Building A Real-Time Mobile App: A Program Geeks’ Guide To Firebase And React Native
Hello, aspiring app developers! 🖖 Have you ever dreamt of creating your very own real-time mobile application, only to be overwhelmed by the myriad of technologies involved? Fear not! Today, we’ll demystify the process using the powerful combination of React Native and Firebase—arguably the most dynamic duo in the app development sphere. Think of it as the perfect harmony of peanut butter and jelly, but infinitely geekier and far more rewarding!
So, make yourself comfortable, whether you’re indulging in a snack or sipping on that much-needed coffee boost, and join me as we embark on an exciting journey to construct amazing apps together.
What Is React Native? 🍉
Before we roll up our sleeves and jump into the code, let’s discuss React Native. Picture your favorite website; now imagine transforming that experience into a fully interactive mobile application! React Native is a framework crafted by Facebook that enables developers to create robust mobile applications using JavaScript and React—a popular library for building user interfaces.
The Marvel of Cross-Platform Development 🚀
Here’s the magic: with React Native, you write your code once, and it effortlessly runs on both iOS and Android platforms. That means no more painstakingly developing parallel apps for different operating systems! Imagine whipping up a fantastic meal and having everyone around you excitedly asking for a taste, all while only cooking one dish—now that’s efficient!
UI Components That Shine 🌟
React Native also offers a plethora of ready-made components, allowing developers to design stunning user interfaces without reinventing the wheel. With styling options akin to CSS and a variety of components at your disposal, your app’s aesthetic can shine just as brightly as its functionality.
Firebase: Your Best Friend In App Development 🤗
Now that you’re buzzing with excitement about React Native, let’s dive into Firebase—Google’s robust platform packed with essential tools for app development. Envision Firebase as your ultimate app Swiss Army knife, amalgamating everything from real-time databases to seamless authentication methods all in one neat package.
Why Choose Firebase?
Here are some standout reasons to incorporate Firebase into your development toolkit:
- Real-time Data Synchronization: Experience instant updates across devices; think of it as giving your users the latest updates with a mere flick of a switch!
- Simplified Authentication: Streamlining user logins is a breeze, eliminating headaches over tedious authentication processes.
- Seamless Scalability: Your app may start small, but with Firebase, it’s easy to grow bigger and better!
Firebase Firestore and Realtime Database: Understanding the Differences 🥨
Let’s decode the choices between Firebase Firestore and Realtime Database. You can think of Firestore as the sleek sportscar of databases—modern, high-performing, and designed for applications that demand rapid updates. In contrast, the Realtime Database is a dependable SUV—sturdy and perfect for scenarios where offline functionality is a must.
- Firestore: Offers a document-oriented structure, excellent for complex queries and robust indexing. This is best suited for advanced applications where efficiency is paramount.
- Realtime Database: Uses a JSON tree structure. It excels in straightforward, structured data scenarios—ideal for basic applications where real-time data syncing is key.
Enhancing Security with Firebase Authentication 🔒
In today’s digital landscape, data breaches are akin to uninvited party crashers. Fortunately, Firebase Authentication provides secure sign-in options, allowing users to log in effortlessly via social platforms (like Google or Facebook) or traditional email and password. Think of it as a VIP entrance that keeps your application safe and exclusive for authenticated users.
Getting Started: Setting Up Your Project 🚀
Excited to get your hands on some code? Here’s a concise step-by-step guide to kickstart your project.
Step 1: Establish the React Native Environment
You can choose between using Expo CLI or the more advanced React Native CLI to bootstrap your project. If you’re looking for a straightforward setup, Expo is highly recommended and user-friendly!
To create your new React Native app, simply run:
npx expo-cli init MyAwesomeApp
This command creates a fresh directory with your app name, making it super simple to get started!
Step 2: Integrate Firebase Into Your Project
Once your React Native environment is set up, it’s time to bring in Firebase. First, head over to the Firebase Console to create a new project. After setting things up, you’ll need to grab your Firebase configuration, which contains essential details about your project. Next, in your project directory, install the Firebase library by running:
npm install --save firebase
With just these initial steps, you’re well on your way to creating a powerful real-time mobile app!
What’s Next? 🔄
Now that you have the foundational elements in place, the next steps will involve diving deeper into coding, exploring components, setting up navigation, and managing state interactions. Indeed, building your real-time mobile app with React Native and Firebase opens doors to an exciting world of development possibilities!
Stay tuned for upcoming tutorials where we will deep dive into creating and managing database collections in Firestore, implementing real-time features, and much more. Happy coding! 🎉
Step 3: Firebase Configuration
Before you can utilize Firebase services in your application, you must properly configure Firebase. This involves setting up a connection between your app and your Firebase project. Here’s how to accomplish this in detail:
-
Create a Configuration File: Start by creating a new JavaScript file in your project root directory and name it
firebaseConfig.js
. This file will hold all the necessary configuration required to establish and manage your Firebase connection. -
Add Firebase SDK: Ensure you install the Firebase SDK if you haven't done so already. You can do this via npm (Node Package Manager) by running the following command in your terminal:
npm install firebase
-
Obtain Firebase Config Object: Go to your Firebase console (https://console.firebase.google.com/), select your project, and navigate to the project settings. Under the "General" tab, you'll find your Firebase configuration settings. Copy the config object, which should look something like this:
const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_SENDER_ID", appId: "YOUR_APP_ID" };
-
Setup Firebase in Your App: In your
firebaseConfig.js
file, paste the configuration you copied and structure your code as follows:import firebase from 'firebase/app'; import 'firebase/auth'; // Import Firebase Authentication import 'firebase/firestore'; // Import Firestore to handle database const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_SENDER_ID", appId: "YOUR_APP_ID" }; // Initialize Firebase only if it hasn’t been initialized yet if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); } // Export the firebase instance for use throughout your app export { firebase };
Step 4: Firebase Authentication
With Firebase configured, you can now implement user authentication. One common method is using email and password authentication. Below is a simplified example of how to set up user registration.
-
Registering a New User: In your application, you might need to allow users to create accounts. Use the following function to register a user with their email and password:
const registerUser = (email, password) => { firebase.auth().createUserWithEmailAndPassword(email, password) .then((userCredential) => { // User registered successfully console.log("User registered:", userCredential.user); }) .catch((error) => { // Handle registration errors here console.error("Error registering user:", error.message); }); };
This function uses the Firebase Authentication service to create a new user. If registration is successful, you can access the
userCredential
object to obtain information about the newly registered user.
Step 5: Firestore Integration
To leverage Firebase's Firestore for real-time database functionalities, you need to integrate Firestore into your application. This will enable you to perform CRUD (Create, Read, Update, Delete) operations on your database seamlessly.
-
Integrate Firestore into Your Configuration: Return to the
firebaseConfig.js
file you created earlier. Add the following line to initialize Firestore:const firestore = firebase.firestore();
This line of code creates a reference to the Firestore service, allowing you to interact with the database.
-
CRUD Operations: Now that Firestore is integrated, you can begin performing various data operations. Here's a brief overview of each CRUD operation:
-
Create: To add a new document to a Firestore collection:
const addDocument = async (data) => { try { const docRef = await firestore.collection('yourCollectionName').add(data); console.log("Document written with ID: ", docRef.id); } catch (error) { console.error("Error adding document: ", error); } };
-
Read: To read documents from a collection:
const fetchDocuments = async () => { const querySnapshot = await firestore.collection('yourCollectionName').get(); querySnapshot.forEach((doc) => { console.log(`${doc.id} => `, doc.data()); }); };
-
Update: To update an existing document:
const updateDocument = async (id, newData) => { try { await firestore.collection('yourCollectionName').doc(id).update(newData); console.log("Document updated successfully"); } catch (error) { console.error("Error updating document: ", error); } };
-
Delete: To delete a document:
const deleteDocument = async (id) => { try { await firestore.collection('yourCollectionName').doc(id).delete(); console.log("Document deleted successfully"); } catch (error) { console.error("Error deleting document: ", error); } };
-
With these foundational steps in place, your application is now configured to harness the power of Firebase for user authentication and real-time database interactions, effectively preparing you for further development and enhancements!
Certainly! Let's expand upon and refine the provided content, ensuring clear explanations and detailed insights into each section while maintaining a cohesive flow.
Adding Data to Firestore
const addData = async (collectionName, data) => {
await firestore.collection(collectionName).add(data);
};
In this snippet, we define an asynchronous function named addData. This function takes two parameters: collectionName
, representing the name of the Firestore collection where we want to store the data, and data
, which is the object containing the information we wish to add. The method firestore.collection(collectionName).add(data)
facilitates the addition of the data to the specified collection in Firestore.
Error Handling
To ensure robustness, consider adding error handling to manage any potential issues that may arise during the data submission process. Here's an improved version:
const addData = async (collectionName, data) => {
try {
await firestore.collection(collectionName).add(data);
console.log("Data added successfully!");
} catch (error) {
console.error("Error adding data: ", error);
}
};
Step 6: Implementing Real-Time Updates 🌟
To enhance your app's interactivity with real-time updates, Firestore provides an elegant solution through document listeners. Setting up listeners allows your application to automatically receive updates when the data changes. Here's a sample implementation:
const unsubscribe = firestore.collection("yourCollection").onSnapshot((snapshot) => {
snapshot.docChanges().forEach((change) => {
if (change.type === "added") {
console.log("New document added: ", change.doc.data());
} else if (change.type === "modified") {
console.log("Document modified: ", change.doc.data());
} else if (change.type === "removed") {
console.log("Document removed: ", change.doc.data());
}
});
});
This onSnapshot
listener observes changes to the collection specified. It uses snapshot.docChanges()
to iterate through each change. This approach ensures you're always working with the most current data. Don't forget to unsubscribe from the listener when it's no longer needed:
// Call this when you're done with the listener
unsubscribe();
Enhancing UI Design with Style 🎨
With your backend infrastructure up and running, it’s time to delve into the aesthetics of your application. A visually appealing interface is pivotal for user engagement. Utilizing component libraries like React Native Paper or Native Base can significantly simplify the UI development process, allowing you to create beautiful, consistent components with minimal effort.
Best Practices for Web Design
If you venture into crafting sophisticated applications such as e-commerce platforms, collaborating with a premier web design company in Mumbai can make a world of difference. These professionals specialize in delivering stellar ecommerce website design in Mumbai, ensuring your application is not only visually stunning but also user-friendly.
Prateeksha Web Design: Your Creative Ally 🌟
When searching for a dependable web development company, Prateeksha Web Design stands out as a beacon of creativity and functionality. They specialize in a diverse range of web design services in Mumbai, adept at transforming your concepts into reality, from simple landing pages to comprehensive ecommerce website development in Mumbai.
Testing Your Application 📱
Testing is an indispensable part of the development lifecycle. It acts as a safeguard, ensuring that your application performs as expected across various scenarios. Utilizing robust testing libraries such as Jest alongside React Native Testing Library can help identify and rectify bugs before they disrupt the user experience.
Ensuring Responsiveness
In today's world, users access applications on a myriad of devices. It is crucial to ensure that your app is responsive across all screen sizes and resolutions. Employing CSS techniques like Flexbox will assist in creating a fluid layout that adapts gracefully. Rigorous testing on various devices guarantees a seamless user experience.
Deploying Your Real-Time Application 🌐
With development complete, the next exciting phase is deployment! For mobile applications built with React Native, you will typically upload your app to the App Store and Google Play. Be sure to meticulously follow their guidelines to ensure a smooth submission and approval process.
Post-Launch: Keeping Your App Fresh 🍃
Just like a fitness regime, your app requires continual maintenance and updates. Actively listen to user feedback, fix any bugs, and refresh the content to keep your application relevant and engaging. This commitment will go a long way in retaining users and enhancing their experience.
Leveraging Analytics
Integrating Firebase Analytics is key to understanding user behavior in-depth. This tool provides valuable insights, akin to having a backstage pass to your audience's preferences and interests, enabling you to tailor your applications to meet their needs effectively.
Final Thoughts & Next Steps 🌈
Congratulations! You've now navigated through the essentials of building a real-time mobile app using React Native and Firebase. Remember, the journey will feature both challenges and triumphs, contributing to your growth as a developer.
So, gear up, crank up that coding playlist, and dive into the exciting world of app development! Remember, every expert started as a novice—embrace the journey, learn actively, and persist through adversity.
Lastly, when you’re ready to elevate your project or seek innovative design solutions, don’t hesitate to contact Prateeksha Web Design. They are not merely a web design agency in Mumbai; they are your partners in creativity and innovation, ready to transform your vision into reality.
With these expansions and refinements, your content is now detailed, engaging, and informative, providing a comprehensive overview of developing and launching a real-time mobile application.
Tip
Utilize Firebase's Firestore for Real-Time Updates: When building your app, leverage Firestore's real-time capabilities to synchronize data across devices seamlessly. This ensures that users receive immediate updates, enhancing the overall user experience. Implement efficient data fetching and consider pagination to manage data flow effectively.
Fact
Firebase and React Native Integration: Firebase provides robust support for React Native, allowing developers to use a variety of Firebase services, such as Authentication, Cloud Firestore, and Crashlytics, directly within their mobile applications. This integration simplifies backend support and accelerates app development.
Warning
Be Cautious with Data Security Rules: While Firebase offers flexible data storage solutions, it’s crucial to set up proper security rules in Firestore to prevent unauthorized access to your data. Improperly configured rules can lead to data breaches or loss of user privacy. Always test your security rules thoroughly before deploying your app to production.
About Prateeksha Web Design
Prateeksha Web Design specializes in creating real-time mobile applications using Firebase and React Native, offering tailored solutions for developers. Their services include seamless integration of Firebase's backend capabilities to enhance app functionality and real-time data synchronization. With expert guidance in React Native, they enable rapid development and cross-platform compatibility. Additionally, they provide comprehensive support from concept to deployment, ensuring a smooth development experience. Ideal for program geeks, their approach combines technical expertise with innovative design to deliver outstanding mobile applications.
Interested in learning more? Contact us today.
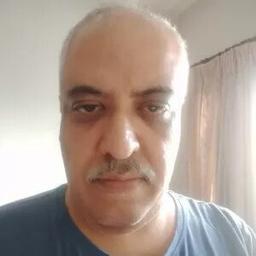