In today’s fast-paced world, handling spreadsheets efficiently is essential for businesses and individuals alike. Whether you’re managing budgets, tracking inventory, or analyzing large datasets, automation can make your life much easier. Enter Python, a programming language celebrated for its simplicity and versatility. With Python, you can automate tedious tasks, streamline workflows, and handle spreadsheet updates effortlessly.
This blog will guide you step-by-step on how to automate spreadsheet updates and data entry with Python, breaking down the concepts in an engaging, accessible way for a 20-year-old audience. Whether you’re a budding coder or just curious about how automation can simplify your life, this guide is for you!
Why Automate Spreadsheet Tasks with Python?
Spreadsheets are widely used for organizing, analyzing, and managing data across industries. They’re versatile and user-friendly but can become overwhelming when repetitive or complex tasks are involved. This is where Python shines. Its ability to handle repetitive, error-prone tasks efficiently makes it an essential tool for spreadsheet management.
Let’s break down why you should use Python for spreadsheet updates and how it can revolutionize your workflow:
1. Time Efficiency
Manual spreadsheet tasks, such as updating cells, formatting rows, or importing/exporting data, can take hours or even days. Python scripts for spreadsheet automation can complete these tasks in seconds.
-
Example: Imagine needing to update a column in a 10,000-row spreadsheet. Manually, this could take hours. With Python:
import pandas as pd df = pd.read_excel('file.xlsx') df['Updated_Column'] = 'New Value' df.to_excel('file_updated.xlsx', index=False)
This simple script updates all rows in seconds, saving you invaluable time.
2. Error Reduction
Humans are prone to mistakes, especially when dealing with repetitive tasks like data entry. Even a small error in financial records or analytics data can have significant consequences. Python eliminates this risk by executing tasks consistently and accurately.
- Example: A typo while entering sales figures could distort your entire sales report. By automating the task, Python ensures:
- Accurate data processing
- Consistent formatting
- Validation to catch anomalies before they escalate
3. Flexibility
Python is highly adaptable and works with a wide range of spreadsheet tools, including Excel, Google Sheets, and even CSV files. Whether you need basic file manipulation or advanced automation, Python offers the flexibility to cater to your specific needs.
- Excel Automation: Libraries like OpenPyXL and XlsxWriter allow you to create and modify Excel files effortlessly.
- Google Sheets: The Google Sheets API enables real-time updates and seamless collaboration.
- CSV Management: Python’s built-in CSV module and Pandas make handling large datasets a breeze.
4. Scalability
Python is built to handle tasks of any scale, from a few rows to millions of data points. As your datasets grow, manual methods become impractical, but Python scales effortlessly.
-
Small Scale: Automate basic tasks like updating column headers or filtering data.
-
Large Scale: Process datasets spanning millions of rows without breaking a sweat.
-
Example: For an e-commerce store tracking customer orders, Python can:
- Process large order datasets
- Analyze trends
- Generate visual reports in seconds
Tools and Libraries for Spreadsheet Automation
Python’s ecosystem is packed with libraries tailored for spreadsheet task automation. Let’s dive into some of the most popular ones:
1. Pandas
Pandas is the Swiss Army knife of data manipulation in Python. It simplifies reading, writing, filtering, and analyzing spreadsheet data.
- Key Features:
- Supports Excel, CSV, and other data formats.
- Provides robust tools for data cleaning and transformation.
- Example: Filter out rows where sales are below a certain threshold:
df = pd.read_excel('sales.xlsx') filtered_df = df[df['Sales'] > 1000] filtered_df.to_excel('filtered_sales.xlsx', index=False)
2. OpenPyXL
OpenPyXL is specifically designed for Excel automation. It’s perfect for creating, reading, and modifying Excel files, as well as formatting and styling sheets.
-
Key Features:
- Modify individual cells or entire ranges.
- Add charts, formulas, and pivot tables.
-
Example: Update a specific cell in an Excel file:
from openpyxl import load_workbook workbook = load_workbook('file.xlsx') sheet = workbook.active sheet['A1'] = 'Updated Value' workbook.save('file_updated.xlsx')
3. XlsxWriter
For creating visually appealing and highly formatted Excel reports, XlsxWriter is an excellent choice.
-
Key Features:
- Advanced formatting (colors, borders, fonts).
- Adding charts and graphs.
-
Example: Generate a formatted sales report:
import xlsxwriter workbook = xlsxwriter.Workbook('sales_report.xlsx') worksheet = workbook.add_worksheet() worksheet.write('A1', 'Product') worksheet.write('B1', 'Sales') worksheet.write('A2', 'Widget') worksheet.write('B2', 1500) workbook.close()
4. Google Sheets API
Google Sheets is a collaborative platform, and Python’s Google Sheets API makes it possible to automate tasks on this platform.
-
Key Features:
- Real-time updates and data sharing.
- Seamless integration with other Google Workspace tools.
-
Example: Update a cell in a Google Sheet:
import gspread from google.oauth2.service_account import Credentials creds = Credentials.from_service_account_file('credentials.json') client = gspread.authorize(creds) sheet = client.open('My Sheet').sheet1 sheet.update('A1', 'New Value')
5. PyAutoGUI
For tasks that require interacting with GUI-based spreadsheet software (e.g., manually opening Excel and clicking buttons), PyAutoGUI can simulate keyboard and mouse actions.
-
Key Features:
- Automates GUI interactions.
- Perfect for non-programmable tasks.
-
Example: Automate a repetitive keyboard shortcut:
import pyautogui pyautogui.click(x=100, y=200) # Click at specific screen coordinates pyautogui.typewrite('Automated Input') # Simulate typing
Setting Up Your Environment
Before diving into automation, let’s ensure your environment is ready:
- Install Python: If you don’t have Python installed, download it from Python.org.
- Set Up a Code Editor: Tools like Visual Studio Code or PyCharm make coding easier and more fun.
- Install Required Libraries: Use
pip
to install libraries:pip install pandas openpyxl gspread google-auth pyautogui
Now that you’re set up, let’s dive into examples!
Automate Excel Updates with Python Scripts
Let’s start with an example of updating Excel files using Python. Imagine you’re a data analyst who needs to update weekly sales figures. Here’s how you can automate it:
Step 1: Import Required Libraries
import pandas as pd
from openpyxl import load_workbook
Step 2: Load and Update the Excel File
# Load the Excel file
file_path = 'sales_data.xlsx'
workbook = load_workbook(file_path)
sheet = workbook.active
# Update specific cells
sheet['B2'] = 'Updated Value'
workbook.save(file_path)
print("Spreadsheet updated successfully!")
This simple script updates a specific cell in your Excel sheet. You can extend it to handle larger datasets or complex updates.
Real-Life Use Case
For businesses, Python tools for Excel automation are game-changers. Prateeksha Web Design often uses such scripts to streamline client data and generate performance reports effortlessly.
Automate Google Sheets with Python
Working on Google Sheets? Python has you covered. Let’s say you’re managing a shared budget tracker and want to automate monthly updates.
Step 1: Enable Google Sheets API
- Go to Google Cloud Console, create a project, and enable the Google Sheets API.
- Download the credentials.json file for authentication.
Step 2: Install Required Libraries
pip install gspread google-auth
Step 3: Automate Updates
import gspread
from google.oauth2.service_account import Credentials
# Authenticate
creds = Credentials.from_service_account_file('credentials.json')
client = gspread.authorize(creds)
# Access the spreadsheet
sheet = client.open('Budget Tracker').sheet1
# Update a cell
sheet.update('A2', 'Updated Value')
print("Google Sheet updated successfully!")
Why It’s Useful
Python for spreadsheet updates in Google Sheets is particularly handy for remote teams. You can automate updates, share insights, and keep everyone on the same page.
Automate Data Entry with Python
If you’re drowning in manual data entry, Python can save the day. Here’s how Python data entry automation works:
Example: Importing CSV Data into Excel
import pandas as pd
# Load CSV data
csv_data = pd.read_csv('data.csv')
# Save to Excel
csv_data.to_excel('data.xlsx', index=False)
print("Data entry automated successfully!")
This script imports data from a CSV file and converts it into an Excel spreadsheet, perfect for streamlining bulk data uploads.
Advanced Spreadsheet Management with Python
As you grow more comfortable with Python, you can explore advanced spreadsheet task automation:
1. Dynamic Dashboards
Use Python to create interactive dashboards with real-time updates.
2. Data Validation
Automate data validation to ensure consistent and error-free entries.
3. Bulk Updates
Scripts can update thousands of rows or columns in seconds.
4. Custom Workflows
Combine libraries like Pandas and OpenPyXL to craft workflows tailored to your needs.
Real-Life Applications of Python Automation
Python's versatility makes it an ideal choice for automating a wide range of tasks across industries. Let’s delve into how Python automation is transforming different fields and the impact it can have:
1. E-commerce Management
E-commerce businesses deal with vast amounts of data, including inventory levels, sales figures, and customer information. Managing these manually is both time-consuming and error-prone. Python simplifies these tasks with automation scripts.
-
Inventory Updates: Python can track inventory in real-time and update stock levels automatically.
-
Example: A script pulls sales data from your website, adjusts inventory in your Excel or database, and generates an updated inventory report.
import pandas as pd # Load sales data sales_data = pd.read_csv('sales.csv') # Adjust inventory based on sales inventory_data = pd.read_excel('inventory.xlsx') inventory_data['Stock'] -= sales_data['Quantity'] inventory_data.to_excel('updated_inventory.xlsx', index=False) print("Inventory updated successfully!")
-
-
Sales Tracking: Python integrates with e-commerce platforms like Shopify or WooCommerce to collect and analyze sales trends.
At Prateeksha Web Design, we’ve developed scripts to automate these processes for our clients, enabling them to make data-driven decisions quickly.
2. Finance
Financial management often involves repetitive tasks like tracking expenses, generating reports, and reconciling accounts. Python’s finance automation capabilities reduce manual effort and improve accuracy.
- Budgeting: Automate the categorization of expenses and compare them against budgets.
- Expense Tracking: Python scripts can scan transaction logs or bank statements and create summary reports.
- Example:
df = pd.read_csv('transactions.csv') budget_summary = df.groupby('Category').sum() budget_summary.to_excel('budget_summary.xlsx') print("Budget summary generated!")
- Example:
- Real-Time Dashboards: With Python and visualization tools like Matplotlib or Plotly, you can create live financial dashboards.
For businesses, Python ensures compliance and accuracy in financial records, saving hours of manual calculations.
3. Education
Educational institutions and instructors handle a variety of repetitive tasks, from grading to attendance tracking. Python streamlines these processes, allowing educators to focus on teaching.
-
Grading Automation: Python scripts can evaluate exam results, assign grades, and generate detailed reports.
-
Example:
import pandas as pd # Load student scores scores = pd.read_excel('scores.xlsx') scores['Grade'] = scores['Marks'].apply(lambda x: 'A' if x > 90 else ('B' if x > 75 else 'C')) scores.to_excel('graded_scores.xlsx', index=False) print("Grading completed!")
-
-
Attendance Records: Automate attendance tracking by integrating Python with systems like Google Sheets or learning management systems (LMS).
-
Resource Management: Python helps librarians track book inventories or manage digital resources efficiently.
Educational institutions benefit from Python for data management automation, enhancing operational efficiency.
4. Healthcare
In healthcare, where data accuracy and confidentiality are critical, Python enables automated workflows that streamline patient data management and administrative tasks.
-
Patient Data Management: Automate the process of updating patient records, scheduling appointments, and sending reminders.
-
Medical Reports: Generate detailed patient reports and summaries from diagnostic data.
-
Inventory Control: Track the usage of medical supplies and ensure adequate stock levels.
-
Example:
import pandas as pd # Load supply usage data usage = pd.read_excel('usage.xlsx') inventory = pd.read_excel('inventory.xlsx') # Update inventory inventory['Remaining'] -= usage['Used'] inventory.to_excel('updated_inventory.xlsx', index=False) print("Medical inventory updated!") ``` By automating these tasks, healthcare providers can focus on delivering better care while maintaining data integrity.
-
Tips for Success in Python Automation
If you’re excited to start automating tasks with Python, here are some practical tips to help you succeed:
1. Start Small
Don’t dive into complex automation projects immediately. Begin with simple tasks, like automating updates for small spreadsheets or importing/exporting data.
- Example: Automate a weekly sales summary before tackling larger datasets.
- Why It Works: You’ll build confidence and avoid feeling overwhelmed.
2. Leverage Libraries
Python’s strength lies in its vast library ecosystem. Familiarize yourself with essential libraries for automation:
- Pandas for data manipulation.
- OpenPyXL for Excel automation.
- Google Sheets API for cloud-based automation.
These libraries act as your toolkit, making tasks simpler and more efficient.
3. Practice Regularly
Automation is a skill, and practice is key to mastering it. Experiment with different types of tasks:
- Update formulas or styles in Excel.
- Create summary reports.
- Automate email notifications based on spreadsheet updates.
The more you practice, the more confident you’ll become in your abilities.
4. Stay Updated
Technology evolves rapidly, and Python is no exception. Follow platforms like Program Geeks to stay informed about:
- New libraries.
- Updated best practices.
- Advanced automation techniques.
By keeping up-to-date, you’ll always be ready to tackle new challenges with the latest tools.
Conclusion
Handling spreadsheet updates and data entry no longer needs to be a tedious chore. With Python tools for Excel automation and Google Sheets integration, you can transform your workflows, save time, and reduce errors. Whether you’re managing small tasks or building automated workflows with Python scripts, the possibilities are endless.
Prateeksha Web Design specializes in leveraging technology like Python to streamline business operations. From e-commerce stores to corporate dashboards, we empower businesses to succeed with smarter tools. If you’re ready to take your spreadsheet management Python skills to the next level, start experimenting today. The world of automation awaits!
About Prateeksha Web Design
- Automate data entry process with Python scripting.
- Streamline spreadsheet updates through Python integration.
- Efficiently handle bulk data manipulation using Python libraries.
- Implement error handling mechanisms for accurate data entry.
- Enhance data management practices with Python for effective spreadsheet updates.
Interested in learning more? Contact us today.
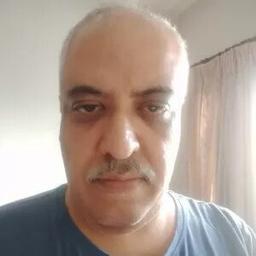