Embarking on your coding journey can feel overwhelming, especially when faced with endless programming languages, frameworks, and tools. But here’s the good news: with the right guidance and mindset, anyone can learn to code! This beginner’s guide will not only break down the steps to get you started but also ground the discussion in recent advancements in technology. So, let’s dive in and unlock your programming potential!
Why Learn to Code in 2024?
In 2024, learning to code is more than just a technical skill—it's a gateway to innovation, creativity, and adaptability in a rapidly evolving digital world. Coding is no longer confined to traditional tech roles; it is now a critical competency across various industries. Whether you aspire to become a software developer, create your own apps, or simply enhance your problem-solving abilities, learning to code opens doors to countless opportunities.
The Importance of Coding in Today’s World
- Problem-Solving Skills: Coding teaches you how to break complex problems into smaller, manageable parts. This skill is invaluable not just in tech but in everyday decision-making and project management.
- Career Opportunities: With digital transformation at its peak, the demand for developers, data scientists, and automation specialists continues to rise. Coding gives you a competitive edge in industries like healthcare, finance, marketing, and more.
- Entrepreneurship and Innovation: Knowing how to code allows you to turn your ideas into reality, whether it’s launching a startup, creating a new product, or automating tedious tasks.
- Interdisciplinary Applications: Coding skills are increasingly valuable in fields like art, design, education, and even social sciences, enabling professionals to push the boundaries of their disciplines.
Latest Advancements in the Coding Landscape
The coding world has seen exciting advancements in recent years, making it easier, faster, and more accessible for beginners to start their journey. Here’s a closer look at the technologies shaping coding in 2024:
-
AI-Powered Development Tools
- What Are They? AI-powered tools like GitHub Copilot and ChatGPT act as coding assistants, helping developers write, debug, and optimize code more efficiently.
- How Do They Help Beginners? These tools provide real-time suggestions, explain code snippets, and offer best practices, reducing the learning curve for new programmers.
- Key Features:
- Auto-completing code as you type.
- Providing detailed explanations for complex programming concepts.
- Debugging and identifying errors with recommendations for fixes.
- Why It Matters in 2024: AI tools enable beginners to experiment and learn in a supportive environment, making coding less intimidating and more engaging.
-
Low-Code and No-Code Platforms
- What Are They? Platforms like Webflow, Bubble, and Appgyver allow users to create applications and websites with minimal or no programming knowledge.
- How Do They Work? These platforms use drag-and-drop interfaces, pre-built components, and visual workflows to build digital products.
- For Beginners: Low-code platforms are a great starting point for understanding the basics of application logic and user interface design without diving into complex syntax.
- Real-World Applications:
- Building a personal portfolio or blog.
- Prototyping an app idea before full development.
- Automating small business workflows.
- Why It Matters in 2024: With the rise of these platforms, even non-programmers can create professional-grade solutions, democratizing technology development.
-
Open Source Growth
- What is Open Source? Open source refers to software and tools whose source code is freely available for anyone to view, modify, and distribute.
- Why Is It Important for Beginners? Open-source projects on platforms like GitHub and GitLab provide free learning resources, real-world examples, and opportunities to collaborate with experienced developers.
- Current Trends in Open Source:
- More beginner-friendly documentation and tutorials.
- Thousands of repositories dedicated to teaching coding basics (e.g., Python learning kits, JavaScript project templates).
- Community-driven initiatives to mentor newcomers.
- Why It Matters in 2024: Open-source communities are thriving, providing an inclusive space for beginners to learn, contribute, and grow.
Step 1: Understand the Basics of Programming
Before diving into coding, understanding the fundamentals of programming is essential. Think of programming as learning a new language—but instead of communicating with people, you're communicating with computers to solve problems or automate tasks.
Key Concepts to Learn First
-
What is Programming?
- Programming is the act of creating instructions for a computer to execute specific tasks. These instructions are written in a programming language and serve as the "recipe" for the computer.
- Think of it this way: You’re teaching the computer step-by-step how to solve a problem, like telling it how to calculate your grocery bill or build a game.
-
Core Programming Elements To begin your coding journey, start with these foundational concepts:
- Variables:
- A variable is like a storage box for data. It holds information (e.g., numbers, words) that can be used or changed during a program.
- Example in Python:
age = 25 # A variable storing the number 25 name = "Alice" # A variable storing the text "Alice"
- Control Structures:
- Control structures guide the flow of a program. The two most common types are:
- Loops: Repeat a task until a condition is met (e.g., printing numbers 1 to 10).
- Conditionals: Make decisions based on logic (e.g., if it’s raining, bring an umbrella).
- Example in Python:
for i in range(1, 6): # A loop printing numbers from 1 to 5 print(i) if age > 18: # A conditional checking age print("You are an adult.")
- Control structures guide the flow of a program. The two most common types are:
- Functions:
- Functions are reusable blocks of code designed to perform specific tasks. Instead of repeating the same code, you write a function and call it whenever needed.
- Example:
def greet(name): print(f"Hello, {name}!") greet("Alice") # Outputs: Hello, Alice!
- Example:
- Functions are reusable blocks of code designed to perform specific tasks. Instead of repeating the same code, you write a function and call it whenever needed.
- Data Structures:
- Data structures like arrays, lists, dictionaries, and sets help organize and manage data efficiently.
- Example in Python:
fruits = ["apple", "banana", "cherry"] # A list of fruits scores = {"Alice": 90, "Bob": 85} # A dictionary of scores
- Example in Python:
- Data structures like arrays, lists, dictionaries, and sets help organize and manage data efficiently.
- Variables:
-
Popular Programming Languages
- Choosing the right language depends on your interests. Here’s a quick overview:
- Python: Known for its readability, Python is great for beginners and widely used in AI, automation, and web development.
- JavaScript: Essential for creating dynamic websites and interactive web pages.
- Java: A robust language for large-scale applications, such as Android apps and enterprise software.
- C++: A high-performance language often used for gaming, system-level programming, and applications requiring speed.
- Choosing the right language depends on your interests. Here’s a quick overview:
Step 2: Choose the Right Programming Language
Selecting the right programming language as a beginner is critical to keeping your journey both productive and enjoyable. Your choice should align with your goals and interests.
Goals and Best Language Matches:
-
- Start with HTML, CSS, and JavaScript.
- HTML structures your content, CSS styles it, and JavaScript makes it interactive.
- Example:
- HTML: Adds a button to a webpage.
- CSS: Styles the button.
- JavaScript: Makes the button clickable to display a message.
-
Data Science or AI:
- Go for Python because of its robust libraries (like NumPy, pandas, and TensorFlow) and straightforward syntax.
- Example in Python:
import pandas as pd data = {"Name": ["Alice", "Bob"], "Score": [95, 85]} df = pd.DataFrame(data) print(df)
-
App Development:
- Use Java for Android apps or Swift for iOS apps.
- Java is versatile for mobile and backend development, while Swift is optimized for Apple’s ecosystem.
- Example:
- Java: Building a weather app for Android.
- Swift: Creating an iPhone fitness tracker.
-
Game Development:
- Explore C++ or Unity with C#.
- C++ provides low-level control, essential for gaming engines. Unity, powered by C#, simplifies creating interactive 2D and 3D games.
- Example in C++:
#include <iostream> using namespace std; int main() { cout << "Welcome to the Game!"; return 0; }
Why Python is Great for Beginners
Python stands out as the most beginner-friendly language due to its simple syntax that resembles natural English. Its readability reduces the intimidation factor, allowing you to focus on learning concepts rather than wrestling with complex syntax.
-
Ease of Use: Writing a “Hello, World!” program in Python requires just one line:
print("Hello, World!")
-
Extensive Libraries: Python has libraries like NumPy for numerical computing, pandas for data manipulation, and Flask for web development, making it highly versatile.
-
Supportive Community: Python’s large community means abundant tutorials, forums, and open-source projects for beginners to learn from.
-
Future-Ready: Python is a leading language in cutting-edge fields like AI, machine learning, and data science. Mastering it prepares you for the jobs of tomorrow.
Step 3: Set Up Your Coding Environment
Before you start writing code, it’s essential to create an environment where you can easily write, test, and debug your programs. Think of your coding environment as the workspace where all the magic happens. Here's a detailed breakdown of the tools you need:
Tools You Need:
-
Code Editor:
- A code editor is a lightweight tool where you write and edit your code. It’s like a digital notebook designed specifically for coding.
- Top Recommendations:
- Visual Studio Code (VS Code):
- Known for its flexibility and powerful extensions.
- Supports multiple programming languages.
- Features include syntax highlighting, auto-completion, and built-in terminal.
- Popular extensions: Prettier (code formatter), ESLint (error checking), and Python extension.
- Sublime Text:
- Lightweight and fast.
- Ideal for beginners who want a clean and straightforward editor.
- Supports multiple languages with minimal setup.
- Visual Studio Code (VS Code):
- Why These Editors? Both are beginner-friendly, customizable, and widely used by professionals.
-
Integrated Development Environment (IDE):
- IDEs are like advanced code editors with added tools to make development smoother. They include features like debugging, project management, and real-time error detection.
- Top Recommendations:
- PyCharm: Perfect for Python developers with features like intelligent code completion and debugging tools.
- IntelliJ IDEA: Ideal for Java and Kotlin, offering excellent code navigation and refactoring tools.
- Who Should Use IDEs? If you're focusing on larger projects or specific languages like Python or Java, IDEs are invaluable.
-
Version Control:
- Version control allows you to track changes to your code and collaborate with others effectively.
- Learn Git:
- Git is the most popular version control system. It helps you save snapshots of your code, called "commits," and revert to them if something breaks.
- Collaborate with GitHub:
- GitHub is an online platform where you can store your code, collaborate with others, and showcase your projects.
- Beginners can start with GitHub Desktop, which provides a graphical interface to manage repositories.
Example Workflow:
- Write code in VS Code or PyCharm.
- Save and commit changes using Git.
- Push your project to GitHub for backup or collaboration.
Step 4: Learn Through Hands-On Practice
Understanding theory is important, but nothing beats learning by doing. Hands-on practice helps you understand concepts, debug errors, and build real-world skills.
Start with Simple Projects:
Begin with small, achievable projects to build confidence and gradually increase complexity.
-
To-Do List App:
- A beginner-friendly project that introduces you to creating, reading, updating, and deleting tasks.
- Languages to use: JavaScript (with HTML and CSS) or Python.
- Example:
tasks = [] def add_task(task): tasks.append(task) add_task("Learn Python") print(tasks)
-
Calculator:
- Learn how to handle user inputs and perform basic mathematical operations.
- Languages to use: Python or JavaScript.
- Example in Python:
def add(a, b): return a + b print(add(3, 5)) # Outputs: 8
-
Personal Website:
- Build a simple website to showcase your portfolio or hobbies.
- Tools to use: HTML, CSS, JavaScript.
- Example: Create a basic HTML page and style it with CSS.
<h1>Welcome to My Website</h1> <p>Hello! I'm learning to code.</p>
Use Online Platforms for Practice:
- FreeCodeCamp:
- Offers structured courses for web development, Python, and more.
- Projects and certifications help solidify learning.
- HackerRank:
- Ideal for practicing coding challenges and problem-solving.
- Topics range from beginner to advanced.
- Codewars:
- Gamified coding platform where you solve puzzles and compete with other coders.
Step 5: Explore Advanced Topics Gradually
Once you’ve mastered the basics, it’s time to level up by exploring advanced programming concepts. These skills prepare you for larger, real-world projects and give you a deeper understanding of programming.
-
Object-Oriented Programming (OOP):
- OOP organizes your code around objects (data) and classes (blueprints for objects).
- Why Learn OOP?
- Makes code reusable and scalable.
- Essential for working with frameworks and libraries.
- Example in Python:
class Person: def __init__(self, name, age): self.name = name self.age = age def greet(self): print(f"Hello, my name is {self.name}") alice = Person("Alice", 25) alice.greet() # Outputs: Hello, my name is Alice
-
APIs (Application Programming Interfaces):
- APIs allow your program to interact with external systems or services.
- Example: Using an API to fetch weather data.
import requests response = requests.get("https://api.openweathermap.org/data/2.5/weather?q=London&appid=YOUR_API_KEY") print(response.json())
-
Frameworks:
- Frameworks simplify development by providing pre-written code for common tasks.
- Examples:
- React (JavaScript): Build interactive web interfaces.
- Flask or Django (Python): Create web applications quickly.
- Benefits:
- Accelerate development by reducing boilerplate code.
- Encourage best practices.
The Role of Community and Collaboration
Learning to code can be challenging at times, especially when you hit a roadblock or feel stuck. That’s where the power of community and collaboration comes in. Being part of a coding community not only provides support but also accelerates your learning by exposing you to diverse perspectives and experiences.
Why Join a Coding Community?
-
Peer Support:
- Communities offer a safe space to ask questions and get answers from people who’ve been in your shoes. They eliminate the fear of judgment, which is common among beginners.
- Example: If you’re stuck debugging a program, someone in your community might have faced the same issue and can guide you to a solution.
-
Learning from Others:
- By collaborating on projects, you’ll learn how to write cleaner, more efficient code. Reviewing others' code helps you spot patterns and techniques you might not have thought of.
- Exposure to different coding styles and practices enhances your skills and broadens your perspective.
-
Motivation and Accountability:
- Sharing your progress with others motivates you to stay consistent. Communities often organize coding challenges, hackathons, and meetups to keep members engaged.
-
Networking Opportunities:
- Joining a coding community can connect you with mentors, industry professionals, and potential employers. Relationships built in these communities often lead to internships, job opportunities, or collaborations on exciting projects.
Best Communities for Coding:
Here are some of the most vibrant and supportive communities for programmers:
-
Reddit:
- Subreddits like r/learnprogramming, r/Python, and r/webdev are great places to ask questions, find resources, and engage with other learners.
- Benefits:
- Anonymity allows you to ask beginner questions without fear.
- Access to a global community with diverse expertise.
-
Stack Overflow:
- Known as the go-to Q&A platform for developers.
- Features:
- Search for answers to common technical issues or post your own question.
- Engage with professionals who often provide detailed, step-by-step solutions.
- Pro Tip: Always search for existing answers before asking a question to avoid duplicate threads.
-
GitHub:
- GitHub is more than just a version control platform; it’s a thriving community of open-source developers.
- Why Join:
- Contribute to open-source projects to gain real-world experience.
- Learn best practices by studying the code of professional developers.
- Build a portfolio that showcases your contributions to potential employers.
-
Discord and Slack Groups:
- Many coding communities have dedicated Discord or Slack channels for real-time collaboration and support.
- Examples:
- FreeCodeCamp’s Discord.
- Dev.to’s Slack group.
-
Local Coding Meetups:
- Check platforms like Meetup.com or join local developer groups to connect with coders in your area.
- Benefits:
- Attend workshops, hackathons, and talks to enhance your skills.
- Build personal connections that can lead to mentorship or job referrals.
SEO and Building Authority for Your Programming Blog
If you’re documenting your coding journey or teaching others, having a programming blog can be incredibly impactful. To ensure it reaches the right audience, you need to optimize it using SEO principles while establishing your authority and trustworthiness.
Incorporating EEAT Principles into Your Blog:
-
Expertise:
- Share detailed, hands-on experiences from your coding journey.
- Post tutorials, code snippets, or case studies to demonstrate your understanding of topics.
- Example: Write a step-by-step guide on building a to-do app using Python and JavaScript.
-
Authoritativeness:
- Cite reliable sources, such as official documentation (e.g., Python.org, MDN Web Docs) or reputable programming blogs (e.g., Smashing Magazine, CSS-Tricks).
- Collaborate with or feature guest posts from experienced developers to lend additional credibility to your content.
-
Trustworthiness:
- Be transparent about your level of expertise. If you’re a beginner, share your learning curve and challenges honestly.
- Use HTTPS for your website to ensure it’s secure.
- Include a detailed About Me page outlining your background, goals, and mission.
Tips for SEO Optimization:
-
Use Relevant Keywords:
- Incorporate keywords like "coding for beginners," "programming basics," or "learn to code guide" naturally in your content.
- Example: "This guide on coding for beginners will walk you through the programming basics step by step."
-
Create High-Quality Content:
- Write in-depth posts that answer questions thoroughly. Avoid shallow content that only scratches the surface.
- Example: Instead of a generic overview of Python, write a comprehensive guide on Python for Data Analysis.
-
Optimize On-Page SEO:
- Use headers (H1, H2, H3) effectively to organize your content.
- Include meta descriptions, alt text for images, and internal links to other relevant blog posts.
-
Engage with Your Audience:
- Encourage readers to leave comments, ask questions, and share your posts.
- Respond promptly to comments to build trust and engagement.
-
Leverage Social Proof:
- Showcase testimonials from readers or community members who’ve benefited from your content.
- Highlight mentions from other blogs or platforms to build credibility.
Why Prateeksha Web Design is Your Ideal Partner
For aspiring bloggers or businesses aiming to document their coding journey, Prateeksha Web Design offers custom website solutions that are not only visually stunning but also SEO-friendly. Our expertise ensures that your blog ranks high on search engines and is optimized to deliver a seamless user experience. Let us help you build a platform that positions you as a trusted authority in the programming world.
Why Choose Prateeksha Web Design for Small Business Websites?
At Prateeksha Web Design, we specialize in creating custom, responsive, and SEO-friendly websites. Whether you’re launching a programming tutorial site or an e-commerce platform, our expertise ensures a polished, high-performing website. We combine technical expertise with creative design to help businesses stand out online.
Conclusion
Starting your programming journey is an exciting and rewarding endeavor. By focusing on programming basics, practicing consistently, and leveraging modern tools, you’ll soon be writing code like a pro. With support from communities and services like Prateeksha Web Design, you can build a successful online presence or even inspire others with your coding journey.
About Prateeksha Web Design
Prateeksha Web Design offers a comprehensive Learn To Code service for beginners looking to start their programming journey. Our experienced instructors provide step-by-step guidance and hands-on practice to help you understand programming concepts and languages. We cover a wide range of topics including HTML, CSS, JavaScript, and more. Our interactive and engaging lessons make learning to code fun and easy. Join us today to kickstart your programming career!
Interested in learning more? Contact us today.
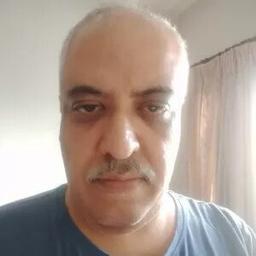