If you’ve ever spent hours manually entering data into spreadsheets, updating databases, or filling out forms, you know how frustrating and time-consuming these tasks can be. But here’s the good news: Python, one of the most versatile programming languages, can take these repetitive tasks off your plate. By automating data entry with Python, you can free up time for more meaningful work and reduce the risk of human error. Let’s dive deep into this transformative process, step-by-step, in a way that’s both engaging and easy to understand.
Why Python for Data Entry Automation? (Explained in Detail)
Python has gained massive popularity as the go-to language for automation, and for good reason. Its combination of simplicity, flexibility, and a rich ecosystem of libraries makes it an excellent choice for automating repetitive tasks like data entry. Whether you're a beginner just dipping your toes into coding or a seasoned programming geek (hello, ProgramGeeks!), Python has something to offer everyone.
Let’s dive deeper into why Python stands out for data entry automation:
1. User-Friendly Syntax
One of Python’s biggest strengths is its readable and beginner-friendly syntax. Here’s what makes it great:
- Easy to Understand: Unlike many other programming languages, Python uses plain English keywords. For example, instead of confusing symbols or brackets, it uses words like
for
,if
, andwhile
.- Example:
for item in list:
is more intuitive than its equivalents in other languages.
- Example:
- Minimal Boilerplate: Python scripts are concise. You can write a program to automate a task in just a few lines of code.
Imagine you’re a 20-year-old exploring coding for the first time. Python feels less like learning a new language and more like writing instructions in plain English. For example:
# Adding two numbers in Python
a = 5
b = 10
print(a + b) # Outputs: 15
This simplicity makes Python an ideal entry point for automating data entry tasks, even if you’ve never coded before.
2. Versatility
Python’s versatility is unmatched. It’s not just limited to one type of automation but can handle a variety of tasks seamlessly. Here’s how it shines across different data entry scenarios:
- Spreadsheets: Automate tasks like updating rows, generating reports, or cleaning data using libraries like
pandas
andopenpyxl
. - Databases: Use Python to insert, update, or query data in SQL or NoSQL databases efficiently.
- Online Forms: Automate filling out forms and submitting them using
selenium
. - API Integration: Automate data fetching or submission using Python’s
requests
library.
Whether you’re managing thousands of rows in Excel, updating a MySQL database, or submitting dozens of online forms, Python can handle it all, making it a true all-rounder.
3. Extensive Libraries and Frameworks
Python boasts a rich ecosystem of libraries specifically designed for automation. These libraries act like ready-made tools, allowing you to focus on the task rather than reinventing the wheel.
Key Libraries for Data Entry Automation:
-
pandas
:- Perfect for manipulating large datasets in spreadsheets or CSV files.
- Use it for filtering, cleaning, or transforming data effortlessly.
- Example:
import pandas as pd data = pd.read_csv('data.csv') print(data.head()) # Displays the first 5 rows
-
openpyxl
:- Specifically built for Excel automation.
- Allows you to read, write, and format
.xlsx
files programmatically. - Example:
import openpyxl workbook = openpyxl.load_workbook('file.xlsx') sheet = workbook.active print(sheet['A1'].value) # Reads the value in cell A1
-
sqlite3
:- Built into Python, this library is excellent for lightweight database management.
- Example:
import sqlite3 conn = sqlite3.connect('data.db') cursor = conn.cursor() cursor.execute("CREATE TABLE IF NOT EXISTS users (id INTEGER, name TEXT)") conn.commit()
-
selenium
:- Best for automating web-based tasks, like filling out and submitting forms.
- Example:
from selenium import webdriver driver = webdriver.Chrome() driver.get('https://example.com/form')
-
requests
:- Ideal for automating interactions with APIs or web services.
- Example:
import requests response = requests.get('https://api.example.com/data') print(response.json())
These libraries give Python an edge by making it possible to automate almost any data entry-related task, whether it involves working offline (spreadsheets, databases) or online (forms, APIs).
Why Python is a Favorite for ProgramGeeks and Data Entry Professionals
Python is a favorite among programming geeks and professionals because it simplifies complex workflows. Instead of spending hours manually handling data, Python scripts can:
- Eliminate Human Error: Automation ensures consistency and accuracy in data entry.
- Save Time: Scripts can handle thousands of operations in seconds.
- Scale Effortlessly: Automate tasks for small-scale projects or enterprise-level workflows without changing tools.
- Integrate Seamlessly: Combine Python scripts with other software tools like Google Sheets, CRMs, or cloud storage.
Real-World Example: Automating Customer Data Updates
Imagine you’re working for an e-commerce company and need to update customer records weekly. The old way involves:
- Exporting data from a CRM.
- Manually editing it in Excel.
- Importing the changes back into the database.
With Python, you can automate this entire workflow:
- Use
pandas
to process the exported data. - Use
sqlite3
to update the database records. - Use
smtplib
to email a summary report to your manager.
This is why Python has become the Swiss Army knife for automation, helping businesses of all sizes save time and increase productivity.
Key Takeaways: Why Python?
- Beginner-Friendly: Perfect for new coders thanks to its simple syntax.
- Powerful: Handles a wide range of tasks, from spreadsheet automation to database updates and web interactions.
- Efficient: Python’s libraries enable you to write fewer lines of code while achieving more.
- Scalable: Suitable for both small personal projects and large enterprise workflows.
With Python, you don’t need to be a professional coder to automate tasks. Tools like pandas
and selenium
bring automation within reach for anyone willing to learn, making Python an ideal choice for anyone looking to simplify data entry tasks.
1. Automating Spreadsheets with Python
Spreadsheets are a cornerstone of data management. Tools like Excel and Google Sheets are great, but they’re not designed for repetitive, large-scale tasks. Python, on the other hand, is perfect for this job.
Key Python Libraries for Spreadsheet Automation
openpyxl
: Ideal for working with Excel files.pandas
: Great for manipulating large datasets with ease.gspread
: Perfect for interacting with Google Sheets.
Use Case: Automating Data Entry in Excel
Imagine you receive daily sales reports in Excel format. You need to clean the data, perform calculations, and generate a summary report. Doing this manually can be tedious, but Python can handle it in seconds.
Here’s a simple Python script to automate data entry in Excel:
import openpyxl
# Load the workbook and select the sheet
workbook = openpyxl.load_workbook("sales_data.xlsx")
sheet = workbook.active
# Example: Add a total column for each row
for row in sheet.iter_rows(min_row=2, max_row=sheet.max_row, min_col=2, max_col=4):
total = sum(cell.value for cell in row if cell.value is not None)
sheet.cell(row=row[0].row, column=5).value = total
# Save the workbook
workbook.save("updated_sales_data.xlsx")
print("Data entry automated successfully!")
Pro Tips for Spreadsheet Automation:
- Use
pandas
for advanced data manipulation. - Leverage Python’s
matplotlib
orseaborn
libraries to create visual reports. - Integrate Python scripts with task schedulers like Cron or Task Scheduler for recurring tasks.
2. Database Automation with Python
Databases are the backbone of most modern applications, storing everything from user data to transaction records. Python makes it easy to interact with databases and automate tasks like data insertion, updates, and retrieval.
Key Python Libraries for Database Management
sqlite3
: For lightweight database management.SQLAlchemy
: A powerful ORM (Object Relational Mapper).pyodbc
: For connecting to databases like MySQL, PostgreSQL, and Microsoft SQL Server.
Use Case: Automating Customer Data Updates
Let’s say you need to regularly update customer records in your database. With Python, you can write a script that reads updated data from a spreadsheet and applies it to your database.
Here’s how:
import sqlite3
import pandas as pd
# Connect to the database
conn = sqlite3.connect("customer_data.db")
cursor = conn.cursor()
# Read updated data from Excel
data = pd.read_excel("updated_customers.xlsx")
# Update records in the database
for index, row in data.iterrows():
cursor.execute("""
UPDATE customers
SET email = ?, phone = ?
WHERE customer_id = ?
""", (row['email'], row['phone'], row['customer_id']))
conn.commit()
conn.close()
print("Database updated successfully!")
Pro Tips for Database Automation:
- Use transaction management to ensure data consistency.
- Automate backups of your database using Python scripts.
- Combine database scripts with cloud storage tools to synchronize data across platforms.
3. Automating Online Forms with Python
Filling out online forms is another repetitive task that Python can automate. This is especially useful for tasks like submitting job applications, uploading product listings, or entering survey data.
Key Python Libraries for Form Automation
selenium
: For interacting with web browsers and automating form submissions.requests
: For sending HTTP requests to APIs or web forms.beautifulsoup4
: For web scraping, if needed.
Use Case: Automating Job Application Forms
Let’s say you’re applying to multiple job postings online and need to fill out similar forms repeatedly. A Python script using Selenium can handle this:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
# Set up the web driver
driver = webdriver.Chrome()
# Open the form page
driver.get("https://example-job-form.com")
# Fill out the form
driver.find_element(By.NAME, "name").send_keys("John Doe")
driver.find_element(By.NAME, "email").send_keys("johndoe@example.com")
driver.find_element(By.NAME, "resume").send_keys("/path/to/resume.pdf")
# Submit the form
driver.find_element(By.ID, "submit").click()
print("Form submitted successfully!")
driver.quit()
Pro Tips for Form Automation:
- Use browser extensions like ChromeDriver to automate Selenium scripts.
- Handle dynamic forms by waiting for elements to load using Selenium’s
WebDriverWait
. - Securely store sensitive data like passwords using environment variables or encrypted storage.
Advanced Tips: Automating Workflows with Python
When you combine Python’s flexibility and powerful libraries, you can create end-to-end automated workflows that save time, reduce errors, and streamline your processes. These workflows bring together multiple automation techniques into a seamless pipeline, handling everything from data extraction to report generation. Let’s explore this in detail.
Example Workflow: Automating End-to-End Data Processing
Step 1: Extract Data from an API or Email
Data often originates from external sources like APIs, emails, or web scraping. Python makes it easy to collect this data programmatically.
-
Extracting Data from APIs: APIs (Application Programming Interfaces) are a standard way to access data from services like weather apps, CRMs, or financial platforms. Python’s
requests
library simplifies this process.import requests # Example: Fetching weather data url = "https://api.openweathermap.org/data/2.5/weather" params = {"q": "Mumbai", "appid": "your_api_key"} response = requests.get(url, params=params) if response.status_code == 200: data = response.json() print(data) else: print("Failed to fetch data")
-
Extracting Data from Emails: Python’s
imaplib
orgmail-api
libraries allow you to access emails, search for specific content, and extract attachments.import imaplib import email # Connect to Gmail mail = imaplib.IMAP4_SSL("imap.gmail.com") mail.login("your_email@gmail.com", "your_password") # Select inbox and search emails mail.select("inbox") result, data = mail.search(None, "ALL") # Fetch email content for num in data[0].split(): result, msg_data = mail.fetch(num, "(RFC822)") msg = email.message_from_bytes(msg_data[0][1]) print(f"Subject: {msg['subject']}")
Step 2: Process and Clean the Data Using pandas
Once the raw data is extracted, it often needs cleaning and transformation to make it usable. This is where Python’s pandas
library comes into play.
-
Cleaning Data: Use
pandas
to handle missing values, remove duplicates, and standardize formats.import pandas as pd # Load data into a DataFrame df = pd.DataFrame([ {"Name": "Alice", "Age": 25, "Email": "alice@example.com"}, {"Name": "Bob", "Age": None, "Email": "bob@example.com"} ]) # Fill missing values df['Age'] = df['Age'].fillna(0) # Remove duplicates df = df.drop_duplicates() print(df)
-
Transforming Data: Convert data into a desired structure or format for easier analysis or storage.
# Add a new column df['Full Name'] = df['Name'] + " Doe" print(df)
Step 3: Store the Data in a Database Using sqlite3
Databases provide a structured way to store large amounts of data. Python’s built-in sqlite3
library lets you interact with a lightweight database without installing additional software.
-
Creating and Populating a Database: Store cleaned data from
pandas
into a SQLite database.import sqlite3 # Connect to SQLite database conn = sqlite3.connect("data.db") cursor = conn.cursor() # Create a table cursor.execute(""" CREATE TABLE IF NOT EXISTS users ( id INTEGER PRIMARY KEY, name TEXT, age INTEGER, email TEXT ) """) # Insert data users = [ (1, "Alice", 25, "alice@example.com"), (2, "Bob", 0, "bob@example.com") ] cursor.executemany("INSERT INTO users VALUES (?, ?, ?, ?)", users) conn.commit() conn.close() print("Data stored in the database successfully!")
Step 4: Generate Reports and Email Them Using smtplib
Once the data is processed and stored, you can generate reports or summaries. Python can then send these reports via email.
-
Generating Reports: Create summaries or visualizations using
pandas
ormatplotlib
.import matplotlib.pyplot as plt # Generate a bar chart df.plot(kind='bar', x='Name', y='Age', title="User Ages") plt.savefig("report.png")
-
Sending Emails: Use
smtplib
to send the generated reports as email attachments.import smtplib from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart from email.mime.base import MIMEBase from email import encoders # Set up email sender_email = "your_email@gmail.com" receiver_email = "recipient@example.com" password = "your_password" msg = MIMEMultipart() msg['From'] = sender_email msg['To'] = receiver_email msg['Subject'] = "Monthly Report" # Attach text msg.attach(MIMEText("Here is the monthly report.", 'plain')) # Attach file with open("report.png", "rb") as file: part = MIMEBase("application", "octet-stream") part.set_payload(file.read()) encoders.encode_base64(part) part.add_header("Content-Disposition", f"attachment; filename=report.png") msg.attach(part) # Send email with smtplib.SMTP_SSL("smtp.gmail.com", 465) as server: server.login(sender_email, password) server.sendmail(sender_email, receiver_email, msg.as_string()) print("Email sent successfully!")
Tools and Resources for Python Automation
-
Text Editors:
- Visual Studio Code: Lightweight, extensible, and perfect for Python scripting.
- PyCharm: Offers advanced features like debugging, code completion, and integrated version control.
-
Task Automation:
- Task Scheduler (Windows): Schedule scripts to run automatically at specific intervals.
- Cron Jobs (Linux/Mac): Automate recurring tasks by scheduling scripts.
-
Online Resources:
- ProgramGeeks: Community-driven platform with tutorials on Python for automation.
- GeeksForGeeks: Comprehensive articles and examples on Python libraries.
Why Choose Prateeksha Web Design for Python Automation Projects?
At Prateeksha Web Design, we specialize in developing Python automation solutions tailored to your business needs. Whether it’s automating spreadsheets, optimizing database workflows, or streamlining online form submissions, our team brings years of expertise to deliver robust and scalable solutions.
Conclusion
With Python, the possibilities for automating data entry tasks are virtually limitless. From simplifying repetitive tasks in spreadsheets to managing complex workflows across databases and forms, Python empowers you to work smarter, not harder. If you’re ready to transform how you handle data, dive into Python today and explore its endless capabilities. Or better yet, let Prateeksha Web Design guide you on this exciting journey.
About Prateeksha Web Design
- Python data automation services
- Spreadsheet automation solutions
- Database integration for automated data entry
- Form data automation using Python
- Streamlined data entry processes with Python
Interested in learning more? Contact us today.
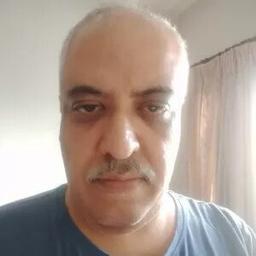