In today’s data-driven world, retrieving information quickly and efficiently from web services is an essential skill for programgeeks and businesses alike. Whether you’re building dashboards, automating workflows, or enhancing user experiences, Python stands out as a versatile tool for automating data collection and integration. This blog will serve as your comprehensive guide to mastering Python web service automation with detailed steps, best practices, and examples to help you retrieve data from APIs, process it, and integrate it seamlessly into your projects.
What Are Web Services and Why Automate Their Data Retrieval?
Web Services: A Quick Overview
Web services are online tools or APIs (Application Programming Interfaces) that enable two systems to communicate with each other over the internet. These systems could range from your favorite weather app fetching weather updates to an e-commerce platform like Shopify retrieving real-time inventory data.
For example:
- Weather APIs deliver forecasts.
- Social Media APIs provide user activity.
- E-commerce APIs power order tracking and inventory.
Automation simplifies repetitive tasks like fetching this data, enabling you to focus on actionable insights rather than manual downloads.
Why Automate Data Retrieval?
Automating data retrieval has several advantages:
- Efficiency: Automate repetitive tasks like pulling data daily or hourly.
- Accuracy: Reduce manual errors by relying on consistent scripts.
- Scalability: Handle large datasets or multiple endpoints without breaking a sweat.
- Integration: Use retrieved data for analytics, dashboards, or other tools in real-time.
For businesses, like the ones we work with at Prateeksha Web Design, automating web service data collection reduces time-to-insight and ensures seamless UX/UI integration.
Setting Up Python for Automating Data Retrieval
Installing Python
If you don’t already have Python installed, visit python.org and download the latest version. Python 3.x is recommended for most tasks.
Setting Up the Environment
Install these essential libraries for working with web services:
requests
- For making HTTP requests.pip install requests
pandas
- For data manipulation.pip install pandas
json
(built-in) - For parsing JSON responses.schedule
- For automating script execution.pip install schedule
Pro Tip for Geeks Program Enthusiasts: Use a virtual environment (venv
) to manage dependencies for specific projects.
Step-by-Step Guide to Automating Data Retrieval
Step 1: Understand the API You’re Working With
Start by reviewing the API documentation of the web service. This documentation usually contains:
- Endpoints: URLs for fetching specific data.
- Authentication: API keys or OAuth tokens.
- Rate Limits: Maximum number of requests allowed per minute/hour.
- Response Format: JSON or XML data structures.
For example, let’s fetch data from a mock weather API. An endpoint might look like this:
https://api.weather.com/v3/weather?city=Mumbai&apikey=YOUR_API_KEY
Step 2: Write a Python Script to Fetch Data
Here’s a simple script to fetch and parse JSON data:
import requests
import json
# Replace YOUR_API_KEY with your actual API key
url = "https://api.weather.com/v3/weather?city=Mumbai&apikey=YOUR_API_KEY"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print("Weather Data:", json.dumps(data, indent=4))
else:
print("Failed to retrieve data:", response.status_code)
Key Takeaways:
- Always check the
status_code
to ensure the request succeeded. - Use
json.dumps()
for a formatted display of JSON data.
Step 3: Automate Authentication
Most APIs require authentication using an API key or tokens. Here’s how to securely integrate authentication:
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
response = requests.get(url, headers=headers)
For more secure storage, consider using environment variables with the os
module:
import os
api_key = os.getenv("API_KEY")
Step 4: Process and Save the Data
Once the data is fetched, use libraries like pandas
to clean and store it:
import pandas as pd
# Example data
data = [{"date": "2024-12-27", "temperature": 28}, {"date": "2024-12-28", "temperature": 29}]
# Create a DataFrame
df = pd.DataFrame(data)
# Save to CSV
df.to_csv("weather_data.csv", index=False)
print("Data saved to weather_data.csv")
Step 5: Schedule the Script
Automate the execution using the schedule
library:
import schedule
import time
def job():
print("Fetching data...")
# Call your data fetching function here
schedule.every().day.at("09:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
Advanced Techniques for Program Geeks
1. Handling API Rate Limits
Use Python to pause requests if you hit the rate limit:
import time
for _ in range(10): # Simulating multiple API calls
response = requests.get(url)
if response.status_code == 429: # Rate limit error
print("Rate limit hit. Sleeping for 60 seconds.")
time.sleep(60)
else:
print(response.json())
2. Error Handling
Use try
and except
blocks to catch and log errors:
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as err:
print("HTTP Error:", err)
Use Cases of Python Web Service Automation
1. E-Commerce Data Integration
Retrieve inventory, pricing, or customer data from Shopify APIs to enhance your e-commerce site.
2. Social Media Analytics
Fetch engagement stats using Instagram or Twitter APIs to drive data-backed marketing strategies.
3. Financial Data Pipelines
Automate stock market data retrieval for financial dashboards.
At Prateeksha Web Design, we specialize in using Python to streamline data workflows for our clients, making their websites smarter and more efficient.
Popular Tools and Libraries for API Automation
When automating data retrieval or working with APIs, leveraging the right tools and libraries can significantly enhance your productivity and efficiency. Here’s a detailed look at three popular tools and libraries—Postman, FastAPI, and Celery—and how they fit into the Python API automation ecosystem.
1. Postman: Testing and Exploring APIs
Postman is a powerful, user-friendly tool for testing and interacting with APIs before integrating them into your automation pipeline. It provides a graphical interface that simplifies the process of constructing, sending, and inspecting API requests.
Key Features of Postman
- Request Building: You can create and save HTTP requests (GET, POST, PUT, DELETE) with parameters, headers, and authentication credentials.
- Response Inspection: View formatted API responses, including JSON, XML, and raw formats, to ensure your endpoints work as expected.
- Automated Testing: Use built-in test scripts to verify API behavior.
- Collection Runner: Execute a series of saved API calls sequentially, which is useful for workflows and integration testing.
- Environment Variables: Manage API keys and URLs for multiple environments (development, staging, production).
Why Use Postman for API Automation?
- Debugging Made Easy: Postman highlights errors in API requests, such as incorrect headers or payloads, so you can troubleshoot before coding.
- Pre-Coding Validation: Ensures that the API works as intended, saving you time when writing Python scripts.
- Team Collaboration: Share API collections and collaborate with team members.
Practical Example
If you’re automating data retrieval from a weather API, you can use Postman to:
- Build the request URL with parameters (e.g., city, API key).
- Inspect the JSON response structure to understand how the data is organized.
- Save the working request to use as a reference when coding your Python script.
Learn More: Postman Documentation
2. FastAPI: Build APIs to Complement Automation
FastAPI is a modern Python framework for building APIs quickly and efficiently. It is designed to create robust, high-performance web services with minimal code. FastAPI can also complement your automation pipeline by enabling you to build custom APIs for internal tools or integrations.
Key Features of FastAPI
- High Performance: Built on Starlette and Pydantic, it provides asynchronous support and fast execution.
- Automatic Documentation: Generates interactive API documentation with Swagger UI and ReDoc.
- Type Safety: Leverages Python type hints for validation and code clarity.
- Ease of Use: Requires minimal boilerplate code to set up endpoints.
- Scalability: Ideal for microservices and large-scale applications.
Why Use FastAPI in API Automation?
- Custom API Creation: If a third-party API doesn’t meet all your needs, you can build your own using FastAPI to preprocess, filter, or combine data.
- Data Transformation: Use FastAPI to format, validate, or transform data retrieved from other APIs before storing or passing it to another system.
- Internal Tools: Create internal APIs that interface with your database or services, allowing your automation scripts to retrieve data efficiently.
Practical Example
Imagine you’re retrieving product data from multiple e-commerce APIs for an inventory management system. You can:
- Use FastAPI to create an internal endpoint that aggregates data from all sources.
- Implement custom filtering logic within FastAPI to return only relevant data to your Python scripts.
- Automate fetching the filtered data using Python.
from fastapi import FastAPI
app = FastAPI()
@app.get("/products/")
def get_products(category: str = None):
# Logic to fetch and filter products
return {"category": category, "products": ["item1", "item2"]}
Learn More: FastAPI Documentation
3. Celery: Task Queue and Scheduling Management
Celery is an asynchronous task queue/job queue library written in Python. It’s ideal for automating and scheduling tasks that don’t need immediate processing, such as periodic API calls or data pipeline updates.
Key Features of Celery
- Task Queues: Define tasks that can run asynchronously or be scheduled for later execution.
- Integration: Works seamlessly with message brokers like RabbitMQ or Redis.
- Periodic Tasks: Schedule recurring tasks using Celery Beat.
- Scalability: Handle thousands of tasks concurrently.
- Error Handling: Retry failed tasks automatically.
Why Use Celery in API Automation?
- Efficient Scheduling: Automate frequent API calls (e.g., hourly data fetching).
- Asynchronous Processing: Offload long-running tasks, such as data cleaning or report generation, to Celery.
- Parallelization: Process multiple API responses simultaneously, reducing execution time.
Practical Example
Suppose you need to fetch sales data from an e-commerce API every hour and save it to a database. Celery allows you to:
- Define a task for data fetching and database insertion.
- Schedule the task to run hourly using Celery Beat.
- Monitor and retry failed tasks automatically.
from celery import Celery
app = Celery('tasks', broker='redis://localhost:6379/0')
@app.task
def fetch_sales_data():
# Logic to fetch and store data
print("Sales data retrieved and saved.")
Learn More: Celery Documentation
Combining These Tools for Maximum Impact
For a comprehensive Python API integration tutorial, you can use these tools together:
- Postman: Test and refine your API requests.
- FastAPI: Build custom endpoints to preprocess or combine data.
- Celery: Schedule periodic data retrieval and automate complex workflows.
Conclusion
Automating data retrieval with Python transforms tedious manual tasks into efficient, scalable workflows. By mastering these techniques, you’ll not only save time but also unlock new possibilities in data integration and analytics. For programming geeks, this skill is a must-have.
Want to implement advanced automation strategies for your e-commerce or data-heavy projects? At Prateeksha Web Design, we combine cutting-edge tools with expert design to deliver seamless solutions. Let us help you turn your data into actionable insights.
About Prateeksha Web Design
- Web scraping with Python
- Automated data extraction
- Python scripts for web services
- Data retrieval automation
- Prateeksha Web Design services
Interested in learning more? Contact us today.
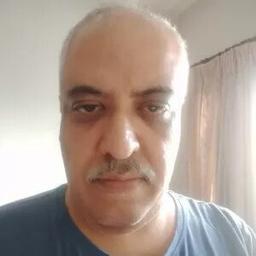