Welcome to the ultimate guide on mastering SOLID principles in C#! Whether you're just stepping into the world of C# development or you've been around long enough to have nightmares about spaghetti code, understanding SOLID programming principles is the key to writing clean, maintainable, and scalable software.
But hey, I get it—software design principles can sometimes feel like those cryptic puzzles in escape rooms. Don’t worry! We're about to break it all down in simple, engaging, and actionable steps, so you walk away feeling like a C# coding rockstar.
So, grab a coffee (or your beverage of choice), and let’s dive deep into the SOLID design principles—the secret sauce of robust object-oriented programming (OOP) in C#!
🚀 What Are SOLID Principles?
Before we get into the details, let’s talk about what SOLID principles actually are.
The term SOLID is an acronym representing five essential principles of object-oriented design. These principles, coined by Robert C. Martin (aka Uncle Bob), help in creating software that is scalable, maintainable, and easy to test.
Here’s the breakdown:
- S – Single Responsibility Principle (SRP)
- O – Open/Closed Principle (OCP)
- L – Liskov Substitution Principle (LSP)
- I – Interface Segregation Principle (ISP)
- D – Dependency Inversion Principle (DIP)
Now, let’s unpack each of these and see how they make your C# code rock solid!
🏆 1. Single Responsibility Principle (SRP) – “Do One Thing and Do It Well”
The Single Responsibility Principle (SRP) states that a class should only have one reason to change. In simpler terms, each class should be responsible for only one thing.
🤔 Why is this important?
Imagine you own a restaurant. You wouldn’t expect the chef to take orders, cook, serve, clean dishes, and manage accounts, right? That would be chaos! Similarly, in SOLID software principles, a class that does too much is a maintenance nightmare.
✅ Bad Example:
public class Invoice
{
public void CalculateTotal() { /*...*/ }
public void PrintInvoice() { /*...*/ }
public void SendEmail() { /*...*/ }
}
What’s wrong? This class does too much—it calculates totals, prints invoices, and sends emails.
💡 Good Example:
public class InvoiceCalculator
{
public void CalculateTotal() { /*...*/ }
}
public class InvoicePrinter
{
public void PrintInvoice() { /*...*/ }
}
public class EmailSender
{
public void SendEmail() { /*...*/ }
}
Each class now has one responsibility—which makes the code more manageable and easier to debug.
🚪 2. Open/Closed Principle (OCP) – “Open for Extension, Closed for Modification”
The Open/Closed Principle (OCP) suggests that a class should be open for extension but closed for modification.
🤔 Why does this matter?
Think of it like buying a smartphone. You can install new apps (extend functionality), but you don’t need to modify the internal OS.
✅ Bad Example:
public class PaymentProcessor
{
public void ProcessPayment(string type)
{
if (type == "CreditCard") { /*...*/ }
else if (type == "PayPal") { /*...*/ }
else if (type == "Crypto") { /*...*/ }
}
}
Adding a new payment method means modifying this class, which violates OCP.
💡 Good Example:
public interface IPaymentMethod
{
void ProcessPayment();
}
public class CreditCardPayment : IPaymentMethod
{
public void ProcessPayment() { /*...*/ }
}
public class PayPalPayment : IPaymentMethod
{
public void ProcessPayment() { /*...*/ }
}
public class PaymentProcessor
{
public void Process(IPaymentMethod paymentMethod)
{
paymentMethod.ProcessPayment();
}
}
Now, to add a new payment method, just create a new class without modifying the existing code.
🔄 3. Liskov Substitution Principle (LSP) – “Subtypes Must Behave Like Their Parent Types”
The Liskov Substitution Principle (LSP) states that subtypes should be replaceable for their base types without altering the correctness of the program.
🤔 Why is this important?
Imagine ordering a drink at a café. Whether it’s tea, coffee, or juice, you expect it to be served in a drinkable format. If suddenly, your “drink” came in the form of a solid ice cube, you’d be confused.
✅ Bad Example:
public class Rectangle
{
public virtual void SetWidth(int width) { /*...*/ }
public virtual void SetHeight(int height) { /*...*/ }
}
public class Square : Rectangle
{
public override void SetWidth(int width)
{
base.SetWidth(width);
base.SetHeight(width); // Violates LSP
}
}
A Square doesn’t behave like a Rectangle, leading to unexpected results.
💡 Good Example:
public interface IShape
{
int GetArea();
}
public class Rectangle : IShape
{
public int Width { get; set; }
public int Height { get; set; }
public int GetArea() => Width * Height;
}
public class Square : IShape
{
public int Side { get; set; }
public int GetArea() => Side * Side;
}
Now, both Rectangle
and Square
conform to LSP, making the design more robust.
🛠️ 4. Interface Segregation Principle (ISP) – “Don’t Force Unnecessary Methods”
The Interface Segregation Principle (ISP) states that a class should not be forced to implement methods it doesn’t use.
🤔 Why does this matter?
Imagine a Swiss Army Knife—it has tons of tools, but do you really need all of them all the time?
✅ Bad Example:
public interface IWorker
{
void Work();
void Eat();
}
public class Robot : IWorker
{
public void Work() { /*...*/ }
public void Eat() { throw new NotImplementedException(); } // Robots don’t eat!
}
💡 Good Example:
public interface IWorkable
{
void Work();
}
public interface IEatable
{
void Eat();
}
public class Robot : IWorkable
{
public void Work() { /*...*/ }
}
public class Human : IWorkable, IEatable
{
public void Work() { /*...*/ }
public void Eat() { /*...*/ }
}
Now, each class only implements what it needs, keeping things clean and efficient.
🔄 5. Dependency Inversion Principle (DIP) – “Depend on Abstractions, Not Concretions”
The Dependency Inversion Principle (DIP) suggests that high-level modules should not depend on low-level modules. Instead, both should depend on abstractions.
🤔 Why is this important?
Think of it like using a universal charger instead of one designed only for iPhones.
✅ Bad Example:
public class LightBulb
{
public void TurnOn() { /*...*/ }
}
public class Switch
{
private LightBulb bulb = new LightBulb();
public void Operate()
{
bulb.TurnOn(); // Tight coupling
}
}
💡 Good Example:
public interface IDevice
{
void TurnOn();
}
public class LightBulb : IDevice
{
public void TurnOn() { /*...*/ }
}
public class Switch
{
private IDevice device;
public Switch(IDevice device)
{
this.device = device;
}
public void Operate()
{
device.TurnOn(); // Loosely coupled
}
}
Now, Switch
works with any device, not just LightBulb
.
🚀 Taking the Next Steps: Applying SOLID Principles in Real-World C# Projects
Now that you’ve grasped the SOLID principles in C#, you’re on your way to writing cleaner, more scalable, and maintainable code. But theory alone won’t make you a C# master—you need to put these principles into practice.
Here’s how you can apply SOLID programming principles effectively in your projects:
🏗️ 1. Refactor Your Existing Codebase
Take a fresh look at your current C# projects. Identify places where classes are too big, where modifications break existing functionality, or where dependencies are tightly coupled. Refactor them step by step, applying one SOLID principle at a time.
🔹 Start with SRP: Break down classes that have too many responsibilities.
🔹 Check OCP: Ensure your code can be extended without modifying the base logic.
🔹 Ensure LSP Compliance: If a subclass is misbehaving, rethink its relationship with the parent class.
🔹 Apply ISP: If interfaces have too many methods, split them.
🔹 Refactor for DIP: If your high-level modules depend on low-level implementations, use interfaces and dependency injection.
🛠 Example: Refactoring a Poorly Designed Class
Before Refactoring:
public class ReportGenerator
{
public void GeneratePDF() { /*...*/ }
public void GenerateExcel() { /*...*/ }
public void SendReportByEmail() { /*...*/ }
}
This violates SRP—this class does too much.
After Refactoring:
public interface IReportFormat
{
void Generate();
}
public class PDFReport : IReportFormat
{
public void Generate() { /*...*/ }
}
public class ExcelReport : IReportFormat
{
public void Generate() { /*...*/ }
}
public class EmailSender
{
public void Send(string email, IReportFormat report) { /*...*/ }
}
Now, each class has a single responsibility, making the code easier to maintain.
🎯 2. Use Design Patterns That Align with SOLID Principles
Many design patterns naturally align with SOLID development principles. Some common patterns that complement SOLID code principles include:
✅ Factory Pattern – Helps follow OCP by creating objects without modifying existing code.
✅ Strategy Pattern – Supports DIP, allowing dynamic behavior changes.
✅ Repository Pattern – Improves SRP by separating data access logic from business logic.
✅ Decorator Pattern – Extends functionality without modifying existing code (follows OCP).
Example: Applying Factory Pattern to Follow OCP
public interface IPaymentMethod
{
void ProcessPayment();
}
public class CreditCardPayment : IPaymentMethod
{
public void ProcessPayment() { Console.WriteLine("Processing Credit Card Payment"); }
}
public class PayPalPayment : IPaymentMethod
{
public void ProcessPayment() { Console.WriteLine("Processing PayPal Payment"); }
}
public class PaymentFactory
{
public static IPaymentMethod CreatePayment(string type)
{
return type switch
{
"CreditCard" => new CreditCardPayment(),
"PayPal" => new PayPalPayment(),
_ => throw new Exception("Invalid Payment Type")
};
}
}
// Usage:
IPaymentMethod payment = PaymentFactory.CreatePayment("PayPal");
payment.ProcessPayment();
This ensures we can add new payment methods without modifying existing code.
📦 3. Implement Dependency Injection (DI) in Your C# Applications
Applying Dependency Inversion Principle (DIP) in real-world applications is easier when you use Dependency Injection (DI) frameworks like:
- Microsoft’s Built-in DI Container (ASP.NET Core)
- Autofac
- Ninject
Example: Using DI in an ASP.NET Core App
public interface IMessageService
{
void SendMessage(string message);
}
public class EmailService : IMessageService
{
public void SendMessage(string message)
{
Console.WriteLine($"Email sent: {message}");
}
}
public class NotificationController
{
private readonly IMessageService _messageService;
public NotificationController(IMessageService messageService)
{
_messageService = messageService;
}
public void NotifyUser()
{
_messageService.SendMessage("Welcome to our service!");
}
}
// In Startup.cs
services.AddTransient<IMessageService, EmailService>();
With DI, you can easily swap out implementations without modifying the core business logic—DIP in action!
🌎 4. Use SOLID Principles in Your Web Development Projects
If you're working with web applications, these principles still apply! At Prateeksha Web Design, we follow SOLID software development principles to create:
✅ Scalable eCommerce Websites
✅ SEO-Friendly Business Websites
✅ Custom Web Applications
Looking for the best web design company in Mumbai? We help businesses develop websites in Mumbai, specializing in eCommerce website design and development. Whether you're building a Shopify store or a Next.js web app, we follow solid object-oriented design principles to ensure high performance!
🎯 Conclusion
Mastering SOLID principles in C# helps you write better, cleaner, and more scalable code. Start applying these principles to your C# projects today!
Need a professional website? Prateeksha Web Design is the best web design company in Mumbai—helping businesses build stunning websites and eCommerce solutions.
Ready to write SOLID code? Get coding now! 🚀
About Prateeksha Web Design
Prateeksha Web Design offers tailored services for mastering Solid Principles in C#, providing a comprehensive step-by-step practical guide. Their solutions include interactive tutorials, personalized coaching sessions, and hands-on coding workshops. Clients benefit from real-world examples and project-based learning to solidify their understanding. Additionally, they provide resources such as code snippets and best practices to enhance ongoing development. Experience a collaborative learning environment that fosters skill mastery and confidence in C#.
Interested in learning more? Contact us today.
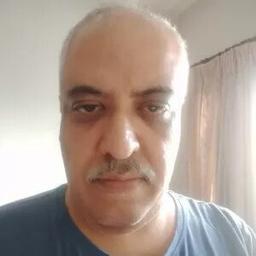