Seamlessly Integrating Shopify with Next.js
Incorporating Shopify into your Next.js setup is straightforward and powerful, allowing you to leverage Shopify's robust e-commerce functionalities while simultaneously customizing your storefront's user experience. Here’s how you can effectively integrate the two:
1. Setting Up Your Shopify Store
Before you can integrate Shopify with Next.js, you need to create your Shopify store. This involves:
- Creating an Account: Sign up for Shopify and select a plan that suits your needs.
- Adding Products: Upload product details, images, and other relevant data.
- Configuring Settings: Set up payment methods, shipping options, and tax rates to prepare your store for transactions.
2. Using Shopify Storefront API
Once your store is ready, you'll want to utilize Shopify’s Storefront API. This API allows your Next.js application to communicate with your Shopify store, enabling you to query products, collections, and even manage the cart experience.
- API Authentication: Generate a Storefront API token from your Shopify Admin dashboard.
- GraphQL Queries: Use GraphQL to make requests to the Storefront API for fetching product data, collections, and more. This is essential for ensuring your Next.js storefront dynamically displays the latest product information.
// Example: Fetching products from Shopify Storefront API using GraphQL
const fetchProducts = async () => {
const response = await fetch('https://your-shop-name.myshopify.com/api/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/graphql',
'X-Shopify-Storefront-Access-Token': 'your-access-token',
},
body: `
{
products(first: 10) {
edges {
node {
id
title
description
variants(first: 5) {
edges {
node {
id
title
price {
amount
currencyCode
}
}
}
}
images(first: 1) {
edges {
node {
originalSrc
altText
}
}
}
}
}
}
}
`,
});
const data = await response.json();
return data.data.products.edges;
};
3. Building Your Next.js Application
With the API set up, it's time to build your Next.js application:
- Directory Structure: Follow a clean and organized directory structure for your components, pages, and utilities.
- Dynamic Pages: Use Next.js's dynamic routes to create individual product pages that showcase your products. This creates a seamless browsing experience for users.
// Example: Dynamic route for product pages in Next.js
import { useRouter } from 'next/router';
const ProductPage = ({ product }) => {
const router = useRouter();
if (router.isFallback) {
return <div>Loading...</div>;
}
return (
<div>
<h1>{product.title}</h1>
<img src={product.images[0].originalSrc} alt={product.images[0].altText} />
<p>{product.description}</p>
<p>{product.variants[0].node.price.amount} {product.variants[0].node.price.currencyCode}</p>
</div>
);
};
4. Managing the Shopping Cart
You can create a custom shopping cart experience by utilizing React's state management:
- State Management: Use React's Context API or a state management library (like Redux) to handle the shopping cart state effectively.
- Cart Functionality: Implement functions to add, remove, and update items in the cart, ensuring a responsive and interactive experience.
5. Enhancing The User Experience
Leverage Next.js’s features to improve the overall shopping experience:
- Image Optimization: Use Next.js’s Image component to optimize and serve images in modern formats. This greatly enhances loading speed and user engagement.
- Code Splitting: Take advantage of automatic code splitting for better performance across different product pages, allowing for faster load times.
Conclusion: Unlocking the Future of E-commerce
By building a scalable e-commerce platform with Next.js and Shopify, you create a lightning-fast, SEO-optimized, and user-friendly online store. The combination of these technologies allows for a high degree of customization and an unmatched shopping experience for your customers.
As program geeks, you’re not just creating an e-commerce site; you’re crafting a seamless journey for your clients. Whether it’s improving site performance, enhancing user interaction, or utilizing headless commerce to bring innovative design to life, the possibilities are as vast as your creativity.
So, what are you waiting for? Start coding, innovate, and bring your e-commerce dreams to life with Next.js and Shopify today! Happy coding!
Integrating Shopify with Next.js can streamline your e-commerce operations, allowing you to harness the full potential of both platforms for a seamless shopping experience. Shopify acts as the robust backend, handling inventory management and transaction processing, while Next.js serves as the elegant frontend, delivering a fast and responsive user interface.
Let’s Break It Down
-
Set Up a Shopify Store: The first step is to establish your Shopify store. Begin by signing up for a Shopify account and configuring your store settings. Populate your store with products by adding detailed descriptions, pricing, and high-quality images. Think of this phase as setting up a well-organized kitchen before you begin cooking; having everything in place is crucial for smooth operations.
-
Connect to Shopify API: Integrate with the Shopify API to access your product data, collections, and other relevant assets. This API serves as a bridge between your Next.js frontend and the Shopify backend, allowing you to pull real-time data. Imagine sending a quick text message to a friend asking for help—it's that straightforward! By utilizing the API, you can ensure that your storefront reflects your latest offerings and inventory levels dynamically.
-
Building the Next.js Storefront: With your product data in hand, it's time to bring your vision to life by building your Next.js storefront. Utilize Next.js components to create visually appealing layouts that effectively showcase your product images. Don’t underestimate the power of compelling call-to-action buttons; they are your nudge to customers, guiding them toward making a purchase. Think of these elements as the garnish that enhances your final dish.
-
Dynamic Next.js Product Pages: Here comes the magic—creating dynamic product pages using Next.js’s routing capabilities. By setting up unique URLs for each product (such as “www.yoursite.com/products/product-name”), you make it easy for customers to access specific items. This approach not only improves SEO but also aids in navigation, ensuring users can find exactly what they’re looking for.
Performance Optimization in Next.js
After putting together your storefront's shiny components, the next crucial step is ensuring optimal performance. A fast-loading site is key to a positive user experience, so let’s dive into some strategies for Next.js performance optimization:
-
Image Optimization: Employ Next.js's
next/image
component to automatically optimize images on your site. This feature compresses images and serves them in next-gen formats, resulting in faster load times and a better user experience. Say goodbye to cumbersome, slow images that can deter potential customers! -
Static Generation: Utilize static generation for crucial pages such as your homepage and product pages during the build time. This technique pre-renders these pages, so they load almost instantly for users visiting your site—imagine having a well-prepared starter menu ready for guests, making for a delightful first impression.
-
Code Splitting: Next.js simplifies code management by automatically performing code splitting. This means that it serves only the necessary components for each page, minimizing load times. Picture it as serving just the right slice of pizza to an individual, rather than the entire pie, to streamline the experience.
-
Prefetching: Advance preparation can enhance user engagement; use Next.js's prefetching capabilities to load pages or data in advance based on user behavior. This preemptive loading is akin to offering freshly baked cookies to entice your visitors into exploring more.
Design Matters: The Aesthetics of E-commerce
While back-end functionality is critical, the front-end aesthetic cannot be overlooked. A compelling e-commerce platform offers not only seamless operations but also captivating visuals. Here’s how to enhance the design of your storefront:
-
Responsive Design: Ensure that your site is responsive and looks great on all devices, from desktops to mobile phones. Leverage Next.js’s built-in CSS support and media queries to create fluid layouts that adapt seamlessly. Nobody enjoys pinching and zooming on a mobile site!
-
Typography and Colors: The choice of fonts, colors, and overall design elements should resonate with your brand’s identity. Good visual design fosters brand recognition and builds trust. If you're unsure about your design choices, consider collaborating with a skilled web design company in Mumbai or your area to enhance your website's look.
-
User Experience (UX): Prioritize smooth user navigation. Design your site so that users can effortlessly find what they’re looking for, ensuring an intuitive journey. Keep the interface simple and uncluttered, guiding customers from landing on your site to completing their purchases as seamlessly as possible.
Conclusion
Integrating Shopify with Next.js is not only about linking two powerful platforms; it's about creating a cohesive ecosystem where backend capabilities meet frontend aesthetics. By following these detailed guidelines—from setting up your Shopify store and leveraging the API to optimizing performance and focusing on design—you can deliver an exceptional e-commerce experience that delights your customers and drives sales.
Collaborating with Expertise: Prateeksha Web Design
In today's fast-paced digital landscape, navigating the complexities of web development can often feel daunting. However, if you ever find yourself feeling overwhelmed by the myriad of tasks involved in launching an online presence, remember—you don't have to tackle this journey alone! Partnering with a reputable web design agency in Mumbai, like Prateeksha Web Design, can significantly ease the burden. Specializing in e-commerce website design in Mumbai, Prateeksha Web Design is dedicated to transforming your vision from good to extraordinary. Their skilled team of Mumbai website designers combines artistic creativity with technical prowess, ensuring that your site not only looks stunning but also functions flawlessly.
Launching Your E-commerce Site: The Moment of Truth
After numerous hours of planning, design, and development, the moment of truth arrives—the launch of your e-commerce website development in Mumbai project! Before you excitedly hit that enticing launch button, it's crucial to conduct a comprehensive final round of quality checks to ensure a seamless user experience. Here are some essential steps to consider:
-
Thorough Testing: It’s imperative to test every link, form, and interactive element on your site. Verify that all submit buttons function correctly and that users can navigate without encountering any bugs. A single broken link or form can lead to customer frustration and lost sales.
-
SEO Review: To maximize your visibility on search engines, conduct a meticulous review of your on-page SEO elements. Utilize powerful tools like Google Search Console and Moz to evaluate your keywords, meta tags, and overall site structure. Ensuring these aspects are in check will set a solid foundation for attracting organic traffic.
-
Social Media Integration: Drive initial traffic to your newly launched site by integrating it with your social media accounts. Create compelling posts that share intriguing aspects of your offerings, and utilize engaging visuals to attract users. Establishing a cohesive online presence across channels will amplify your reach and engagement.
Post-Launch Tactics: Sustaining Momentum
Congratulations! You’ve successfully launched your e-commerce website development in Mumbai project. However, this achievement is just the beginning. To ensure long-term success, it's essential to implement strategies that maintain momentum and enhance user experience:
-
Utilize Analytics: Leverage tools like Google Analytics to gain valuable insights into user behavior on your site. By observing patterns, you can make data-driven decisions that improve site functionality and cater to your target audience's preferences.
-
Feedback Loops: Cultivate a culture of feedback by encouraging customer reviews and promptly responding to inquiries. This not only helps in improving your offerings but also builds trust and promotes customer loyalty. Addressing concerns demonstrates that you value customer opinions and are committed to enhancing their experience.
-
Content Marketing: Establishing a blog on your site creates a platform for sharing valuable content that resonates with your audience. Regularly posting insightful articles will keep users engaged and informed, positioning your brand as an authority within your niche. Additionally, high-quality content can help improve your search engine rankings over time.
-
Regular Updates: Frequently updating your inventory and refreshing your website design is vital for retaining repeat customers. Ensure that your product offerings reflect current trends and consumer demands. A visually appealing and dynamically updated site enhances user experience, encouraging visitors to return.
Final Thoughts: Crafting Your E-commerce Masterpiece
Building a scalable e-commerce platform using technologies like Next.js and Shopify is akin to sculpting a masterpiece. With the right tools, resources, and reliable partners like Prateeksha Web Design, you can craft an unforgettable shopping experience that captivates your customers.
Remember, you hold the reins in shaping your e-commerce journey. Embrace the challenges, tap into your creativity, and dare to take that leap! Start constructing, exploring, and optimizing your platform—with the ultimate goal of transforming your unique vision into reality.
And should you ever find yourself facing roadblocks or uncertainties, rest assured that a community of experts and resources is available to support you. Don’t hesitate to reach out and ask questions—collaboration and sharing knowledge can propel you toward success!
Tip:
Leverage Static Site Generation: Utilize Next.js's Static Site Generation (SSG) features to build pages that load quickly and reduce server load. Pre-render product pages and use Incremental Static Regeneration (ISR) to update content automatically without a full rebuild, ensuring users always see the latest products.
Fact:
Headless CMS Flexibility: By integrating Shopify with Next.js, developers create a headless e-commerce setup that decouples the front end from the back end. This allows for greater flexibility in design and functionality, enabling developers to create customized user experiences while still taking advantage of Shopify's powerful commerce features.
Warning:
Monitor API Rate Limits: Be cautious of Shopify’s API rate limits when building your application. Excessive requests can lead to throttling, resulting in slower site performance or even downtime. Implement efficient data-fetching strategies such as caching and batching requests to avoid hitting these limits while ensuring a seamless user experience.
About Prateeksha Web Design
Prateeksha Web Design specializes in creating scalable e-commerce platforms by leveraging the powerful combination of Next.js and Shopify. Their services include custom design and development tailored to enhance user experience and optimize performance. With a focus on responsive layouts and fast loading times, they ensure seamless integration with Shopify's robust features. Prateeksha's team of experts employs best practices in coding and architecture to support rapid growth and scalability. Clients benefit from ongoing support and maintenance, driving long-term success in the competitive online marketplace.
Interested in learning more? Contact us today.
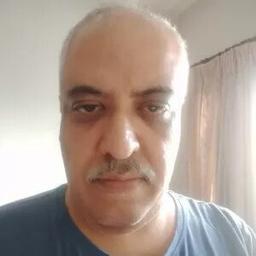