So, you’re diving into the world of Word document automation with .NET Core and C#? Great choice! Whether you’re building reports, documentation, or legal contracts, automating the Table of Contents (TOC) in Word is a game-changer.
Manually updating a TOC in Word is like herding cats—frustrating, chaotic, and never quite right. But fear not! You can create, modify, and update a Table of Contents programmatically using Open XML SDK (OOXML) and C#.
By the end of this guide, you’ll have a working solution for handling TOCs programmatically—saving you hours of tedious manual work.
🚀 Why Automate the Table of Contents in Word?
If you've ever worked with large Word documents, you know the struggle of keeping the Table of Contents (TOC) updated. You might add a new chapter, rename a section, or restructure an entire document—only to realize that your TOC is outdated.
Manually updating a TOC can be tedious and error-prone, especially if you're dealing with hundreds or thousands of pages. That’s why automating TOC generation using .NET Core and C# is a game-changer. Let's break it down with some solid reasons why automating this process is worth it.
✅ 1. Efficiency: Save Time, Work Smarter!
Imagine you're handling multiple documents—maybe reports, legal contracts, or an e-commerce product catalog—each with dozens of headings and sections.
Scenario 1: Manual TOC Updates
- Open each document individually.
- Click "Update TOC" manually.
- Fix errors where Word fails to recognize headings.
- Repeat for each document (ugh 😩).
Scenario 2: Automating TOC with C# and Open XML
- Run a script that updates all documents with one click.
- Every TOC in every document updates instantly and accurately.
🚀 Result? You save hours (or even days) of manual effort. Time that can be better spent on real work—or even a coffee break. ☕
✅ 2. Consistency: No More Human Errors
Have you ever:
❌ Missed a heading in your TOC?
❌ Seen duplicate or incorrectly formatted TOC entries?
❌ Had page numbers in your TOC not match the actual content?
These errors are common when updating TOCs manually. Even experienced professionals make mistakes when dealing with long documents.
Automating TOC creation ensures:
✅ Every TOC follows the same structure and style.
✅ All headings appear correctly without duplicates.
✅ Page numbers update automatically whenever content changes.
No more scrambling to fix errors at the last minute before a client meeting!
✅ 3. Scalability: Handle Large Documents with Ease
Whether you're working on business reports, research papers, legal contracts, or e-books, the size of your document shouldn’t dictate how difficult it is to manage.
🔹 A small document (5-10 pages)? A manual TOC update isn’t too painful.
🔹 A medium document (50-100 pages)? You might still manage it manually.
🔹 A large document (500+ pages)? 😨 Forget it—manual TOC updates are a nightmare!
Automating TOC generation means:
✅ Your document size doesn’t matter—TOC updates happen instantly.
✅ You don’t have to worry about missing sections or broken links.
✅ Whether you're working on one document or a thousand, the process remains the same.
This is especially useful in industries like:
- Publishing (e-books, academic research, technical manuals).
- Corporate (business reports, whitepapers, legal contracts).
- E-commerce (product catalogs, invoices, receipts).
✅ 4. Integrations: Automate Across Systems
One of the coolest things about .NET Core and Open XML SDK is that you can integrate TOC automation with other business applications.
Imagine generating a TOC dynamically as part of a reporting system that pulls data from:
📌 A CRM (e.g., auto-generating sales reports).
📌 An ERP system (e.g., invoice generation).
📌 A Content Management System (CMS) (e.g., creating documentation automatically).
📌 An e-commerce website development system (e.g., auto-generating product catalogs).
🚀 Real-World Example:
Let’s say you run an e-commerce website in Mumbai that sells customized business reports. You could automate report generation, including a dynamically updated TOC, with one click!
🔹 Need a structured report for clients? Auto-generate TOC.
🔹 Want to create product manuals for an online store? TOC updates automatically.
🔹 Building legal contracts that change frequently? No more manual TOC fixes.
🔧 Setting Up the Environment
Now that you're convinced that automating a Table of Contents (TOC) in Word using .NET Core and C# is the way to go, let’s get everything set up.
📌 Prerequisites
Before we start writing code, make sure you have the following:
✅ .NET Core SDK – The latest version is recommended to take advantage of new features and performance improvements.
✅ Open XML SDK (DocumentFormat.OpenXml) – This allows you to manipulate Word documents without needing Microsoft Word installed.
✅ Visual Studio or your favorite C# IDE – A good IDE makes development easier with built-in debugging and NuGet package management.
📥 Installing Open XML SDK
To interact with Word documents programmatically, we need the Open XML SDK. Install it via NuGet:
dotnet add package DocumentFormat.OpenXml
Or, if you prefer the NuGet Package Manager in Visual Studio:
1️⃣ Open your project.
2️⃣ Go to Manage NuGet Packages.
3️⃣ Search for DocumentFormat.OpenXml.
4️⃣ Click Install.
Once installed, you’re ready to start working with Word documents.
📝 Creating a Table of Contents in Word Programmatically
Now comes the fun part—writing C# code to generate and modify a Table of Contents in a Word document.
📌 Step 1: Create a New Word Document
Let’s start by creating a new Word document programmatically using Open XML.
using DocumentFormat.OpenXml;
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Wordprocessing;
using System;
class Program
{
static void Main()
{
string filePath = "MyWordDocument.docx";
using (WordprocessingDocument wordDocument = WordprocessingDocument.Create(filePath, WordprocessingDocumentType.Document))
{
MainDocumentPart mainPart = wordDocument.AddMainDocumentPart();
mainPart.Document = new Document();
Body body = new Body();
mainPart.Document.Append(body);
}
Console.WriteLine("Word document created successfully!");
}
}
💡 What’s Happening Here?
🔹 We create a new Word document using Open XML.
🔹 We add a main document part (which holds the actual content).
🔹 We append an empty body, so the document structure is ready for content.
Run this program, and you'll have a blank Word document created programmatically.
📌 Step 2: Add Headings to the Document
Since a Table of Contents is based on headings, we need to add some headings to our document.
static void AddHeading(MainDocumentPart mainPart, string text, string style)
{
Body body = mainPart.Document.Body;
Paragraph paragraph = new Paragraph();
Run run = new Run();
run.Append(new Text(text));
ParagraphProperties properties = new ParagraphProperties();
properties.ParagraphStyleId = new ParagraphStyleId() { Val = style };
paragraph.Append(properties);
paragraph.Append(run);
body.Append(paragraph);
}
using (WordprocessingDocument wordDocument = WordprocessingDocument.Open("MyWordDocument.docx", true))
{
MainDocumentPart mainPart = wordDocument.MainDocumentPart;
AddHeading(mainPart, "Introduction", "Heading1");
AddHeading(mainPart, "Chapter 1: Getting Started", "Heading1");
AddHeading(mainPart, "Chapter 2: Advanced Techniques", "Heading1");
}
💡 What’s Happening Here?
🔹 We define a method AddHeading that inserts text formatted as a Word heading style.
🔹 The style name (e.g., "Heading1") is essential for Word to recognize it in the TOC.
🔹 The document is re-opened in edit mode (true
parameter) to append content.
At this point, your document now has formatted headings, which will be detected by the TOC.
📌 Step 3: Insert the Table of Contents
Now, let’s insert the TOC using Open XML.
static void AddTableOfContents(MainDocumentPart mainPart)
{
Body body = mainPart.Document.Body;
Paragraph tocParagraph = new Paragraph();
Run run = new Run();
FieldChar fieldCharBegin = new FieldChar() { FieldCharType = FieldCharValues.Begin };
Run runBegin = new Run(fieldCharBegin);
Run runToc = new Run();
runToc.Append(new Text(" TOC \\o \"1-3\" \\h \\z \\u ")); // TOC field code
FieldChar fieldCharSeparate = new FieldChar() { FieldCharType = FieldCharValues.Separate };
Run runSeparate = new Run(fieldCharSeparate);
Run runEnd = new Run(new FieldChar() { FieldCharType = FieldCharValues.End });
tocParagraph.Append(runBegin, runToc, runSeparate, runEnd);
body.Append(tocParagraph);
}
using (WordprocessingDocument wordDocument = WordprocessingDocument.Open("MyWordDocument.docx", true))
{
MainDocumentPart mainPart = wordDocument.MainDocumentPart;
AddTableOfContents(mainPart);
}
💡 What’s Happening Here?
🔹 We create a TOC field using Open XML’s FieldChar
class.
🔹 The TOC \\o "1-3" \\h \\z \\u
command generates the TOC based on Heading 1-3 levels.
🔹 Word will now recognize this as a TOC, but it won’t update automatically.
🔄 Updating the Table of Contents in Word
At this point, the TOC exists, but you must manually update it by pressing F9 in Word.
To automate this update, you can use Microsoft Word Interop:
using Microsoft.Office.Interop.Word;
Application wordApp = new Application();
Document doc = wordApp.Documents.Open("MyWordDocument.docx");
foreach (TableOfContents toc in doc.TablesOfContents)
{
toc.Update();
}
doc.Save();
doc.Close();
wordApp.Quit();
This refreshes the TOC automatically upon opening the document.
🛠️ Modifying the TOC Appearance
Want to customize how your TOC looks? Modify the field codes:
runToc.Append(new Text(" TOC \\o \"1-3\" \\h \\z \\u ")); // Default TOC
runToc.Append(new Text(" TOC \\o \"1-3\" \\h \\z ")); // No underline
runToc.Append(new Text(" TOC \\o \"1-3\" \\h ")); // No hyperlinks
Field Code Breakdown:
\o "1-3"
– Includes headings 1 to 3.\h
– Adds hyperlinks to sections.\z
– Hides tab leader.\u
– Uses underline.
Adjust these to match your document’s style.
🌟 Final Thoughts
You did it! 🎉 You now know how to:
✅ Create a Word document in .NET Core using C#
✅ Insert headings programmatically
✅ Generate a Table of Contents dynamically
✅ Modify and update the TOC for a professional look
This solution is perfect for business reports, e-commerce invoices, documentation, or large-scale content generation. Speaking of e-commerce, if you're looking for the best web design company in Mumbai, Prateeksha Web Design can build stunning websites, including e-commerce website design in Mumbai and enterprise-level applications.
🚀 Ready to take your document automation to the next level? Let’s build something awesome!
🔹 Need a website? Check out Prateeksha Web Design
🔹 Have questions? Drop a comment below!
About Prateeksha Web Design
Prateeksha Web Design offers specialized services for creating and updating dynamic tables of contents in Word documents through .NET Core and C#. Our expertise includes developing custom solutions that automate the process, ensuring seamless integration with existing applications. We provide comprehensive guidance on using libraries such as Open XML SDK for efficient document manipulation. Our services enhance user experience by enabling easy navigation and content management in Word files. Trust us to empower your document workflows with our tailored programming solutions.
Interested in learning more? Contact us today.
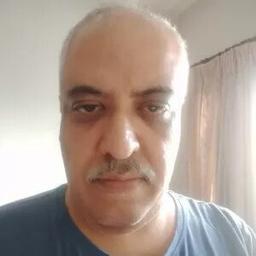