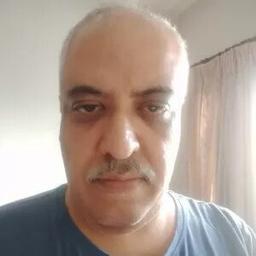