Welcome, fellow developers and tech enthusiasts! If you're ready to embark on the journey of Building Scalable Multi-Tenant SaaS Applications with Laravel, you’re in for a treat. Prepare your favorite beverage, find your most comfortable seat, and let’s dive into this exciting and technical adventure. This journey promises a rich experience filled with knowledge, insights, and a sprinkle of coding magic!
Understanding Multi-Tenant SaaS Applications
Before we dive into the nitty-gritty of coding, it's crucial to have a clear understanding of what a multi-tenant SaaS application actually is. Picture a multi-tenant architecture as a modern apartment complex. Each tenant (user) possesses their own familiar space (data and configurations), while they all share the same infrastructure (server resources and codebase). This approach is immensely efficient, allowing providers to optimize resource usage and maintain cost-effectiveness.
In simpler terms, a multi-tenant application allows multiple organizations or users to share the same software instance while keeping their data and settings distinct from one another. This approach yields significant advantages, including lowered operational costs, efficient scaling possibilities, and simplified management.
Key Takeaway
Multi-tenant architecture streamlines resource management and reduces costs by enabling multiple users to operate within a shared infrastructure while ensuring the integrity and confidentiality of their individual data.
Why Choose Laravel for Your SaaS Adventure?
Now, you may be asking, "Why are we choosing Laravel for our venture?" Laravel stands out as an exceptional PHP framework, akin to having a versatile Swiss Army knife at your disposal. Its elegant syntax not only facilitates development but makes it a joy to work with.
Laravel is revered in the developer community, boasting a wealth of features that cater specifically to SaaS app development:
- Built-in User Authentication: Safeguard your application with Laravel's robust authentication features right out of the box.
- Expressive and Intuitive ORM (Eloquent): Efficiently manage database interactions with Eloquent, making data handling seamless.
- Database Migrations: Version control for your database schema allows for smooth transitions and updates.
Additionally, Laravel’s extensive community and rich ecosystem of packages simplify many development tasks, making it easier to maintain and scale your application.
Key Takeaway
Laravel is ideally suited for SaaS development due to its effective resource management, user-friendly syntax, mature ecosystem, and a rich set of functionalities that enhance application efficiency and maintainability.
Setting Up Multi-Tenancy in Laravel
Let's get hands-on! Implementing multi-tenancy in Laravel can be approached in several ways, each having its strengths and weaknesses. Here's a closer look at three common multi-tenancy strategies:
-
Single Database, Shared Schema:
- In this configuration, all tenant data resides in the same database tables, identified with a
tenant_id
column. This is simpler and more straightforward, making it easier to manage, but as the number of tenants increases, performance could potentially lag.
- In this configuration, all tenant data resides in the same database tables, identified with a
-
Single Database, Separate Schemas:
- Each tenant is allocated their own schema within a single database. This configuration promotes better organization of data and provides an added layer of data separation, facilitating maintenance.
-
Multiple Databases:
- In this structure, every tenant is assigned a unique database. While this promotes the highest level of separation and security, it can also present more management challenges and complexities in scaling.
Key Takeaway
Selecting the appropriate multi-tenancy strategy hinges on your application's specific needs and your anticipated growth trajectory. Consider scalability requirements, maintenance challenges, and cost implications when making your choice.
Implementing Multi-Tenancy with Laravel
Now that you've grasped the foundation, let's dive into implementation. First and foremost, you need to set up your Laravel project. You can create a new Laravel application using the following command:
composer create-project --prefer-dist laravel/laravel your-app-name
After successfully initializing your project, it's time to enhance your application with multi-tenancy capabilities by incorporating specialized packages. A popular and reliable option is the “Tenancy for Laravel” package. Installation is easy with Composer:
composer require stancl/tenancy
After installing the package, follow the documentation provided to set up multi-tenancy in your application. This involves configuring your database connections and implementing necessary middleware for routing tenant requests.
Configuration Steps
- Publish Configuration Files: Publish the package’s configuration files to customize the multi-tenancy behavior.
php artisan vendor:publish --provider="Stancl\Tenancy\TenancyServiceProvider"
- Define Routes and Model: Set up Tenant and Website models according to your tenancy strategy.
- Middleware Setup: Implement middleware to handle tenant identification from the incoming request.
Key Takeaway
Implementing multi-tenancy in Laravel involves both installation of necessary packages and careful configuration of your application to ensure seamless routing and data management for multiple tenants.
With this foundational understanding and initial setup, you are well on your way to developing a scalable and efficient multi-tenant SaaS application using Laravel. Keep exploring, experimenting, and most importantly, have fun while you code!
After Installation: Publishing Configuration
Once you have successfully installed the necessary packages for your multi-tenant application in Laravel, the next crucial step is to publish the configuration files. This step is vital as it allows you to customize the settings specific to your project's requirements. To do this, execute the following command in your terminal:
php artisan vendor:publish --provider="Stancl\Tenancy\TenancyServiceProvider"
Upon executing this command, you will be guided through a series of configuration options. This is an opportunity to contemplate how you want to structure your tenants and their configurations:
-
Which method aligns best with your project?: Multi-tenancy can be implemented in various ways: using a single database with shared schemas, multiple databases, or even multiple schemas within a single database. Each method has its pros and cons, depending on scalability, data isolation, and maintenance.
-
How will you structure your data?: Consider how tenant data will be organized. For instance, if you opt for a shared schema, you'll need to ensure that tenant-specific data can be easily distinguished and queried efficiently.
Key Takeaway: Leverage the "Tenancy for Laravel" package to streamline the setup process for your multi-tenant application, making it adaptable to your chosen architecture.
Laravel Database Partitioning: Enhancing Performance
When we talk about Laravel database partitioning, we are addressing a fundamental architectural practice that optimizes the performance and maintainability of your application. To illustrate, think of partitioning in the same way you would organize a closet—by categorizing clothes by type or season, you can quickly find what you need. Translating this to databases, partitioning allows for systematic data organization that can accelerate query performance and ease data retrieval for different tenants.
Example:
If you choose the single database, shared schema method, you can significantly enhance the performance of your queries by creating indexes on frequently queried columns, such as tenant_id
. This index allows the database to locate and retrieve data faster.
Additionally, many cloud providers, such as AWS and Google Cloud, provide advanced database management solutions that not only support partitioning but also enable you to scale your databases seamlessly as the number of tenants grows.
Key Takeaway: Efficiently implementing database partitioning is crucial for improving the performance and manageability of your multi-tenant application, helping it operate smoothly as demand increases.
Queue Management in Laravel: Ensuring Smooth Operations
In a multi-tenant environment, you might encounter scenarios where several tenants are making requests simultaneously. Instead of panicking, you can harness the power of Laravel's queue management to streamline these processes effectively. Picture a bustling café where a skilled barista skillfully manages incoming orders—recording them and handling them in the sequence they are received. Laravel does just that for your application.
With Laravel, you have various queue drivers at your disposal, such as database, Redis, and Beanstalkd. Here’s a straightforward way to set up queue management with the database driver:
php artisan queue:table
php artisan migrate
Once you've run these commands, you're all set to dispatch jobs to the queue. This mechanism ensures that each tenant’s requests are processed in an orderly manner without causing bottlenecks or delays, keeping the user experience seamless.
Key Takeaway: Utilize Laravel's queue management features to prioritize and efficiently process various tasks, ensuring that tenant requests do not conflict and result in slowdowns.
The Power of Laravel Microservices: Embracing Scalability
As your Software as a Service (SaaS) application continues to expand, you may find it beneficial to embrace Laravel microservices architecture. This approach resembles having multiple dishwashers in a busy kitchen—each one is capable of performing its specific tasks independently, contributing to overall efficiency without hindering the work of others.
Adopting a microservices architecture allows you to decompose your application into smaller, more manageable services that can communicate and function together effortlessly. Each microservice can be developed, deployed, and scaled independently, which enhances maintainability and can lead to faster feature development and deployment cycles. This flexibility is particularly advantageous in a multi-tenant context, as it allows for tailored services catering to different tenant needs without disrupting the entire application.
Key Takeaway: Transitioning to a microservices architecture can significantly enhance the scalability and maintainability of your Laravel application, allowing for better resource allocation and development speed as your user base grows.
Sure! Let’s expand, explain in detail, and refine your content on creating a multi-tenant SaaS (Software as a Service) application using Laravel.
Modular Design for Multi-Tenant SaaS Applications
In today’s fast-paced digital landscape, having a well-architected application is crucial for success. One effective approach is to use microservices within your Laravel framework. Here are some essential services you should consider:
-
Authentication Service:
- This service is responsible for managing user access and roles across all tenants.
- It ensures secure logins, handles user registration, account recovery, and manages roles and permissions.
- By centralizing authentication, you streamline user management, making it easier to enforce consistent security policies across different tenants.
-
Billing Service:
- This is the backbone of your SaaS application, managing subscriptions, billing cycles, and payment processing.
- It should handle various payment methods, recurring billing, and invoicing while providing a seamless user experience.
- An effective billing service also entails managing discounts, promotions, and refunds, ensuring that your tenants are billed accurately for their usage.
-
Notification Service:
- This service ensures that your tenants are informed about relevant events through email or push notifications.
- Notifications could include alerts about billing cycles, new feature releases, or important updates regarding their accounts.
- A well-structured notification service enhances user engagement and keeps tenants informed, which can improve customer satisfaction.
By leveraging Laravel’s API resources, each of these services can communicate efficiently and effectively. This modular design not only aids in scalability but also simplifies code maintenance. As you evolve your application, you can develop or modify services independently without the risk of impacting the entire system.
Key Takeaway:
Adopt a microservices architecture to enhance the scalability and maintainability of your Laravel SaaS application.
Best Practices for Developing Laravel SaaS Applications
Now that you understand the foundational services for your application, let’s explore some essential best practices that are critical to the development of a robust multi-tenant SaaS application:
-
Environment Configuration:
- Utilize environment variables for managing sensitive data, such as API keys and database credentials.
- Laravel’s
.env
file provides a secure way to handle these configurations, ensuring that sensitive information is not hard-coded within your codebase.
-
Version Control:
- Implement a version control system such as Git. This practice not only tracks changes and allows you to manage project versions effectively but also serves as a backup for your codebase.
- Regular commits and branching strategies can significantly streamline development and collaboration among team members.
-
Robust Testing:
- Writing comprehensive tests is crucial to maintaining the integrity of your application.
- With Laravel's built-in testing tools, you can easily create unit tests and feature tests to validate functionality, ensuring that your application behaves as expected.
- Regular testing can save you from facing critical bugs in production.
-
User Analytics and Onboarding:
- Integrating user analytics allows you to gather insights into tenant behavior and preferences, enabling you to tailor your offerings effectively.
- A smooth onboarding process is equally important, guiding users through your application's features effortlessly and enhancing user satisfaction.
-
Security First:
- Prioritize security by utilizing Laravel’s built-in features such as Cross-Site Request Forgery (CSRF) protection, SQL injection prevention, and secure password hashing.
- Regularly update dependencies and apply security patches to further bolster your application’s defenses.
Key Takeaway:
Implementing these best practices ensures that your SaaS application is secure, scalable, and easier to maintain.
Partnering with the Best in Design and Development
As you embark on developing your eCommerce website or any web project, consider partnering with a reputable web design company in Mumbai, such as Prateeksha Web Design. Their specialization in creating seamless user experiences not only enhances visual appeal but can also significantly impact the overall success of your SaaS application.
With their expertise in eCommerce website development, you can ensure that your application distinguishes itself from the competition by delivering a user-friendly, intuitive design that meets the needs of your target audience.
Key Takeaway:
Collaborate with top-notch web design professionals to ensure your SaaS application stands out in terms of visual appeal and user experience.
Conclusion: Take the Leap!
Building a scalable multi-tenant SaaS application with Laravel may initially seem overwhelming, but with the right approach, mindset, and tools, you have the potential to create remarkable software.
Embrace the coding journey—experiment, innovate, and remember to enjoy the process.
The path to creating your groundbreaking application starts with that first line of code. So, let’s get started! Who knows? Your application could be the next big success in the SaaS industry!
Tip:
Optimize Database Design: When building a multi-tenant SaaS application, consider using a shared database with tenant identifiers for each table or a partitioned database approach. Ensure that your database schema is designed to efficiently handle queries with tenant-specific filtering to minimize data retrieval times.
Fact:
Multi-Tenancy Models: There are three primary models of multi-tenancy in SaaS applications: Shared Database, Shared Schema; Shared Database, Separate Schema; and Separate Databases per Tenant. Each model comes with its own trade-offs in terms of complexity, resource utilization, and tenant isolation. Understanding these models is crucial for making informed architectural decisions.
Warning:
Data Security Risks: Multi-tenancy increases the risk of data leakage between tenants if not implemented correctly. Learn about and apply proper data access controls, encryption techniques, and regular security audits to prevent unauthorized access and ensure tenant data isolation. Failing to secure the application adequately can lead to severe legal and financial consequences.
About Prateeksha Web Design
Prateeksha Web Design specializes in crafting scalable multi-tenant SaaS applications using Laravel, ensuring robust architecture and seamless user experiences. Their services include custom design and development, database optimization, and efficient tenant management systems. They focus on responsive design and integration with third-party APIs for enhanced functionality. Additionally, the team offers ongoing support and maintenance to ensure the application scales smoothly with growing user demands. Trust Prateeksha to deliver secure, high-performance SaaS solutions tailored to your business needs.
Interested in learning more? Contact us today.
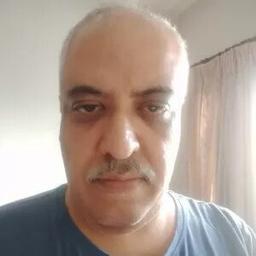