Data validation is a critical step in building robust applications. Whether you’re developing APIs, handling user inputs, or processing data files, ensuring the integrity of the data can prevent bugs and unexpected behavior. Python offers many libraries for this purpose, but Pydantic has emerged as one of the most popular and effective tools for data validation. This guide will explore Pydantic in-depth, providing examples, usage tips, and best practices for scenarios ranging from validating file paths to using custom validators.
What is Pydantic?
Pydantic is a Python library designed for data parsing and validation, leveraging Python’s type hints to define the structure and rules of your data. Unlike traditional approaches that involve manually writing validation code, Pydantic automates much of the validation process, providing a cleaner, more reliable way to work with structured data.
At its core, Pydantic uses Python’s built-in data types (like str
, int
, list
, etc.) to create models that describe how your data should look. When you pass data to a Pydantic model, the library automatically validates it against the defined types and raises errors if it doesn’t conform. This ensures that the data your application processes is accurate and complete, preventing potential bugs or unexpected behavior.
Why Pydantic Stands Out
Pydantic combines type hinting and validation seamlessly, enabling developers to write clean and maintainable code. By integrating validation directly into the definition of the data structure, Pydantic eliminates the need for repetitive and error-prone manual checks.
Key Features of Pydantic
1. Automatic Data Validation
When you create a Pydantic model, it automatically validates the data passed to it at instantiation. This means you don’t need to write explicit validation logic for each field. If the input doesn’t match the expected type or constraints, Pydantic raises a ValidationError.
Example:
from pydantic import BaseModel
class User(BaseModel):
name: str
age: int
# Valid input
user = User(name="Alice", age=25)
# Invalid input raises an error
user = User(name="Alice", age="twenty-five")
Output:
ValidationError:
1 validation error for User
age
value is not a valid integer (type=type_error.integer)
2. Type Coercion
Pydantic doesn’t just validate—it also coerces compatible types into the expected types when possible. For example, if a field is defined as int
but receives a string representation of a number, Pydantic will convert it automatically.
Example:
class Product(BaseModel):
id: int
product = Product(id="123") # Pydantic converts the string "123" to an integer
print(product.id) # Output: 123
This feature is especially useful for handling data from external sources, where the format might not always match your expectations perfectly.
3. Custom Validation
With Pydantic, you can define your own validation logic for fields using custom validators. These allow you to enforce more complex rules beyond basic type checks.
Example:
from pydantic import BaseModel, validator
class User(BaseModel):
name: str
age: int
@validator('age')
def check_age(cls, value):
if value < 18:
raise ValueError("Age must be at least 18.")
return value
# Invalid input raises an error
try:
user = User(name="Alice", age=16)
except ValueError as e:
print(e)
4. Nested Models
Pydantic makes it easy to work with nested data structures by supporting models within models. This is particularly useful when working with hierarchical data like JSON objects.
Example:
class Address(BaseModel):
street: str
city: str
class User(BaseModel):
name: str
address: Address
user = User(name="Alice", address={"street": "123 Main St", "city": "Springfield"})
print(user)
Output:
name='Alice' address=Address(street='123 Main St', city='Springfield')
5. JSON Serialization
Pydantic provides built-in methods to serialize models to JSON and back. This is incredibly useful for APIs and systems that rely on JSON for data exchange.
Example:
user = User(name="Alice", address={"street": "123 Main St", "city": "Springfield"})
print(user.json()) # Output: '{"name": "Alice", "address": {"street": "123 Main St", "city": "Springfield"}}'
6. Integration with FastAPI
One of Pydantic’s most popular use cases is its integration with FastAPI, a Python web framework. FastAPI uses Pydantic models to automatically validate request bodies, query parameters, and more, making it easier to build robust APIs with minimal effort.
Example:
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class User(BaseModel):
name: str
age: int
@app.post("/users/")
async def create_user(user: User):
return {"message": f"User {user.name} added successfully!"}
In this example, FastAPI uses Pydantic to validate the incoming JSON payload against the User
model, ensuring the data is correct before it’s processed.
Setting Up Pydantic
Before diving into usage, you need to install Pydantic. Run the following command:
pip install pydantic
Getting Started with Pydantic Models
Pydantic’s BaseModel is the foundation for creating data models. Let’s start with a simple example:
Example: Required String and Integer Validation
from pydantic import BaseModel
class User(BaseModel):
name: str
age: int
# Valid input
user = User(name="John Doe", age=30)
print(user)
# Invalid input raises validation error
try:
user = User(name="John Doe", age="thirty")
except ValueError as e:
print(e)
Output:
name='John Doe' age=30
1 validation error for User
age
value is not a valid integer (type=type_error.integer)
Advanced Field Validation with Pydantic
1. Custom Validators
Custom validation can be achieved using the @validator
decorator.
Example: Validating an Email Address
from pydantic import BaseModel, EmailStr, validator
class User(BaseModel):
name: str
email: EmailStr
@validator('name')
def name_must_not_be_empty(cls, value):
if not value.strip():
raise ValueError("Name cannot be empty.")
return value
# Valid input
user = User(name="Jane Doe", email="jane.doe@example.com")
print(user)
# Invalid input raises validation error
try:
user = User(name=" ", email="jane.doe@example.com")
except ValueError as e:
print(e)
2. File Path Validation
Pydantic offers a FilePath
type for validating file paths.
Example: Validating a File Path
from pydantic import BaseModel, FilePath
class Config(BaseModel):
filepath: FilePath
# Valid file path
try:
config = Config(filepath="valid_file.txt") # Replace with an actual valid file path
print(config)
except ValueError as e:
print(e)
# Invalid file path
try:
config = Config(filepath="invalid_file.txt")
except ValueError as e:
print(e)
3. Annotated Field Validation
Using Annotated
allows you to enhance the readability of field validation.
Example: Annotated File Path Validation
from typing import Annotated
from pydantic import BaseModel, FilePath
class Config(BaseModel):
filepath: Annotated[str, FilePath]
# Example usage
config = Config(filepath="valid_file.txt") # Replace with a valid path
Nested Models and Complex Data Structures
Pydantic supports nested models, making it easy to work with hierarchical data.
Example: List of Dictionaries and Nested Models
from pydantic import BaseModel
from typing import List
class Address(BaseModel):
city: str
zipcode: str
class User(BaseModel):
name: str
addresses: List[Address]
# Valid input
user = User(
name="John Doe",
addresses=[{"city": "New York", "zipcode": "10001"}, {"city": "Boston", "zipcode": "02108"}]
)
print(user)
Working with FastAPI and Pydantic
FastAPI leverages Pydantic models for request validation, making it effortless to build robust APIs.
Example: FastAPI Validation
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class User(BaseModel):
name: str
age: int
@app.post("/users/")
async def create_user(user: User):
return {"name": user.name, "age": user.age}
With this setup, FastAPI automatically validates incoming requests against the User
model.
Best Practices for Data Validation with Pydantic
- Use Type Hints Effectively: Leverage Python's type hints for clarity and precision.
- Combine Built-in Validators: Use Pydantic's built-in types like
EmailStr
,FilePath
, etc. - Define Custom Validators: For specific business logic, use
@validator
or@root_validator
. - Test Your Models: Write test cases to validate your Pydantic models.
Advanced Features
1. Asynchronous Validation
Pydantic supports asynchronous validators using @validator(..., pre=True)
.
from pydantic import BaseModel, validator
class AsyncModel(BaseModel):
value: int
@validator('value', pre=True)
async def async_validator(cls, value):
if value < 0:
raise ValueError("Value must be positive.")
return value
2. Schema and JSON Serialization
Pydantic models can be serialized to JSON and schemas.
Example: JSON Serialization
user = User(name="John Doe", age=30)
print(user.json())
Example: Schema Generation
print(User.schema_json())
3. Dataclasses Integration
Pydantic supports dataclasses with the @pydantic.dataclasses.dataclass
decorator.
from pydantic.dataclasses import dataclass
@dataclass
class Product:
name: str
price: float
product = Product(name="Laptop", price=1200.99)
print(product)
4. Troubleshooting Common Issues
- Validation Errors: Check error messages for type mismatches or missing required fields.
- Nested Model Errors: Ensure nested models are properly defined and instantiated.
Conclusion
Pydantic is a powerful tool for data validation in Python. From validating simple fields like strings and integers to handling complex data structures, Pydantic simplifies the process with its robust feature set. Integrating Pydantic into your projects, especially with FastAPI, can significantly enhance the reliability and maintainability of your applications.
This guide explored core and advanced features of Pydantic, including custom validators, field validation, and best practices. Whether you're building APIs, validating file paths, or working with nested models, Pydantic makes data validation both easy and Pythonic.
About Prateeksha Web Design
Prateeksha Web Design is a premier web design and development agency dedicated to crafting innovative and custom solutions tailored to meet the unique needs of businesses across industries. Based on a foundation of creativity, technical expertise, and a customer-first approach, we pride ourselves on delivering websites that are not just visually appealing but also highly functional, user-friendly, and designed to drive results.
Our Mission
At Prateeksha Web Design, our mission is to empower businesses, both large and small, by helping them establish a strong online presence. We aim to bridge the gap between technology and creativity, enabling our clients to reach their target audience effectively and achieve their digital goals.
Interested in learning more? Contact us today | Pydantic Documentation | FastAPI Documentation.
FAQs
-
What is Pydantic and what are its main features? Pydantic is a Python library for data parsing and validation that utilizes Python’s type hints. Its main features include automatic data validation, type coercion, custom validation, support for nested models, and built-in JSON serialization.
-
How does Pydantic handle data validation? Pydantic automatically validates data upon model instantiation based on the defined types and constraints. If the data does not conform, it raises a
ValidationError
. -
Can I define custom validation rules with Pydantic? Yes, Pydantic allows the creation of custom validation logic using the
@validator
decorator. This enables developers to enforce more complex rules beyond basic type checks. -
Is Pydantic compatible with FastAPI? Yes, Pydantic integrates seamlessly with FastAPI, automatically validating request data against Pydantic models, thus streamlining the process of building robust APIs.
-
What are some best practices for using Pydantic? Some best practices include using type hints effectively, combining built-in validators, defining custom validators for specific logic, and thoroughly testing Pydantic models to ensure reliability and correctness.
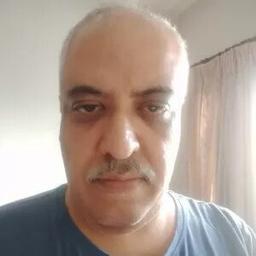