How to Prevent Screenshots in React Native Apps: A Complete Guide
In the world of mobile app development, security is a top priority. Whether you’re building a banking app, a confidential document viewer, or just want to keep your app’s content private, screenshot prevention is an essential feature. React Native, the go-to framework for cross-platform mobile app development, offers tools and techniques to address this concern. In this blog, we’ll explore how to prevent screenshots in React Native apps while maintaining a casual, engaging tone that makes security fun to learn. Oh, and don’t forget—we’ll be highlighting how Prateeksha Web Design can help you create secure, user-friendly mobile apps.
Why Prevent Screenshots in React Native Apps?
Before diving into the technical implementation of screenshot prevention in React Native, let’s explore why this feature is vital. Understanding the "why" lays the foundation for appreciating the need for this functionality and making informed decisions about integrating it into your app.
1. Protecting Sensitive Data
In today’s digital age, mobile apps often handle an immense amount of sensitive and confidential user data. Apps in industries like finance, healthcare, and communications are particularly vulnerable.
-
Banking Apps: These apps contain sensitive financial information, including account numbers, transaction details, and even passwords. Allowing users to take screenshots could inadvertently expose this data to malicious actors.
-
Healthcare Apps: Apps that store medical records, lab reports, or doctor consultations are bound by strict privacy regulations like HIPAA. If sensitive health information is screenshotted and shared, it could lead to legal and ethical complications.
-
Messaging Apps: Applications like WhatsApp, Signal, or Telegram often deal with personal and private conversations. Screenshots can compromise the privacy of these conversations if shared without consent.
Why it matters:
Screenshots provide a loophole for data breaches. Even if your app encrypts stored data or uses secure channels for transmission, a screenshot can capture sensitive information and share it outside your app’s secure environment. Preventing screenshots helps mitigate this risk.
2. Maintaining Intellectual Property
Beyond sensitive data, many apps are built on unique, valuable content that forms the core of their business. For instance:
-
E-learning Platforms: Online courses, exclusive tutorials, and proprietary study materials are often accessed through e-learning apps. Screenshots could allow users to duplicate this content without proper authorization, bypassing subscription fees or copyright protections.
-
Entertainment Apps: Apps offering premium videos, images, or music, such as subscription-based streaming platforms, can suffer from piracy if users are allowed to take screenshots and distribute content freely.
-
Design and Creative Tools: Apps that involve creative outputs—like graphic design, architecture tools, or photo editing apps—often feature proprietary templates and designs. Allowing screenshots could result in theft of intellectual property.
Why it matters:
Your content is your brand’s identity. Screenshots can undermine your efforts to protect exclusive or subscription-based content, leading to revenue loss and legal issues. Screenshot prevention reinforces your app’s commitment to protecting intellectual property.
3. Enhancing User Trust
User trust is one of the most valuable assets for any app. Screenshot prevention can play a significant role in building and maintaining this trust.
-
Privacy Expectations: Users expect apps handling their personal information to prioritize their privacy. Seeing measures like screenshot prevention sends a clear message: “We care about protecting your data.”
-
Sensitive Features: For apps with ephemeral content (like Snapchat) or secure data-sharing features, preventing screenshots is essential for delivering the experience users expect. Without such features, users might question the app’s reliability and commitment to security.
Why it matters:
User trust leads to loyalty. By taking proactive steps like preventing screenshots, you demonstrate that your app puts users’ privacy and security first, which can result in higher retention rates and positive reviews.
Challenges of Screenshot Prevention in React Native
While the reasons to prevent screenshots are compelling, implementing this feature comes with its own set of challenges. Let’s break them down.
1. Platform-Specific Features
Android and iOS, the two dominant mobile platforms, handle screenshots differently:
-
Android:
Android provides a built-in mechanism for screenshot prevention using theFLAG_SECURE
window flag. This flag blocks screenshots and screen recordings at the operating system level. It’s relatively straightforward to implement, but it has limitations, such as being restricted to the app’s current screen. -
iOS:
Apple’s iOS does not provide a direct API to block screenshots. Developers must rely on creative workarounds, such as detecting screenshots via system notifications or overlaying sensitive content with blurred views when the app goes into the background. These solutions are less foolproof and require constant updates as iOS evolves.
Why it’s challenging:
React Native is a cross-platform framework, meaning it aims to abstract platform-specific implementations into a single codebase. However, when it comes to native-level functionality like screenshot prevention, developers often need to dive into platform-specific code for both Android and iOS, complicating the development process.
2. User Experience Balance
Security is important, but it shouldn’t come at the expense of a seamless user experience.
-
Frustrating Restrictions: Users may feel restricted if they can’t take screenshots, especially for legitimate reasons like saving an important transaction ID or sharing content with support teams.
-
False Positives: Aggressive implementation might lead to unintended consequences, such as blocking screenshots in non-sensitive parts of the app where it’s unnecessary.
Why it’s challenging:
Balancing security and usability is a tightrope walk. An overly restrictive approach can frustrate users, while a lax approach may compromise security. Striking this balance requires thoughtful planning and user feedback.
3. React Native’s Cross-Platform Nature
React Native’s biggest advantage is its ability to use a single codebase for both Android and iOS apps. However, when dealing with native features like screenshot prevention, developers often encounter limitations:
-
Native Modules: To implement screenshot prevention, you may need to create or use native modules for Android and iOS separately, which adds complexity and increases development time.
-
Consistency Issues: Even with native modules, achieving a consistent experience across platforms can be challenging due to differences in how Android and iOS handle screenshots.
Why it’s challenging:
React Native simplifies cross-platform development but doesn’t eliminate the need for platform-specific solutions. Developers must often work around React Native’s abstractions to implement features like screenshot prevention effectively.
How to Prevent Screenshots in React Native
Screenshot prevention is a critical feature for many React Native apps, especially when handling sensitive or proprietary information. Both Android and iOS platforms have unique ways of implementing this feature. Below, we’ll break down the methods for preventing screenshots on each platform, ensuring your app is secure while maintaining a seamless user experience.
1. Prevent Screenshots on Android
Android provides a built-in mechanism for disabling screenshots using the FLAG_SECURE
window flag. This makes it relatively straightforward to implement screenshot prevention. There are two primary ways to enable this feature in React Native: using a library or directly modifying native code.
Using a Library: react-native-screenshot-prevention
The react-native-screenshot-prevention library simplifies the process of enabling screenshot prevention in your React Native app. Here’s how you can use it:
Steps to Implement:
-
Install the Library
Open your terminal and run the following command to install the library:
npm install react-native-screenshot-prevention
-
Link the Library
For React Native 0.60 and above, auto-linking is supported. For older versions, use:
react-native link react-native-screenshot-prevention
-
Use the Library in Your App
Import and activate screenshot prevention using the provided hook:
import { usePreventScreenCapture } from 'react-native-screenshot-prevention'; const App = () => { usePreventScreenCapture(true); // Enable screenshot prevention return ( <View> <Text>Screenshot prevention is enabled!</Text> </View> ); }; export default App;
This approach is simple and requires minimal configuration, making it an excellent choice for React Native developers.
Direct Native Code Implementation
If you prefer more control or don’t want to rely on a library, you can implement screenshot prevention by modifying the native Android code.
Steps to Implement:
-
Open the MainActivity.java File
Navigate to your Android project’s main activity file, typically located at:
android/app/src/main/java/com/yourprojectname/MainActivity.java
-
Add the FLAG_SECURE Flag
Inside the
onCreate
method, add the following line:@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); getWindow().setFlags(WindowManager.LayoutParams.FLAG_SECURE, WindowManager.LayoutParams.FLAG_SECURE); }
This modification prevents users from taking screenshots or screen recordings while the app is in use.
2. Prevent Screenshots on iOS
Unlike Android, iOS does not natively support blocking screenshots. However, there are effective workarounds to address this limitation, such as detecting screenshots or obscuring sensitive content.
Detecting Screenshots
iOS provides a system notification, UIApplicationUserDidTakeScreenshotNotification
, which triggers when a screenshot is taken. You can use this notification to detect screenshots and take appropriate action.
Steps to Implement:
-
Modify AppDelegate.m
Open the
AppDelegate.m
file in your iOS project and add the following code:[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(didTakeScreenshot) name:UIApplicationUserDidTakeScreenshotNotification object:nil]; - (void)didTakeScreenshot { NSLog(@"Screenshot detected!"); // Add your custom logic here, e.g., show an alert or log the event. }
-
Respond to Screenshot Events
You can customize the
didTakeScreenshot
method to respond to screenshot events. For example:- Display an alert to the user.
- Log the event for security purposes.
- Clear sensitive information from the screen.
Covering Sensitive Content
Another way to handle screenshot prevention on iOS is by covering sensitive content when the app goes into the background or loses focus. This doesn’t block screenshots but ensures sensitive data isn’t visible.
Example Implementation:
-
Create a Blur Overlay
Use the React Native
AppState
API to detect when the app transitions to the background and overlay sensitive content with a blurred or semi-transparent view.import React, { useEffect } from 'react'; import { AppState, View, StyleSheet } from 'react-native'; const BlurOverlay = () => { const handleAppStateChange = (nextAppState) => { if (nextAppState === 'background') { // Add blur overlay logic here } else if (nextAppState === 'active') { // Remove blur overlay logic } }; useEffect(() => { const subscription = AppState.addEventListener( 'change', handleAppStateChange ); return () => { subscription.remove(); }; }, []); return <View style={styles.overlay}></View>; }; const styles = StyleSheet.create({ overlay: { ...StyleSheet.absoluteFillObject, backgroundColor: 'rgba(0,0,0,0.5)', // Semi-transparent overlay }, }); export default BlurOverlay;
-
Integrate Into Your App
Add the
BlurOverlay
component to your app’s root component to ensure sensitive content is always protected when the app transitions to the background.
3. Third-Party Tools for Enhanced Security
If you’re looking for robust and comprehensive solutions, several third-party tools provide advanced security features, including screenshot prevention:
-
AppDome: AppDome offers a no-code platform to integrate security features like screenshot prevention into React Native apps. It’s ideal for enterprise-grade applications.
-
MobileIron: MobileIron provides mobile app security solutions, including advanced content protection and anti-screenshot capabilities.
Why Choose Third-Party Tools? Third-party tools often come with additional benefits like encryption, malware protection, and secure data storage. They’re a great choice if you’re building an app that requires high levels of security.
Best Practices for React Native Security
When developing mobile apps with React Native, security should always be a top priority. While preventing screenshots is one way to protect sensitive information, there are several other practices you can adopt to ensure your app is secure. Below, we’ll dive deeper into these best practices and explain why they are essential for safeguarding your app and its users.
1. Encrypt Sensitive Data
Encryption ensures that sensitive information is stored securely on the user’s device. Without encryption, if an attacker gains access to the device or the app’s local storage, they could easily extract unprotected data.
How to Implement Encryption in React Native
-
Use libraries like
react-native-encrypted-storage
to encrypt data at rest:npm install react-native-encrypted-storage
-
Example usage:
import EncryptedStorage from 'react-native-encrypted-storage'; // Save data securely const storeData = async () => { try { await EncryptedStorage.setItem( 'user_session', JSON.stringify({ token: 'secure_token', userId: '12345', }) ); console.log('Data securely saved.'); } catch (error) { console.error('Failed to save data:', error); } }; // Retrieve data const getData = async () => { try { const session = await EncryptedStorage.getItem('user_session'); if (session) { console.log('Retrieved data:', JSON.parse(session)); } } catch (error) { console.error('Failed to retrieve data:', error); } };
-
Why it’s important: Encryption prevents data from being read in plain text, even if the storage is compromised.
2. Use HTTPS for Secure Communication
All communication between the app and the server should be encrypted to prevent data interception or tampering. HTTPS (Hypertext Transfer Protocol Secure) ensures that the data transmitted is encrypted using protocols like TLS (Transport Layer Security).
Steps to Ensure HTTPS in React Native Apps
-
Use HTTPS URLs
Make sure all API endpoints use HTTPS instead of HTTP. -
Enable SSL Pinning
SSL pinning verifies the server's identity by comparing its SSL certificate with a trusted copy embedded in the app. Use libraries likereact-native-ssl-pinning
:npm install react-native-ssl-pinning
-
Validate Certificates
Reject any requests with invalid certificates. For example:fetch('https://secure-api.example.com', { method: 'GET', headers: { 'Content-Type': 'application/json', }, }).catch((error) => { console.error('Connection is not secure:', error); });
- Why it’s important: HTTPS ensures that sensitive information like login credentials, personal data, and financial transactions are transmitted securely, protecting users from man-in-the-middle (MITM) attacks.
3. Implement Two-Factor Authentication (2FA)
Two-Factor Authentication (2FA) adds an extra layer of security to user accounts by requiring users to verify their identity through a secondary method, such as a code sent to their phone or email.
How to Add 2FA in React Native
-
Use an authentication service like Firebase Authentication or Auth0, which supports 2FA out of the box.
-
Example with Firebase:
npm install @react-native-firebase/auth
Enable phone authentication in the Firebase console, and then:
import auth from '@react-native-firebase/auth'; const sendOtp = async (phoneNumber) => { try { const confirmation = await auth().signInWithPhoneNumber(phoneNumber); console.log('OTP sent!'); // Save the confirmation object for verification } catch (error) { console.error('Failed to send OTP:', error); } }; const verifyOtp = async (confirmation, otpCode) => { try { await confirmation.confirm(otpCode); console.log('OTP verified, user authenticated!'); } catch (error) { console.error('Invalid OTP:', error); } };
-
Why it’s important: 2FA reduces the risk of unauthorized access, even if a user’s password is compromised.
4. Monitor Permissions
React Native apps often request permissions to access device features like location, camera, or storage. However, requesting excessive permissions can compromise user privacy and security.
Best Practices for Managing Permissions
-
Use the
react-native-permissions
library to handle permissions effectively:npm install react-native-permissions
-
Example of requesting permissions:
import { check, request, PERMISSIONS, RESULTS, } from 'react-native-permissions'; const requestPermission = async () => { const result = await request(PERMISSIONS.ANDROID.CAMERA); if (result === RESULTS.GRANTED) { console.log('Camera permission granted!'); } else { console.log('Camera permission denied!'); } };
-
Avoid requesting unnecessary permissions. For example, if your app doesn’t require GPS, don’t request location access.
-
Why it’s important: Excessive permissions can make your app vulnerable to misuse or raise red flags with users and app store reviewers.
5. Regularly Update Dependencies
Keeping your app and its dependencies up to date is crucial for maintaining security. Outdated libraries may have vulnerabilities that attackers can exploit.
How to Stay Updated
-
Audit Your Dependencies Regularly Use tools like npm audit to identify vulnerabilities:
npm audit
-
Update Packages Run the following commands to update your dependencies:
npm outdated npm update
-
Monitor Security Advisories Check the npm security advisories and React Native release notes to stay informed about new updates and patches.
-
Test After Updates After updating dependencies, thoroughly test your app to ensure compatibility and functionality.
- Why it’s important: Regular updates patch known vulnerabilities and improve performance, keeping your app secure and stable.
How Prateeksha Web Design Can Help
At Prateeksha Web Design, we understand that security is non-negotiable in today’s app ecosystem. Here’s how we can help:
- Custom React Native Modules: We build tailored solutions for advanced security features like screenshot prevention.
- Comprehensive Security Audits: Our team conducts detailed audits to identify and fix vulnerabilities in your app.
- Cross-Platform Expertise: With extensive experience in both Android and iOS, we ensure your app is secure across all devices.
- User-Centric Design: We strike the perfect balance between security and user experience, ensuring your app feels safe yet seamless.
Conclusion
Preventing screenshots in React Native apps is a crucial step in safeguarding sensitive data, intellectual property, and user trust. While challenges exist, solutions like the react-native-screenshot-prevention
library, native code modifications, and creative workarounds make it achievable. Combined with best practices for mobile app security, these techniques will elevate your app’s security game.
And remember, if you’re looking for a partner to build secure, user-friendly apps, Prateeksha Web Design has your back. With our expertise in React Native security and mobile app development, we’ll help you create an app that’s not just functional but also secure.
About Prateeksha Web Design
Prateeksha Web Design offers specialized services for preventing screenshots in React Native applications, enhancing user privacy and content protection. Their approach includes implementing native code solutions and leveraging React Native libraries to detect and block screenshot attempts. With a focus on user experience, they ensure seamless integration of security features without compromising app performance. The team also provides comprehensive testing and support to address potential vulnerabilities. Choose Prateeksha for robust, innovative strategies to safeguard your app's sensitive information.
Interested in learning more? Contact us today.
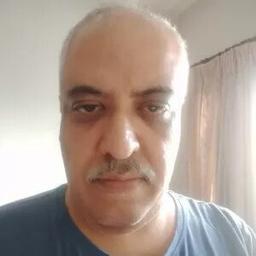