So, you’ve built a React Native app, and everything looks great—until it starts slowing down like a turtle on a lazy Sunday. 🐢 No worries! You’re not alone. React Native performance issues can sneak up on the best of us, but with the right optimizations, your app can run smoother than a fresh jar of peanut butter. 🥜
In this guide, we’ll break down the best strategies to turbocharge your React Native app in 2025—from optimizing rendering to cutting down load times. And if you’re searching for a best web design company in Mumbai to help with your app or develop a website in Mumbai, we’ll sprinkle in a few recommendations along the way. Let’s dive in!
🚀 1. Minimize Re-Renders with Pure Components & Memoization
🔥 Why It Matters:
When building a React Native app, one of the most common performance issues developers face is unnecessary re-renders. Every time your component receives new props or the state updates, React re-renders the entire component tree. While this is part of how React works, excessive re-renders can slow down your app—especially if you have nested components or a large number of UI elements.
Imagine you’re running a food delivery app, and every time a new order comes in, the entire list of orders refreshes, even for orders that haven’t changed. That’s a waste of resources, and it leads to a sluggish user experience.
By optimizing rendering, you can reduce unnecessary UI updates, making your app faster and more responsive.
💡 The Fix:
To tackle unnecessary re-renders, we can use:
- React.memo for function components
- PureComponent for class components
1️⃣ Using React.memo to Optimize Functional Components
If your component only relies on props and doesn’t manage internal state, you can wrap it in React.memo
. This tells React to only re-render the component when its props change—not on every parent component update.
🔹 Example: Before Optimization (Component re-renders unnecessarily)
import React from 'react';
import { Text } from 'react-native';
const UserGreeting = ({ name }) => {
console.log('Rendering...');
return <Text>Hello, {name}!</Text>;
};
export default UserGreeting;
📌 Problem: Every time the parent re-renders, this component re-renders—even if name
hasn’t changed.
✅ Optimized Version Using React.memo:
import React, { memo } from 'react';
import { Text } from 'react-native';
const UserGreeting = memo(({ name }) => {
console.log('Rendering...');
return <Text>Hello, {name}!</Text>;
});
export default UserGreeting;
📌 Why This Works:
React.memo()
compares the new props with the previous ones. If there’s no change, React skips rendering.- Now, if the parent component updates but
name
remains the same,UserGreeting
won’t re-render!
2️⃣ Using PureComponent for Class Components
For class-based components, use PureComponent
instead of Component
.
🔹 Example: Before Optimization (Re-renders unnecessarily)
import React, { Component } from 'react';
import { Text } from 'react-native';
class UserGreeting extends Component {
render() {
console.log('Rendering...');
return <Text>Hello, {this.props.name}!</Text>;
}
}
export default UserGreeting;
📌 Problem: Just like with functional components, this class component re-renders every time its parent updates, even if name
is unchanged.
✅ Optimized Version Using PureComponent:
import React, { PureComponent } from 'react';
import { Text } from 'react-native';
class UserGreeting extends PureComponent {
render() {
console.log('Rendering...');
return <Text>Hello, {this.props.name}!</Text>;
}
}
export default UserGreeting;
📌 Why This Works:
PureComponent
automatically implements a shallow comparison of props and state.- If the props haven’t changed, React skips rendering.
3️⃣ Avoid Inline Functions & Objects in Props
Even with React.memo
or PureComponent
, React might still re-render if you’re passing new objects or functions as props.
❌ Bad Example: Passing an Inline Function
<OptimizedComponent fetchData={() => fetch('https://api.example.com/data')} />
📌 Problem: Every render creates a new function, causing re-renders.
✅ Solution: Use useCallback
const fetchData = useCallback(() => fetch('https://api.example.com/data'), []);
<OptimizedComponent fetchData={fetchData} />
📌 Why This Works: useCallback
ensures that the function reference stays the same unless dependencies change.
⚡ 2. Optimize Navigation with React Navigation
🔥 Why It Matters:
Imagine you’re using an app, and every time you switch screens, there’s a noticeable lag—or worse, a flash of blank space before the new screen loads. That’s because React Navigation, if not optimized, can become a bottleneck, especially with complex navigation stacks.
A slow navigation experience feels frustrating to users and can hurt retention rates. In 2025, users expect buttery smooth navigation, and we can achieve that with the right techniques.
💡 The Fix:
- Use Native Stack Navigator
- Lazy-load screens to improve memory efficiency
1️⃣ Use Native Stack Navigator
By default, React Navigation uses JavaScript-based transitions, which can be slow. The Native Stack Navigator utilizes native platform navigation (like Swift for iOS & Kotlin for Android), making transitions much faster.
✅ Optimized Navigation Setup
import { createNativeStackNavigator } from '@react-navigation/native-stack';
const Stack = createNativeStackNavigator();
<Stack.Navigator screenOptions={{ animation: 'fade' }}>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Profile" component={ProfileScreen} />
</Stack.Navigator>;
📌 Why This Works:
- Uses native navigation APIs, making screen transitions much faster.
- Reduces JavaScript overhead, improving responsiveness.
- Supports gesture-based navigation (like swipe to go back).
2️⃣ Lazy-Load Screens for Faster Initial Load
When your app starts, React Navigation loads every screen in memory, which can slow down launch speed. Instead, load screens only when needed.
✅ How to Lazy-Load Screens
<Stack.Screen
name="Settings"
component={React.lazy(() => import('./SettingsScreen'))}
/>
📌 Why This Works:
- Reduces initial app load time.
- Loads screens only when the user navigates to them.
🎨 3. Use Hermes for a Speed Boost
🔥 Why It Matters:
JavaScript execution speed plays a crucial role in React Native performance, especially in complex apps that handle a lot of UI updates, animations, or data processing. By default, React Native runs on the JavaScriptCore (JSC) engine, which works fine but isn’t fully optimized for mobile devices—especially lower-end Android devices.
Enter Hermes, a lightweight JavaScript engine built specifically for React Native. It significantly improves startup times, reduces memory consumption, and enhances execution speed. If your app has noticeable lag or slow startup, enabling Hermes can be a game-changer.
💡 The Fix: Enable Hermes in Your React Native Project
Step 1: Enable Hermes in Your Build Configuration
Go to your android/app/build.gradle
file and enable Hermes by setting enableHermes: true
:
project.ext.react = [
enableHermes: true // Enable Hermes for better performance
]
Step 2: Reinstall Dependencies & Rebuild Your App
After enabling Hermes, reinstall dependencies and rebuild your app:
cd android && ./gradlew clean
cd ..
npm install
npx react-native run-android
Step 3: Verify Hermes is Enabled
To check if Hermes is running, use the following command:
console.log(HermesInternal ? "Hermes is enabled" : "Hermes is not enabled");
If Hermes is enabled, your app should now:
✅ Launch faster (reduced JavaScript initialization time)
✅ Use less memory (ideal for low-end devices)
✅ Execute JavaScript operations more efficiently
🖼️ 4. Optimize Images & Assets
🔥 Why It Matters:
Let’s be real—nobody likes waiting for images to load. 📷 But when you’re working with React Native, unoptimized images can drastically slow down your app.
Heavy images increase memory usage, take longer to load, and can make your app feel laggy, especially on older devices. If your app displays multiple images (e.g., e-commerce apps, social media feeds), failing to optimize them could kill your performance.
💡 The Fix: Optimize How You Handle Images
1️⃣ Use react-native-fast-image Instead of Image Component
React Native’s default <Image>
component is not optimized for performance. Instead, use react-native-fast-image, which is built for speed and supports:
✅ Caching (prevents unnecessary re-downloads)
✅ Faster loading (optimized for both iOS & Android)
✅ Better memory management
Installation:
npm install react-native-fast-image
Usage:
import FastImage from 'react-native-fast-image';
<FastImage
style={{ width: 100, height: 100 }}
source={{ uri: 'https://example.com/image.jpg' }}
resizeMode={FastImage.resizeMode.cover}
/>;
2️⃣ Compress Images Before Using Them
🔹 JPEG over PNG: Use JPEG (smaller size) instead of PNG unless transparency is needed.
🔹 Use an Image Optimization Tool: Services like TinyPNG, ImageOptim, or Squoosh can reduce file sizes significantly.
🔹 Use WebP Format: WebP images are smaller and load faster compared to PNG/JPEG.
3️⃣ Load Images Asynchronously
If your app displays lots of images at once, load them asynchronously instead of blocking UI updates.
const [imageLoaded, setImageLoaded] = useState(false);
return (
<View>
{!imageLoaded && <ActivityIndicator size="small" color="#0000ff" />}
<FastImage
style={{ width: 100, height: 100 }}
source={{ uri: 'https://example.com/image.jpg' }}
onLoad={() => setImageLoaded(true)}
/>
</View>
);
📌 Why This Works: The Activity Indicator ensures a smooth user experience while the image loads.
4️⃣ Use Lazy Loading for Offscreen Images
Instead of loading all images at once, lazy load them only when needed to save memory.
<FlatList
data={imageList}
keyExtractor={(item) => item.id.toString()}
renderItem={({ item }) => (
<FastImage
style={{ width: 100, height: 100 }}
source={{ uri: item.url }}
/>
)}
initialNumToRender={5} // Only load the first 5 images initially
removeClippedSubviews={true} // Unmount images not in view
/>
📌 Why This Works: FlatList renders only the visible images, making your app much faster.
🏎️ 5. Avoid Unnecessary State Updates
🔥 Why It Matters:
State management is the heartbeat of your React Native app. Every time the state changes, React triggers a re-render for that component (and potentially its children). If you’re not careful, unnecessary state updates can bog down performance, especially when they trigger multiple re-renders. Think of it like having to repaint a house every time someone knocks on the door—exhausting and unnecessary! 🏠
Imagine a scenario where you’re building a live chat feature. Every incoming message causes the entire chat history to re-render instead of just updating the new message. This can create lag and a poor user experience, especially on older devices.
💡 The Fix:
1️⃣ Keep Your State Local Where Possible If a piece of state is only relevant to a specific part of your component, keep it local to avoid unnecessary re-renders higher up in the component tree.
// Good: Local state for a specific component
const MessageInput = () => {
const [input, setInput] = useState('');
return <TextInput value={input} onChangeText={setInput} />;
};
2️⃣ Use useReducer Instead of useState for Complex Logic When dealing with complex state logic (multiple related state variables or state that depends on previous state), useReducer provides better control and optimization.
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
const Counter = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<>
<Text>{state.count}</Text>
<Button onPress={() => dispatch({ type: 'increment' })} title="+" />
<Button onPress={() => dispatch({ type: 'decrement' })} title="-" />
</>
);
};
3️⃣ Avoid Modifying State Directly Inside Loops Updating state inside loops can lead to unexpected behavior due to how React batches state updates. Instead, use a functional update pattern.
// Avoid this:
for (let i = 0; i < 10; i++) {
setCount(count + 1); // Will only update once
}
// Use this:
for (let i = 0; i < 10; i++) {
setCount(prevCount => prevCount + 1); // Correctly updates 10 times
}
By using prevCount
, each state update considers the latest state, ensuring accuracy and avoiding unnecessary re-renders.
⚙️ 6. Optimize List Performance with FlatList
🔥 Why It Matters:
Lists are a common element in apps—whether it's a list of products, messages, or posts. If your list has hundreds or thousands of items, using ScrollView can be disastrous. ScrollView loads all items at once, consuming lots of memory and leading to crashes or sluggish performance.
With FlatList, only the items currently on the screen are rendered, while the others are efficiently managed in the background, making even massive lists scroll smoothly.
💡 The Fix:
1️⃣ Use FlatList with keyExtractor
The keyExtractor
prop helps React identify which items have changed, are added, or are removed, making it crucial for performance.
<FlatList
data={data}
keyExtractor={(item) => item.id.toString()} // Ensure each item has a unique key
renderItem={({ item }) => <Text>{item.name}</Text>}
initialNumToRender={10} // Render only a few items initially
removeClippedSubviews={true} // Unmount items not in view
/>
2️⃣ Use getItemLayout for Fixed Height Items
If your list items are of fixed height, you can use getItemLayout
to optimize scroll performance. This allows FlatList to calculate the positions of items without rendering them.
<FlatList
data={data}
keyExtractor={(item) => item.id.toString()}
renderItem={({ item }) => <Text>{item.name}</Text>}
getItemLayout={(data, index) => (
{ length: ITEM_HEIGHT, offset: ITEM_HEIGHT * index, index }
)}
/>
This setup is perfect for chat apps or feeds where items are consistent in size.
3️⃣ Pagination and Lazy Loading Instead of loading all data at once, implement pagination or infinite scrolling.
const [page, setPage] = useState(1);
const fetchMore = () => {
setPage((prevPage) => prevPage + 1);
};
<FlatList
data={data}
renderItem={({ item }) => <Text>{item.name}</Text>}
onEndReached={fetchMore} // Fetch next set of items
onEndReachedThreshold={0.5} // Trigger fetch when halfway through the list
/>
📡 7. Use Background Threads for Heavy Operations
🔥 Why It Matters:
React Native runs JavaScript on a single thread, meaning if you’re performing heavy tasks (like image processing, data crunching, or complex animations), it blocks the UI thread, causing the app to stutter or freeze. To keep everything smooth, offload these tasks to background threads.
💡 The Fix:
1️⃣ Use react-native-reanimated for Animations This library runs animations directly on the native thread, bypassing JavaScript and reducing lag.
import Animated, { useAnimatedStyle, withSpring } from 'react-native-reanimated';
const animatedStyle = useAnimatedStyle(() => ({
transform: [{ translateY: withSpring(100) }], // Smooth animation
}));
2️⃣ Offload Heavy Tasks with Worker Threads For CPU-intensive tasks, consider using Worker Threads. This lets you run JavaScript code in parallel threads without blocking the main thread.
import { Worker } from 'react-native-workers';
const worker = new Worker('worker.js');
worker.postMessage({ data: heavyDataProcessing() });
worker.onmessage = (message) => {
console.log('Processed data:', message.data);
};
3️⃣ Consider Native Modules For extremely heavy tasks (like video encoding), write Native Modules in Swift, Kotlin, or Objective-C. This ensures the task runs natively, freeing up the JavaScript thread entirely.
💾 8. Reduce Bundle Size with Code Splitting
🔥 Why It Matters:
A large bundle size directly impacts app load time, memory usage, and overall performance. The bigger the JavaScript bundle, the longer it takes to parse and execute, leading to slow startup times—especially on lower-end devices.
For example, if you’re building a React Native e-commerce app, loading all checkout, user profile, and admin components upfront makes no sense if the user is just browsing products. Instead, we should dynamically load components only when needed, reducing the initial load burden.
💡 The Fix:
1️⃣ Use Dynamic Imports with React.lazy()
Instead of importing everything at once, dynamically load components only when they are needed.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const App = () => (
<Suspense fallback={<Text>Loading...</Text>}>
<LazyComponent />
</Suspense>
);
📌 Why This Works:
- The component isn’t loaded until needed, reducing initial bundle size.
Suspense
provides a fallback UI while the component loads asynchronously.
2️⃣ Remove Unused Dependencies with Bundle Analysis
Unused npm packages increase your bundle size. Use react-native-bundle-visualizer to identify and remove them.
npx react-native-bundle-visualizer
📌 How It Helps:
- Identifies large dependencies.
- Helps you remove unnecessary libraries.
- Keeps your app lean and efficient.
3️⃣ Optimize Third-Party Packages
Some third-party libraries are huge and unnecessary. Before using a package, ask:
✅ Is there a smaller alternative?
✅ Do I really need this dependency?
For example:
❌ moment.js (Large, ~250KB) → ✅ day.js (Small, ~2KB)
❌ lodash (Complete Library) → ✅ lodash-es (Tree-shakable)
By reducing bundle size, your app loads faster, feels more responsive, and performs better on low-end devices. 🚀
🎯 9. Optimize Network Requests
🔥 Why It Matters:
Network performance is critical in a mobile app. If your app makes too many requests, loads unnecessary data, or doesn't handle caching efficiently, it can:
🚨 Slow down your app
🚨 Increase data consumption
🚨 Drain battery life
💡 The Fix:
1️⃣ Use Axios for API Requests with Caching
Instead of fetching the same data repeatedly, cache API responses.
import axios from 'axios';
import LRU from 'lru-cache';
const cache = new LRU({ max: 50 });
const fetchData = async (url) => {
if (cache.has(url)) return cache.get(url);
const response = await axios.get(url);
cache.set(url, response.data);
return response.data;
};
📌 Why This Works:
- Prevents redundant API calls.
- Reduces server load and improves speed.
2️⃣ Optimize API Calls with GraphQL Instead of REST
REST APIs often return too much data, slowing down the app. Instead, GraphQL only fetches the required data.
import { gql, useQuery } from '@apollo/client';
const GET_USER = gql`
query {
user {
name
email
}
}
`;
const { data } = useQuery(GET_USER);
📌 Why This Works:
- Fetches only what’s needed, reducing payload size.
- Minimizes over-fetching, improving response times.
3️⃣ Compress JSON Responses
If your server returns huge JSON responses, compress them using Gzip or Brotli before sending them to the client.
const express = require('express');
const compression = require('compression');
const app = express();
app.use(compression()); // Enable Gzip compression
📌 Why This Works:
- Smaller data payloads = Faster API calls.
- Reduces network usage and improves response time.
By optimizing API calls, your app will load data faster, consume less bandwidth, and provide a smoother experience. 🚀
🔋 10. Use Native Modules for Performance-Intensive Tasks
🔥 Why It Matters:
React Native runs JavaScript on a single thread, meaning if your app performs heavy tasks like video processing, encryption, or file operations, it will block the UI, causing lag and crashes.
To fix this, we can offload intensive operations to native code, leveraging the power of Swift, Objective-C (iOS), Java, and Kotlin (Android).
💡 The Fix:
1️⃣ Use react-native-mmkv for Super-Fast Storage
AsyncStorage is slow for large data operations. Use react-native-mmkv, a fast, native-backed storage solution.
import { MMKV } from 'react-native-mmkv';
const storage = new MMKV();
storage.set('user', JSON.stringify({ name: 'John' }));
console.log(JSON.parse(storage.getString('user')));
📌 Why This Works:
- Much faster than AsyncStorage.
- Uses native C++ storage backend, reducing JavaScript overhead.
2️⃣ Write Native Modules for Heavy Computations
For operations like image processing, AI inference, or Bluetooth, write a native module.
✅ Android (Kotlin Example)
package com.example
import com.facebook.react.bridge.ReactMethod
import com.facebook.react.bridge.ReactApplicationContext
import com.facebook.react.bridge.ReactContextBaseJavaModule
class MathModule(reactContext: ReactApplicationContext) : ReactContextBaseJavaModule(reactContext) {
override fun getName() = "MathModule"
@ReactMethod
fun multiply(a: Int, b: Int, callback: (Int) -> Unit) {
callback(a * b)
}
}
✅ React Native (Call Native Module in JavaScript)
import { NativeModules } from 'react-native';
const { MathModule } = NativeModules;
MathModule.multiply(5, 6, (result) => {
console.log('Result:', result); // Output: 30
});
📌 Why This Works:
- Heavy calculations are processed natively, freeing up JavaScript resources.
- Reduces UI lag and improves responsiveness.
🚀 Recap: Key Takeaways
✅ Reduce bundle size by using code splitting, lazy loading, and removing unnecessary dependencies.
✅ Optimize API calls with caching, GraphQL, and compression.
✅ Use Native Modules for intensive tasks like storage, computations, and processing.
By following these React Native performance best practices, your app will be faster, leaner, and optimized for the best user experience in 2025! 🚀🔥
🚀 Conclusion: Make Your React Native App Fly!
Optimizing React Native speed isn’t just about fixing one thing—it’s about improving multiple small aspects for the best results. By following these tips, your app will be faster, smoother, and more efficient in 2025.
If you’re looking for a web design company in Mumbai to help with ecommerce website development in Mumbai, check out Prateeksha Web Design—we specialize in crafting high-performance web and mobile experiences.
🔥 Need help building your next project? Whether you need website design in Mumbai, web design services in Mumbai, or a web developer in Mumbai, we’ve got you covered! Let’s build something amazing together. 🚀
About Prateeksha Web Design
Prateeksha Web Design offers specialized services to enhance React Native performance for 2025 through expert consultation and tailored strategies. Our team provides in-depth analysis to identify bottlenecks and implement optimizations, such as efficient state management and component rendering. We focus on leveraging the latest tools and libraries to boost app speed and responsiveness. Comprehensive testing and monitoring ensure sustained performance improvements. Trust us to elevate your React Native projects to new heights!
Interested in learning more? Contact us today.
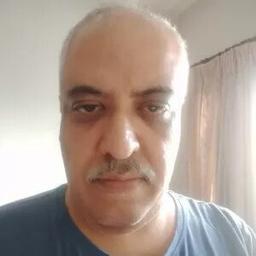