Introduction
Are you ready to dive into the world of mobile app development? If you’re new to coding or looking to expand your skills, React Native is a fantastic framework to explore. With its ability to build cross-platform apps efficiently, React Native has become a go-to solution for developers worldwide. Whether you’re a budding programmer or someone curious about app development, this React Native guide will set you on the right path.
At Prateeksha Web Design, we’ve worked on countless mobile app projects and witnessed firsthand the transformative power of React Native. In this blog, we’ll walk you through the essentials of getting started with React Native, share the best practices for beginners, and provide expert tips to boost your journey.
What is React Native?
React Native is a revolutionary framework that has reshaped mobile app development since its release by Facebook in 2015. Let’s break it down in detail to understand why it’s so popular and what makes it a game-changer.
Definition and Core Concept
React Native is an open-source framework that enables developers to build mobile applications using JavaScript and React. It’s unique because it lets you write code once and deploy it across multiple platforms, including iOS and Android, without needing to write separate native code for each platform.
At its heart, React Native is powered by React, a JavaScript library used for building user interfaces, but with the added capability to render to native platforms. Unlike traditional web development, where React renders to the browser, React Native translates the components to native UI elements, making your app feel and perform like a true native app.
How Does React Native Work?
React Native uses a "bridge" mechanism that connects JavaScript code to native modules. Here’s a simplified explanation:
- JavaScript Thread: The bulk of the app logic, including UI and state management, runs in JavaScript.
- Native Thread: Handles platform-specific code for rendering components and interacting with the hardware.
- Bridge: Acts as a communication channel between JavaScript and native threads, allowing data to flow back and forth.
This design ensures that React Native apps deliver a performance that closely mimics native apps while allowing developers to use the more accessible and versatile JavaScript language.
Key Features of React Native
1. Cross-Platform Development
React Native’s biggest selling point is its ability to develop apps for iOS and Android using a single codebase. This eliminates the need for separate development teams for each platform, reducing costs and development time.
2. Native Performance
By rendering UI elements using native components (e.g., UIView
for iOS and View
for Android), React Native ensures that the apps look and feel native. Unlike hybrid frameworks like Cordova, which rely on WebView, React Native delivers superior performance.
3. Hot Reloading
React Native includes a feature called Hot Reloading that allows developers to see changes in their code reflected immediately in the app, without the need for recompiling. This speeds up development and debugging, making the process more efficient.
4. Rich Ecosystem and Community
React Native boasts a massive developer community, meaning that resources, plugins, tutorials, and troubleshooting assistance are readily available. You can also use a wide array of pre-built libraries for functionality like navigation, animations, and state management.
5. Modular Architecture
The framework’s modular approach makes it easy to separate development work into distinct modules, simplifying collaboration and maintenance.
Why Choose React Native for Mobile App Development?
React Native’s popularity stems from its ability to bridge the gap between performance, cost-efficiency, and ease of development. Here’s why developers and businesses alike gravitate toward it:
1. Cross-Platform Capability
The "write once, run everywhere" approach eliminates redundant work. Developers can build one app and deploy it on both iOS and Android, ensuring consistent performance and appearance.
2. Cost-Effectiveness
With React Native, you don’t need to hire separate developers for iOS (Swift/Objective-C) and Android (Java/Kotlin). A single team of React Native developers can handle both platforms, cutting costs significantly.
3. Time-Saving
By sharing the same codebase for multiple platforms, developers can drastically reduce development and testing time, speeding up the time-to-market for apps.
4. Real-Time Development Feedback
The Hot Reloading feature not only improves developer productivity but also enhances learning for beginners by allowing them to see the effects of their code instantly.
5. Strong Community and Support
The active and vibrant React Native community is one of its greatest strengths. From comprehensive documentation to countless tutorials and third-party libraries, help is never far away.
6. Integration with Native Code
If your app requires platform-specific functionality that React Native doesn’t support out of the box, you can integrate native code into your React Native project. This hybrid approach provides the best of both worlds.
React Native vs. Other Frameworks
Feature | React Native | Flutter | Native Development |
---|---|---|---|
Language | JavaScript | Dart | Swift/Objective-C (iOS), Java/Kotlin (Android) |
Cross-Platform | Yes | Yes | No |
Performance | Near-Native | Near-Native | Native |
Learning Curve | Low | Medium | High |
Community Support | Extensive | Growing | Limited to each platform |
Who Uses React Native?
Many of the world’s top apps are built with React Native, including:
- Facebook: The creators of React Native use it extensively in their app.
- Instagram: React Native powers parts of the Instagram app.
- Uber Eats: Known for its smooth UI and performance, Uber Eats utilizes React Native.
- Tesla: Tesla’s mobile app is developed using React Native.
These success stories highlight the framework’s ability to handle apps of varying complexity and scale.
React Native Basics: Breaking Down the Building Blocks
Understanding how React Native apps are structured and how their fundamental components work is key to mastering the framework. Here’s an in-depth explanation of the core concepts that form the building blocks of React Native development.
1. Components
Components are the foundational elements of a React Native app. Think of them as Lego blocks that combine to build your app’s user interface (UI). A component in React Native can represent anything from a button to an entire screen.
Types of Components
- Functional Components: These are simple JavaScript functions that take in data (props) and return UI elements. They’re ideal for straightforward UIs without complex logic.
- Class Components: These are ES6 classes that extend
React.Component
. They’re used when you need to manage a component’s state or lifecycle methods.
Example: A Simple Functional Component
import React from 'react';
import { Text, View } from 'react-native';
const HelloWorld = () => {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
};
export default HelloWorld;
View
: A container for holding other components, similar to adiv
in HTML.Text
: Used to display text content.
Tip: Functional components are more commonly used today due to the introduction of React Hooks, which simplify state and lifecycle management.
2. Styling
Styling in React Native is inspired by CSS, but instead of writing CSS in a separate file, you define styles as JavaScript objects. This approach makes styles easier to manage and integrate directly into components.
Inline Styling Example
import React from 'react';
import { Text, View } from 'react-native';
const StyledComponent = () => {
return (
<View style={{ backgroundColor: 'lightblue', padding: 20 }}>
<Text style={{ color: 'white', fontSize: 18 }}>Hello, Stylish World!</Text>
</View>
);
};
export default StyledComponent;
Using StyleSheet
React Native’s StyleSheet
API allows you to define and reuse styles efficiently.
import React from 'react';
import { Text, View, StyleSheet } from 'react-native';
const StyledComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Styled with StyleSheet!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
backgroundColor: 'lightblue',
padding: 20,
},
text: {
color: 'white',
fontSize: 18,
},
});
export default StyledComponent;
3. Props and State
Props
Props (short for “properties”) are used to pass data from a parent component to a child component. Props are read-only, meaning the child component cannot modify them.
Example: Using Props
const Greeting = (props) => {
return <Text>Hello, {props.name}!</Text>;
};
<Greeting name="John" />;
State
State is used to manage dynamic data within a component. Unlike props, state can be updated.
Example: Managing State
import React, { useState } from 'react';
import { Text, Button, View } from 'react-native';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<View>
<Text>Count: {count}</Text>
<Button title="Increase" onPress={() => setCount(count + 1)} />
</View>
);
};
export default Counter;
4. Navigation
Navigation is an essential part of building mobile apps. React Native doesn’t have built-in navigation, but it provides excellent third-party libraries like React Navigation.
Getting Started with React Navigation
- Install the library:
npm install @react-navigation/native
- Install additional dependencies:
npm install react-native-screens react-native-safe-area-context react-native-gesture-handler react-native-reanimated
- Set up your app’s navigation structure:
import React from 'react'; import { NavigationContainer } from '@react-navigation/native'; import { createStackNavigator } from '@react-navigation/stack'; const Stack = createStackNavigator(); const HomeScreen = () => <Text>Welcome Home!</Text>; const App = () => { return ( <NavigationContainer> <Stack.Navigator> <Stack.Screen name="Home" component={HomeScreen} /> </Stack.Navigator> </NavigationContainer> ); }; export default App;
Best Practices for Beginners in React Native
1. Start Small and Build Gradually
Starting with simple projects, like a to-do app or a calculator, helps you build confidence and understand the basics.
2. Use Expo for Rapid Development
Expo simplifies the setup process, making it easier to start coding. It’s perfect for beginners but allows for more advanced setups as you progress.
3. Follow the DRY Principle
Don’t Repeat Yourself—reuse components and styles to keep your codebase clean and maintainable.
4. Learn Debugging Tools
React Native provides tools to help you debug efficiently:
- React Developer Tools: Inspect your app’s React component hierarchy.
- Flipper: A debugging platform for React Native with features like crash reporting and performance monitoring.
5. Focus on Performance Optimization
- FlatList and SectionList: Use these components for rendering long lists efficiently.
- Memoization: Prevent unnecessary re-renders using
React.memo
anduseMemo
.
6. Test on Real Devices
While emulators are useful, testing on real devices ensures your app performs well in real-world conditions.
Advanced Tips to Level Up Your Skills
As you grow more confident in React Native, diving into advanced practices will help you tackle larger projects, write more efficient code, and deliver a better user experience. Here’s a detailed explanation of advanced tips to take your skills to the next level.
1. State Management
Managing state becomes more complex as your app grows, especially when multiple components need access to shared data. React Native offers several solutions to manage state effectively:
Using Context API
The Context API is a built-in React feature that provides a simple way to manage state globally without needing external libraries. It’s best for small to medium-sized apps.
Example: Context API for Theme Management
import React, { createContext, useState, useContext } from 'react';
// Create Context
const ThemeContext = createContext();
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
};
// Use Context
const ThemeSwitcher = () => {
const { theme, setTheme } = useContext(ThemeContext);
return (
<Button
title={`Switch to ${theme === 'light' ? 'dark' : 'light'} mode`}
onPress={() => setTheme(theme === 'light' ? 'dark' : 'light')}
/>
);
};
Using Redux
Redux is a powerful library for managing complex application states. It centralizes your app’s state, making it predictable and easier to debug.
Why Choose Redux?
- Centralized state management.
- Middleware like Redux Thunk for handling asynchronous logic.
Basic Example
npm install redux react-redux
import { createStore } from 'redux';
import { Provider } from 'react-redux';
// Reducer
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
default:
return state;
}
};
// Store
const store = createStore(counterReducer);
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
2. Code Splitting
Large apps benefit greatly from breaking down code into smaller modules. This approach, known as code splitting, enhances maintainability and reduces app load times.
Using Dynamic Imports with React.lazy
React.lazy allows you to load components dynamically, improving performance.
Example:
import React, { Suspense } from 'react';
const HeavyComponent = React.lazy(() => import('./HeavyComponent'));
const App = () => (
<Suspense fallback={<Text>Loading...</Text>}>
<HeavyComponent />
</Suspense>
);
This ensures the HeavyComponent
is loaded only when needed, reducing the initial app load time.
3. Use TypeScript
TypeScript is a typed superset of JavaScript that improves code reliability by catching errors during development.
Why Use TypeScript in React Native?
- Static typing prevents runtime errors.
- Better documentation through type annotations.
- Enhanced IDE support with autocompletion and type hints.
Getting Started with TypeScript
- Install TypeScript:
npm install --save-dev typescript
- Rename files from
.js
to.tsx
.
Example with Type Annotations
interface Props {
name: string;
age: number;
}
const Greeting: React.FC<Props> = ({ name, age }) => {
return <Text>Hello, {name}! You are {age} years old.</Text>;
};
4. Leverage Native Modules
React Native provides built-in modules for many common tasks, but sometimes your app requires platform-specific features, such as using native APIs or accessing device hardware. In such cases, creating custom native modules is the solution.
Example: Bridging a Native Module
- Write the native code (Java/Swift) for the functionality.
- Expose the native code to JavaScript via a bridge.
Example: iOS (Swift)
@objc(MyNativeModule)
class MyNativeModule: NSObject {
@objc
func greet(_ name: String, callback: RCTResponseSenderBlock) {
callback(["Hello, \(name)"])
}
}
Using in React Native
import { NativeModules } from 'react-native';
const { MyNativeModule } = NativeModules;
MyNativeModule.greet('React Native', (message) => {
console.log(message); // Output: Hello, React Native
});
Common Challenges and How to Overcome Them
Every developer faces roadblocks, especially when working with a framework as flexible as React Native. Here’s how to tackle common challenges:
1. App Crashes
Problem
React Native apps may crash due to unhandled errors, bad API calls, or incorrect configurations.
Solution
- Use tools like Sentry or BugSnag to track and debug errors.
- Wrap components with
ErrorBoundary
for catching errors in the UI tree.class ErrorBoundary extends React.Component { state = { hasError: false }; static getDerivedStateFromError() { return { hasError: true }; } render() { if (this.state.hasError) { return <Text>Something went wrong.</Text>; } return this.props.children; } }
2. Dependency Issues
Problem
The vast React Native ecosystem often leads to conflicts between libraries or versions.
Solution
- Use Yarn over npm for consistent dependency management:
yarn install
- Check for version mismatches and update dependencies:
npx react-native upgrade
3. Learning Curve
Problem
Beginners often feel overwhelmed by the number of concepts, tools, and best practices to learn.
Solution
- Focus on learning React before diving into React Native.
- Build small, functional apps to practice core concepts like components, props, and state.
- Join React Native communities on GitHub, Reddit, or Discord for support and resources.
Why Choose Prateeksha Web Design for Your React Native Projects?
At Prateeksha Web Design, we’re passionate about creating seamless mobile app experiences. Here’s what sets us apart:
- Expertise in React Native: With years of experience, we deliver high-quality, scalable apps.
- Focus on Beginners: Our team loves mentoring and guiding budding developers.
- End-to-End Solutions: From ideation to deployment, we handle it all.
Our mission is to empower individuals and businesses with cutting-edge app development solutions. Whether you’re looking for professional guidance or a partner to build your next app, Prateeksha Web Design has got your back!
Conclusion
Embarking on your React Native journey is exciting and rewarding. With this React Native guide, you now have the tools and knowledge to start building amazing apps. Remember, every expert was once a beginner, and the key is consistent learning and practice.
React Native makes mobile app development accessible to everyone, and with best practices in place, you’re already on the path to success. If you’re looking for expert advice or want to collaborate on a project, don’t hesitate to reach out to Prateeksha Web Design. Let’s build something incredible together!
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services for beginners looking to get started with React Native. Their best practices guide includes hands-on tutorials, coding standards, and performance optimization tips. They focus on creating responsive mobile applications, providing essential resources for UI components and navigation. Tailored mentorship ensures newcomers gain confidence and proficiency in React Native development. With a supportive community, learners can share insights and troubleshoot challenges effectively.
Interested in learning more? Contact us today.
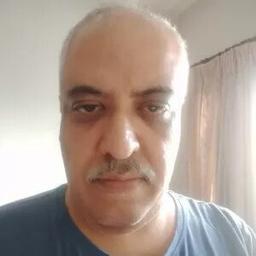