Python has gained massive popularity for its simplicity and flexibility, making it the go-to language for many developers and data scientists. Yet, with great flexibility comes great responsibility—especially when it comes to data validation. Whether you're dealing with APIs, database inputs, or user forms, ensuring the accuracy of your data is non-negotiable.
Enter Pydantic, a powerful library that takes the hassle out of data validation in Python. It's like having a personal assistant for your data, ensuring everything is tidy, consistent, and ready to go. In this guide, we’ll explore how to use Pydantic for data validation while highlighting Python best practices to make your code clean, efficient, and foolproof.
If you're just starting out or you're a seasoned developer looking to level up, this guide is for you. By the end, you'll not only master data validation but also understand why Pydantic is a game-changer for Python developers.
What is Pydantic?
Pydantic is an open-source Python library designed to make data validation and data parsing easier and more efficient. It uses Python type annotations as its foundation, allowing developers to define models that enforce type constraints and validate data effortlessly. In essence, Pydantic ensures that your data is both structured and correct before you process or manipulate it.
Instead of manually writing repetitive and error-prone validation logic, Pydantic automates the process, freeing you to focus on your application's functionality. It’s widely used in modern Python projects, particularly in API development (with frameworks like FastAPI) and applications where data consistency is critical.
How Does Pydantic Work?
Pydantic’s magic lies in its ability to interpret Python’s type annotations. When you define a Pydantic model, it validates incoming data against the specified types and constraints. If the data doesn't meet the criteria, Pydantic raises detailed, user-friendly errors, making debugging a breeze.
Here’s a simple example:
from pydantic import BaseModel
class User(BaseModel):
name: str
age: int
email: str
# Correct data
user = User(name="Alice", age=25, email="alice@example.com")
print(user)
# Incorrect data
invalid_user = User(name="Bob", age="not_a_number", email="bob@example.com")
When the invalid data is processed, Pydantic identifies the issue (age
is not an integer) and raises a ValidationError
with clear details.
Key Features of Pydantic
-
Type Enforcement
- Pydantic ensures that data matches the types specified in your model.
- For example, if you declare a field as an
int
, any non-integer input will trigger a validation error. - This eliminates many bugs that stem from incorrect data types.
class Product(BaseModel): name: str price: float product = Product(name="Gadget", price="not_a_float") # Raises ValidationError
-
Validation Rules
- Pydantic allows you to enforce additional constraints through validators. These custom rules help handle complex validation scenarios effortlessly.
- Example: Ensuring a user’s age is above a certain value.
from pydantic import BaseModel, validator class User(BaseModel): name: str age: int @validator('age') def check_age(cls, value): if value < 18: raise ValueError("Age must be at least 18.") return value
-
Parsing
- Pydantic can automatically parse complex data (e.g., JSON) into Python objects while ensuring type safety.
- This is especially useful when dealing with APIs or external data sources.
json_data = '{"name": "Alice", "age": 25, "email": "alice@example.com"}' user = User.parse_raw(json_data) print(user.name) # Output: Alice
-
Error Handling
- Pydantic provides detailed, user-friendly error messages when validation fails, making it easier to debug and fix issues.
Example of an error message:
pydantic.error_wrappers.ValidationError: 1 validation error for User age value is not a valid integer (type=type_error.integer)
-
Serialization
- Pydantic can serialize Python objects into other formats like JSON.
- This feature is invaluable when creating APIs or saving data to external systems.
user = User(name="Alice", age=25, email="alice@example.com") print(user.json()) # Converts the object to JSON
Why Use Pydantic for Data Validation?
Pydantic simplifies and enhances data validation by automating many repetitive and error-prone tasks. Let’s break down its advantages:
-
Efficiency
- Without Pydantic, you’d need to write custom logic to validate each field, handle errors, and ensure type safety. This is not only tedious but prone to bugs.
- Pydantic automates these tasks, making your codebase cleaner and easier to maintain.
-
Readability
- Models defined using Pydantic are easy to understand, even for developers unfamiliar with the project.
- A well-defined Pydantic model serves as documentation, showing exactly what data is expected and how it should be structured.
-
Integration with FastAPI
- FastAPI, one of the most popular Python frameworks for API development, uses Pydantic for request validation and response serialization.
- This tight integration means less boilerplate code and more time focusing on functionality.
from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str price: float @app.post("/items/") async def create_item(item: Item): return {"name": item.name, "price": item.price}
-
Pythonic Design
- Pydantic takes advantage of Python’s type hinting system, making it intuitive for Python developers.
- You don’t need to learn a new syntax—just extend Python’s existing capabilities with additional validation features.
Real-World Applications
1. API Development
- Validate incoming requests and responses in web applications.
- Ensure data integrity between the client and server.
2. Data Transformation
- Parse and convert raw data (e.g., from APIs or files) into Python objects while ensuring consistency.
3. Configuration Management
-
Validate and load application configuration files (e.g.,
.env
files) with type safety.from pydantic import BaseSettings class Config(BaseSettings): app_name: str debug_mode: bool config = Config(app_name="MyApp", debug_mode="True") # Automatically converts "True" to a boolean
Why We Recommend Pydantic at Prateeksha Web Design
At Prateeksha Web Design, we prioritize clean, maintainable, and error-free code. Pydantic aligns perfectly with these goals:
- Reliability: Data validation ensures that errors are caught early, reducing bugs in production.
- Scalability: Pydantic models make it easy to extend or modify data structures as your application grows.
- Speed: Automating validation allows us to deliver projects faster without compromising quality.
Whether we're building APIs for e-commerce platforms or integrating third-party tools, Pydantic enables us to create robust, user-friendly solutions for our clients.
Getting Started with Pydantic
Before diving into validation, let’s set up the basics.
Installation
Install Pydantic using pip:
pip install pydantic
A Simple Example
Here’s a quick demo to showcase Pydantic's magic:
from pydantic import BaseModel
class User(BaseModel):
name: str
age: int
email: str
# Valid data
user_data = {"name": "Alice", "age": 25, "email": "alice@example.com"}
user = User(**user_data)
print(user)
# Invalid data
invalid_data = {"name": "Bob", "age": "twenty-five", "email": "bob@example.com"}
user = User(**invalid_data)
Output for invalid data:
pydantic.error_wrappers.ValidationError: 1 validation error for User
age
value is not a valid integer (type=type_error.integer)
Deep Dive: Validating Data with Pydantic
Now that you’ve seen the basics, let’s explore Pydantic’s robust validation capabilities.
1. Default Values
You can set default values for fields, ensuring your models work even when some data is missing.
class Product(BaseModel):
name: str
price: float = 9.99 # Default price
in_stock: bool = True
product = Product(name="Gadget")
print(product)
2. Custom Validation
Use @validator
decorators to add custom validation logic.
from pydantic import BaseModel, validator
class User(BaseModel):
name: str
age: int
@validator("age")
def check_age(cls, value):
if value < 18:
raise ValueError("Age must be at least 18.")
return value
user = User(name="John", age=20) # Valid
user = User(name="Doe", age=15) # Raises ValidationError
3. Nested Models
Pydantic allows nested data validation with ease.
class Address(BaseModel):
street: str
city: str
class User(BaseModel):
name: str
address: Address
user_data = {
"name": "Alice",
"address": {"street": "123 Main St", "city": "Wonderland"}
}
user = User(**user_data)
print(user)
4. List and Dict Validation
Pydantic handles lists and dictionaries seamlessly.
class ShoppingCart(BaseModel):
items: list[str]
quantities: dict[str, int]
cart = ShoppingCart(items=["apple", "banana"], quantities={"apple": 2, "banana": 3})
print(cart)
5. Enums and Constraints
Restrict values with Enums and enforce constraints.
from pydantic import BaseModel, conint
from enum import Enum
class Color(Enum):
RED = "red"
GREEN = "green"
BLUE = "blue"
class Product(BaseModel):
name: str
quantity: conint(gt=0) # Quantity must be greater than 0
color: Color
product = Product(name="T-Shirt", quantity=10, color=Color.RED)
Handling Errors Gracefully
Error handling is a breeze with Pydantic. Validation errors are raised as ValidationError
, making debugging straightforward.
from pydantic import ValidationError
try:
User(name="Alice", age="not_a_number")
except ValidationError as e:
print(e.json())
Advanced Pydantic Features
1. Configuring Models
You can tweak Pydantic's behavior with Config
.
class User(BaseModel):
name: str
age: int
class Config:
allow_population_by_field_name = True
validate_assignment = True
2. Data Transformation
Pydantic supports data transformation with alias
.
class User(BaseModel):
first_name: str
last_name: str
class Config:
allow_population_by_field_name = True
fields = {"first_name": "firstName", "last_name": "lastName"}
data = {"firstName": "Alice", "lastName": "Smith"}
user = User(**data)
3. Using Pydantic with FastAPI
FastAPI and Pydantic are a match made in heaven. They make API development faster, more reliable, and developer-friendly.
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
price: float
@app.post("/items/")
async def create_item(item: Item):
return {"name": item.name, "price": item.price}
Python Best Practices for Data Validation with Pydantic
Pydantic is a fantastic tool, but like any technology, using it effectively requires following best practices. Here’s a detailed breakdown of how to get the most out of Pydantic while adhering to Python best practices.
1. Use Type Annotations
Why it matters:
- Type annotations are the foundation of Pydantic. They tell the library what types of data to expect and validate.
- Using annotations ensures clarity and prevents errors by catching mismatches early in the development process.
Best practices:
- Always specify types for every field in your models.
- Use precise types (e.g.,
List[str]
instead of justlist
) to enhance validation.
Example:
from pydantic import BaseModel
from typing import List, Optional
class Product(BaseModel):
name: str
price: float
tags: Optional[List[str]] # Optional field that can be a list of strings or None
2. Keep Models Simple
Why it matters:
- Overcomplicating your models with excessive validation logic or nested fields can make them hard to maintain and debug.
- A clean model is easier for collaborators (or your future self) to understand and work with.
Best practices:
- Focus on defining fields and their types in the model.
- Offload complex logic to dedicated utility functions or services outside the model.
Example:
# Good: Simple and clean
class User(BaseModel):
name: str
age: int
# Bad: Too much logic inside the model
class User(BaseModel):
name: str
age: int
def is_adult(self):
return self.age >= 18
Instead of embedding logic like is_adult
in the model, handle it elsewhere in the application.
3. Leverage Validators
Why it matters:
- Validators allow you to define custom validation rules for specific fields or the entire model.
- They make it easy to enforce business logic without cluttering the rest of your code.
Best practices:
- Use
@validator
for field-specific rules. - Use
@root_validator
when validation depends on multiple fields. - Keep validators short and focused on a single purpose.
Example of field-specific validator:
from pydantic import BaseModel, validator
class User(BaseModel):
name: str
age: int
@validator('age')
def validate_age(cls, value):
if value < 18:
raise ValueError("Age must be at least 18.")
return value
Example of root validator:
from pydantic import BaseModel, root_validator
class Order(BaseModel):
item: str
quantity: int
price_per_unit: float
@root_validator
def check_total_price(cls, values):
if values['quantity'] * values['price_per_unit'] > 1000:
raise ValueError("Total price cannot exceed 1000.")
return values
4. Document Your Models
Why it matters:
- Clear documentation helps other developers (and you) understand the purpose and requirements of each model.
- It reduces onboarding time for team members and minimizes errors.
Best practices:
- Use Python docstrings to explain what each model and its fields represent.
- Include examples of valid data when possible.
Example:
class User(BaseModel):
"""
Represents a user in the system.
Fields:
- name: Full name of the user.
- email: Email address of the user. Must be a valid email format.
- age: Age of the user. Must be at least 18.
"""
name: str
email: str
age: int
5. Test Your Models
Why it matters:
- Writing tests ensures your validation logic works as intended and helps catch edge cases.
- Automated tests provide confidence that changes to the model won’t introduce regressions.
Best practices:
- Write unit tests for all validators.
- Test both valid and invalid data scenarios.
- Use pytest or another testing framework for a clean and maintainable test suite.
Example using pytest:
import pytest
from pydantic import BaseModel, ValidationError
class User(BaseModel):
name: str
age: int
@validator('age')
def validate_age(cls, value):
if value < 18:
raise ValueError("Age must be at least 18.")
return value
def test_valid_user():
user = User(name="Alice", age=25)
assert user.name == "Alice"
assert user.age == 25
def test_invalid_user_age():
with pytest.raises(ValidationError):
User(name="Bob", age=15)
Why Prateeksha Web Design Chooses Pydantic
At Prateeksha Web Design, we’re committed to delivering robust and scalable applications for our clients. Pydantic helps us:
- Reduce Bugs: Strong validation catches errors early.
- Improve Code Quality: Cleaner, more maintainable code leads to faster development cycles.
- Enhance User Experience: Validated data ensures smooth operations for end-users.
Conclusion
Data validation might not sound glamorous, but it's the backbone of reliable software development. Pydantic takes the pain out of data validation, allowing developers to focus on building features rather than debugging data issues. Its integration with modern Python tools like FastAPI makes it a must-have for any developer.
If you're building Python projects and care about Python best practices, Pydantic should be at the top of your toolkit. And if you're looking to take your projects to the next level, Prateeksha Web Design is here to help you deliver clean, efficient, and scalable solutions.
About Prateeksha Web Design
Prateeksha Web Design offers expert services in implementing data validation using Pydantic in Python. We specialize in crafting robust data models that ensure data integrity and type safety. Our team builds user-friendly interfaces that seamlessly integrate Pydantic validations into web applications. We provide comprehensive documentation and support for developers to optimize their workflow. Enhance your projects with our tailored solutions for efficient data handling and validation.
Interested in learning more? Contact us today.
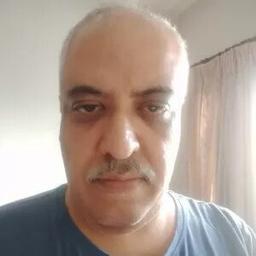