Introduction: Why Arrows Matter in PDFs (And How WPF Can Help You Master Them!)
Ever found yourself staring at a PDF document, wishing you could add a simple arrow to highlight something important? Maybe you want to point out a crucial detail in a business report or make annotations on a design file? Whatever the case, mastering arrow shapes in PDFs is a skill worth having—especially if you're working with WPF (Windows Presentation Foundation).
But let’s be real—modifying PDFs can feel like trying to solve a Rubik’s cube blindfolded. 🤯 Luckily, with the right approach, it doesn’t have to be. In this guide, we’ll break down how to add, edit, and remove arrows in PDF files using WPF.
And since you’re here, we won’t just stop at arrows. We’ll also explore how to erase elements on a PDF, create a table of contents programmatically in Word, and even modify the TOC dynamically. Sounds exciting? Let’s dive in!
1. Adding Arrows to a PDF: Drawing Like a Pro 🎯
Let’s start with the basics—how to add arrows in a PDF using WPF.
Why Arrows?
Arrows are great for:
✅ Highlighting key points in a document
✅ Directing attention to a specific section
✅ Annotating PDFs for better readability
How to Draw an Arrow in a PDF Using WPF
To draw an arrow in a PDF, you’ll need a WPF application and a good PDF library like PDFsharp or Syncfusion. Here's a simple way to do it:
using PdfSharp.Drawing;
using PdfSharp.Pdf;
// Create a PDF document
PdfDocument document = new PdfDocument();
PdfPage page = document.AddPage();
XGraphics gfx = XGraphics.FromPdfPage(page);
// Define the arrow points
XPoint startPoint = new XPoint(100, 100);
XPoint endPoint = new XPoint(200, 100);
// Draw a line (arrow shaft)
gfx.DrawLine(XPens.Black, startPoint, endPoint);
// Draw the arrowhead
gfx.DrawLine(XPens.Black, endPoint, new XPoint(190, 90));
gfx.DrawLine(XPens.Black, endPoint, new XPoint(190, 110));
// Save the document
document.Save("output.pdf");
document.Close();
🔹 What’s Happening Here?
- We create a new PDF document.
- We add a new page and start drawing using
XGraphics
. - We draw an arrow shaft and use two extra lines to form the arrowhead.
- Finally, we save the document as a PDF.
Boom! You’ve got yourself an arrow in a PDF! 🎉
2. Removing Arrows and Other Annotations from a PDF
Maybe you’ve accidentally added an arrow (oops! 😅) or want to clean up a document before sharing it. You need a way to remove arrows from a PDF.
How to Remove Arrows and Annotations
To erase an arrow (or any other shape) in a PDF, you’ll need a PDF library that supports annotation removal. Here’s how you can do it:
using Syncfusion.Pdf;
using Syncfusion.Pdf.Parsing;
// Load an existing PDF
PdfLoadedDocument document = new PdfLoadedDocument("input.pdf");
PdfLoadedPage page = document.Pages[0] as PdfLoadedPage;
// Remove all annotations (including arrows)
page.Annotations.Clear();
// Save and close the document
document.Save("output_clean.pdf");
document.Close(true);
🔹 Quick Breakdown:
- We load the existing PDF.
- We clear all annotations, including arrows.
- We save the modified document.
And just like that, the arrows are gone! 🎩✨
💡 Bonus Tip: If you only want to remove specific arrows, you can loop through page.Annotations
and check for their type before deleting them.
3. Creating a Table of Contents in Word Programmatically
Now that you’ve mastered arrows in PDFs, let’s take it a step further. Ever had to create a table of contents (TOC) in Word manually? Painful, right? 😵 Instead, let’s automate it!
How to Programmatically Create a Table of Contents in Word
Using C# and OpenXML, you can generate a dynamic TOC like this:
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Wordprocessing;
// Create a Word document
using (WordprocessingDocument doc = WordprocessingDocument.Create("TOC_Document.docx", WordprocessingDocumentType.Document))
{
MainDocumentPart mainPart = doc.AddMainDocumentPart();
mainPart.Document = new Document(new Body());
Body body = mainPart.Document.Body;
Paragraph tocPara = new Paragraph(new Run(new Text("Table of Contents")));
body.AppendChild(tocPara);
// Add TOC field
Paragraph toc = new Paragraph(new Run(new FieldChar { FieldCharType = FieldCharValues.Begin }));
body.AppendChild(toc);
doc.Save();
}
🔹 Key Takeaways:
✅ You can generate a TOC dynamically using OpenXML.
✅ This saves time and ensures updated page numbers automatically.
💡 Need to modify the TOC later? Just update the field in Word!
4. Erasing Elements on a PDF Document: A Deep Dive
Why Erase Elements in a PDF?
There are many reasons why you might need to erase or remove specific content from a PDF document. Some common use cases include:
✅ Redacting sensitive information (such as confidential data or personal details)
✅ Correcting mistakes (like typos or outdated information)
✅ Removing unwanted annotations, drawings, or shapes (such as arrows, text boxes, or highlights)
✅ Cleaning up a document before publishing or sharing
Since PDFs are not as easy to edit as Word documents, removing elements can be tricky. While you might think of "deleting" elements, most PDF libraries do not offer a built-in delete function for specific parts of the document. Instead, the best way to "erase" something is to cover it up with a white rectangle—effectively acting as an eraser.
How to Erase Elements in a PDF Using WPF and PDFsharp
When working with PDFsharp, a popular open-source library for PDF manipulation, you can erase elements by drawing a white rectangle over them. This method works because:
- PDF content is static and cannot be selectively deleted like a Word document.
- Instead, you overlay a new shape on top of the content you want to hide.
- The white color blends with the background, making the covered content appear erased.
Example: Erasing a Section of a PDF
Here’s a C# code snippet using PDFsharp to remove a section of a PDF:
using PdfSharp.Drawing;
using PdfSharp.Pdf;
using PdfSharp.Pdf.IO;
class Program
{
static void Main()
{
// Load the existing PDF document
PdfDocument document = PdfReader.Open("input.pdf", PdfDocumentOpenMode.Modify);
// Select the first page
PdfPage page = document.Pages[0];
// Create graphics object to edit the page
XGraphics gfx = XGraphics.FromPdfPage(page);
// Define the rectangle to "erase" (X, Y, Width, Height)
XRect eraseArea = new XRect(100, 100, 200, 50);
// Draw a white rectangle over the unwanted content
gfx.DrawRectangle(XBrushes.White, eraseArea);
// Save the modified document
document.Save("output_erased.pdf");
// Close the document
document.Close();
}
}
How This Code Works
-
Load an Existing PDF:
- The
PdfReader.Open("input.pdf", PdfDocumentOpenMode.Modify);
function loads a PDF for editing. PdfDocumentOpenMode.Modify
ensures we can make changes to the document.
- The
-
Select a Page to Edit:
document.Pages[0]
selects the first page of the document.- You can loop through multiple pages if needed.
-
Create a Graphics Object (
XGraphics
) for Drawing on the PDF:XGraphics.FromPdfPage(page);
enables you to draw on the PDF page.
-
Define the Area to Be Erased:
XRect eraseArea = new XRect(100, 100, 200, 50);
- This defines a rectangle of width 200px and height 50px, starting from coordinates (100,100).
- The X and Y coordinates represent the top-left corner of the rectangle.
-
Draw a White Rectangle to Cover the Content:
gfx.DrawRectangle(XBrushes.White, eraseArea);
creates a white overlay that acts as an eraser.
-
Save and Close the PDF:
- The modified document is saved as
"output_erased.pdf"
, effectively removing the selected content.
- The modified document is saved as
How to Identify What Needs to Be Erased?
Before using this method, you need to determine where the content is located in your PDF. There are a few ways to do this:
-
Manually Estimate Coordinates
- Open the PDF in Adobe Acrobat or another viewer.
- Use the ruler tool to estimate the X, Y coordinates of the area you want to erase.
- Adjust the values in
XRect(x, y, width, height)
accordingly.
-
Use a PDF Library to Extract Text Locations
- If you need to erase specific text rather than a fixed area, you might use a library like iTextSharp or PdfSharp to locate text coordinates dynamically before drawing a white rectangle.
Additional Considerations
🔹 What if the Background is Not White?
-
If the PDF has a colored background, a white rectangle might stand out.
-
In that case, use the same color as the background instead of
XBrushes.White
. -
Example: If the background is light gray (
#F5F5F5
), use:gfx.DrawRectangle(new XSolidBrush(XColor.FromArgb(245, 245, 245)), eraseArea);
🔹 Can I Erase Multiple Areas?
-
Yes! Just repeat the
gfx.DrawRectangle
function with different coordinates:gfx.DrawRectangle(XBrushes.White, new XRect(100, 100, 200, 50)); gfx.DrawRectangle(XBrushes.White, new XRect(300, 150, 100, 30));
🔹 What If I Want to Remove Specific Shapes (Like Arrows)?
-
Instead of erasing everything in a fixed area, you can loop through annotations in a PDF and remove them selectively:
using Syncfusion.Pdf; using Syncfusion.Pdf.Parsing; // Load an existing PDF PdfLoadedDocument document = new PdfLoadedDocument("input.pdf"); PdfLoadedPage page = document.Pages[0] as PdfLoadedPage; // Remove all annotations (including arrows) page.Annotations.Clear(); // Save and close the document document.Save("output_clean.pdf"); document.Close(true);
5. Web Design Services in Mumbai: Why Choose Prateeksha Web Design?
So, you’ve nailed PDF editing in WPF—nice! 🎯 But let’s switch gears for a second. Whether you’re an entrepreneur, a startup founder, or an established business, having a high-quality website is just as crucial as handling PDFs properly. If you’re looking for the best web design company in Mumbai, Prateeksha Web Design is your go-to solution.
🚀 Why? Because your website is your digital storefront. It’s where customers form their first impression, make purchasing decisions, and interact with your brand. So, you don’t just need a website—you need a website that performs, converts, and looks stunning.
What Makes Prateeksha Web Design the Best Web Design Agency in Mumbai?
✅ Custom eCommerce Website Design in Mumbai
- Whether you’re launching a Shopify store, WooCommerce shop, or custom eCommerce platform, we build tailor-made solutions that fit your brand.
- We don’t use cookie-cutter templates—we design unique, conversion-focused stores.
✅ SEO-Friendly Website Development
- What’s the point of a website if no one can find it? 🤷♂️
- Our sites are built SEO-first to help your business rank higher on Google and attract organic traffic.
✅ Modern UI/UX to Attract More Customers
- A slow, clunky, or outdated website is an instant turnoff. We create sleek, user-friendly, and high-performance websites that keep visitors engaged.
- Whether it’s mobile-friendly layouts or intuitive navigation, we optimize for maximum user experience.
✅ Lightning-Fast Performance
- Slow websites kill conversions. 🚀 We optimize for speed and performance, ensuring your site loads in under 3 seconds.
✅ Responsive Across All Devices
- With most users browsing on mobile devices, your website needs to look great on all screen sizes.
- We ensure your website is fully responsive, delivering a seamless experience on mobile, tablet, and desktop.
✅ Affordable Web Design Services in Mumbai
- High-quality web design doesn’t have to break the bank. We offer cost-effective solutions without compromising on quality.
Ecommerce Website Development in Mumbai: Build, Launch & Scale 🚀
If you’re in Mumbai and looking for an eCommerce website development company, Prateeksha Web Design is the perfect fit. We handle:
- Shopify Store Development
- WooCommerce & WordPress eCommerce Development
- Custom eCommerce Web Design
- Multi-Vendor Marketplace Development
- Ecommerce SEO & Digital Marketing
💡 Need an eCommerce store? Contact us for expert web design services in Mumbai!
Web Design Services in Mumbai: Our Expertise Covers Everything!
If you’re searching for:
🔹 Best Web Design Company in Mumbai
🔹 Web Design Agency in Mumbai
🔹 Website Design in Mumbai
🔹 Web Designing Company in Mumbai
🔹 Web Developer Mumbai
🔹 Website Development Company Mumbai
You’ve found the right team! 💪
We cater to businesses, startups, agencies, and entrepreneurs looking for high-performance websites that drive sales and engagement.
Why Invest in a High-Quality Website?
📌 75% of users judge a company’s credibility based on its website—first impressions matter!
📌 A fast, well-designed, and SEO-optimized site can increase conversions by up to 200%.
📌 In today’s digital world, your website is your brand’s most important asset.
Let’s Build Something Amazing Together!
🚀 Ready to develop a website in Mumbai that stands out? We’re here to help.
📩 Contact Prateeksha Web Design today to get started with:
✅ Custom Web Design
✅ Ecommerce Development
✅ SEO & Performance Optimization
Let’s create something extraordinary for your business! 🌟
Conclusion: Take Control of Your PDFs & Word Docs Like a Pro!
We’ve covered how to add, edit, and remove arrows in PDFs, along with creating and modifying TOCs in Word programmatically. Whether you’re an aspiring developer or a business owner who needs PDF annotations, these skills will level up your workflow.
And if you need a stunning website, trust Prateeksha Web Design, your go-to web designing company in Mumbai. 🚀
📢 What’s Next?
✅ Try adding arrows to a PDF using WPF
✅ Automate a TOC in Word and see how it works
✅ Need a website? Reach out to the best web design company in Mumbai today!
About Prateeksha Web Design
Prateeksha Web Design offers specialized services in developing WPF applications for mastering arrow functions in PDF files. Our solutions enable users to seamlessly create, edit, and delete shapes, enhancing document interactivity and visual clarity. With a focus on intuitive design and robust functionality, we ensure a user-friendly experience. Our team is dedicated to delivering customized features tailored to client needs. Elevate your PDF management with our expert WPF implementations.
Interested in learning more? Contact us today.
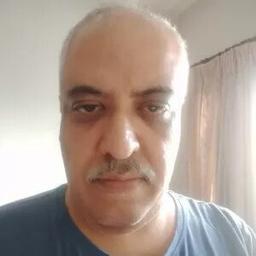