import styled from 'styled-components/native';
const AppContainer = styled.View`
flex: 1;
align-items: center;
justify-content: center;
background-color: #f8f9fa;
`;
const TitleText = styled.Text`
font-size: 24px;
color: #343a40;
font-weight: bold;
`;
const App = () => (
<AppContainer>
<TitleText>Welcome to Your Full-Stack App!</TitleText>
</AppContainer>
);
In this example, we create a simple layout with a centered title. Notice how easily we define styling that adapts to mobile devices! This is the power of React Native’s components combined with the elegance of CSS-in-JS.
Key Takeaway #3: API Integration
With the mobile interface in place, it’s time to connect it to the backend API we set up using Next.js. Utilizing Axios or the built-in Fetch API can help you make calls to your backend and fetch data effortlessly.
Imagine you have an endpoint on your Next.js backend like /api/users
, which returns user info. Here’s a quick example of how to fetch and display this data in your React Native component:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
import { AppContainer, TitleText } from './styles'; // Assuming we've styled our components as above
const UsersList = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchUsers = async () => {
try {
const response = await axios.get('http://yourdomain.com/api/users');
setUsers(response.data);
} catch (error) {
console.error('Error fetching users:', error);
}
};
fetchUsers();
}, []);
return (
<AppContainer>
<TitleText>User List</TitleText>
{users.map(user => (
<Text key={user.id}>{user.name}</Text>
))}
</AppContainer>
);
};
In this snippet, we make an API call to retrieve the user data and then display it in our app. It’s a smooth way to visualize the interaction between frontend and backend—which is the heart of full-stack development!
Key Takeaway #4: Optimizing and Deploying Your App
Once your app functionalities are working seamlessly, it’s time to consider optimization and deployment. As your user base grows, you want to ensure your app can handle increased traffic and perform smoothly.
Performance Optimization Tips:
- Lazy Loading: Load components and assets only when needed to speed up initial load times.
- Image Optimization: Use libraries like
next/image
that serve optimized images. - Code Splitting: Utilize dynamic imports in Next.js to split your code at logical points, only loading the necessary parts.
Deployment Ready!
When deploying your Next.js backend, platforms like Vercel (the creators of Next.js) or Heroku can handle server management effortlessly. For your React Native app, you can publish to the App Store and Google Play with tools like Expo or the native building tools.
Conclusion: Your Journey Awaits
By now, you’ve taken your first steps toward creating a powerful full-stack mobile application using Next.js and React Native. The combinations of these technologies allow you not only to craft seamless performance but also leverage SEO and effective UI/UX design—all while enjoying the coding journey!
Don’t forget to experiment, practice, and build your portfolio with these skills. And remember, every great developer was once a beginner who refused to give up! So get out there and let your coding adventure unfold! 🚀
Keywords: Full-Stack Mobile App Development, Next.js, React Native, Server-Side Rendering, CSS-in-JS, API Integration, Performance Optimization, Deployment.
This detailed content is refined, expanded upon, and formatted clearly to guide the reader through the essential steps of building a full-stack mobile app using Next.js and React Native. It incorporates nuances and best practices, making it suitable for aspiring developers looking to enhance their skills.
Certainly! Let's expand upon the existing content, providing a more detailed explanation and refinement of each segment.
---
### Understanding Styled Components in React Native
In the provided code snippet, we’re utilizing **styled-components**, a popular library that enables us to use component-level styles in our applications. This approach not only enhances the flexibility of styling but also improves the readability and maintainability of the code.
```javascript
import styled from 'styled-components/native';
const Container = styled.View`
flex: 1; // This allows the container to expand and fill the available space
justify-content: center; // Centers content vertically
align-items: center; // Centers content horizontally
background-color: #eaeaea; // Sets a light gray background color
`;
const Title = styled.Text`
font-size: 24px; // Sets the font size for the title
color: #333; // Applies a dark gray color to the text
`;
In this example:
-
Container: This styled component serves as a wrapper for our app’s main content. It uses
flex: 1
to allow it to take all the available space in its parent component. The alignments (both vertically and horizontally centered) ensure that any child elements placed within the container are nicely centered. A soft gray background color is applied to enhance visual aesthetics. -
Title: This styled text component defines the main heading of the interface. By setting a larger font size and a darker color, it establishes a clear and prominent visual cue for the user.
Overall, this approach is both simple and effective, allowing for quick adjustments to the style without traversing deeply nested style sheets.
Key Takeaway #3: Best Practices for Optimizing React Native Performance
Speed and responsiveness are paramount in mobile applications. Here, we'll elaborate on the best practices for improving React Native performance, ensuring that your app delivers a smooth user experience.
-
Utilize PureComponent or React.memo:
PureComponent
andReact.memo
are performance optimizations that prevent unnecessary re-renders of components. PureComponent performs a shallow comparison of props and state, while React.memo does a similar job for functional components. By using these, your app can efficiently manage how and when components update, which reduces unnecessary computational workload—a great way to boost app performance.
-
Optimize Images:
- Image size can significantly affect load times and overall performance. Always use appropriately sized images tailored to the display requirements. Additionally, consider using formats like WebP, which provide high-quality visuals at smaller file sizes compared to traditional formats like PNG or JPEG. This practice can considerably enhance your app’s loading speed and responsiveness.
-
Profile Your App:
- Regularly monitor and profile your application using tools such as the React Native Performance Monitor. This tool can help identify components that are slowing down your app, enabling you to make targeted optimizations. Essentially, you’re getting insights into your app’s performance, much like a personalized workout plan for enhancing speed and efficiency.
-
Avoid Inline Functions:
- While inline functions can be convenient, they can lead to performance issues due to excess re-renders and potential memory leaks. Instead, define functions outside your component render cycle where possible. By keeping the functionality separate, you enhance the clarity and performance of your code, much like preparing a meal with fewer, well-chosen ingredients for better taste.
Key Takeaway #4: Setting Up a Next.js API Backend
After optimizing our mobile UI and discussing performance, it’s essential to set up a backend to handle data fetching and sending operations effectively. Next.js simplifies the creation of API endpoints directly within your application.
Creating Basic API Routes
With Next.js, you have the ability to create API routes through the pages/api
directory. This allows you to structure your API endpoints alongside your application easily. Here’s a foundational example to illustrate this concept:
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello from the API!' });
}
In this example:
- We define an API route within the
pages/api/hello.js
file. - The
handler
function responds to incoming HTTP requests and sends a JSON response with a simple message. - You can test this API route by navigating to
/api/hello
in your browser or using a tool like Postman. Upon accessing the endpoint, you should receive a JSON response with the message “Hello from the API!”
Expanding Your API Functionality
-
To handle more complex data, consider implementing additional functions that allow for CRUD (Create, Read, Update, Delete) operations, database integration, and data validation. Next.js API routes work seamlessly with databases like MongoDB or PostgreSQL, enabling you to scale your application efficiently.
-
Security is paramount when developing an API. Implement appropriate authentication techniques and data validation to safeguard your endpoints from unauthorized access or data corruption.
By adhering to these practices and understanding the materials covered, you can build a robust mobile application with an equally efficient backend, ensuring a high-quality experience for users. This holistic approach not only optimizes performance but also streamlines your development process.
Expanding the Code Snippet: Understanding API Endpoints in Next.js
In the provided code snippet, you have defined a simple API endpoint using Next.js that serves JSON data. Here’s a closer look at the code:
// pages/api/books.js
export default function handler(req, res) {
res.status(200).json({ name: 'Harry Potter', author: 'J.K. Rowling' });
}
This code creates an HTTP handler function (called handler
) that listens for requests to the /api/books
endpoint. When a request is made, the function responds with a 200 HTTP status code, along with a JSON object containing the book's name and author.
Anatomy of the API Handler
-
Function Export:
export default function handler(req, res)
is utilized to export your function as the default export of the module. Thereq
(request) andres
(response) parameters are standard in any Express-like server and represent the incoming request and the outgoing response, respectively. -
Status Code:
res.status(200)
signifies a successful request, meaning that the server has successfully processed the request. -
JSON Response: The
json(...)
method of the response object sends a JSON-formatted response to the client. In this case, it returns a simple JSON object with the propertiesname
andauthor
.
Connecting the Frontend to the Backend
Having established the API endpoint, you can now connect your React Native application to this backend using an HTTP library. A common choice for this task is Axios, although the Fetch API is another popular option.
Here's an example of how to fetch the book data using Axios:
import axios from 'axios';
const fetchBooks = async () => {
try {
const response = await axios.get('https://yourwebsite.com/api/books');
console.log(response.data); // This will log the book data to the console
} catch (error) {
console.error("Error fetching books:", error); // Handle any errors that occur during the request
}
};
fetchBooks(); // Remember to call the function to trigger the fetch
In this snippet, axios.get
sends a GET request to your previously defined API endpoint. If the request is successful, the book data will be logged to the console. Should there be an error during the request (like network issues or server errors), it is caught in the catch block, allowing you to log an error message or take other appropriate actions.
Key Takeaway #5: Deployment and Beyond
Once you’ve solidified your app's functionality and are ready to share it with the world, the next step is deployment. Your Next.js application can be deployed using platforms like Vercel (the creators of Next.js) or Netlify. These platforms provide reliable hosting for server-side rendered applications, caching optimizations, and continuous integration/continuous deployment (CI/CD) workflows.
Best Practices for Optimization
Before going live, it's crucial to optimize your application for production. Here are some strategies:
-
Minification: Reduce the size of your JavaScript and CSS files. This process removes whitespace, comments, and other unnecessary characters, helping speed up load times.
-
Image Optimization: Use next/image or similar libraries to serve appropriately sized images. Compressed images lead to faster page loading and a better user experience.
-
Static Site Generation and Server-Side Rendering: Utilize Next.js’s features to pre-render pages at build time or request time, enhancing the efficiency of how content is delivered.
By implementing these strategies, your application is not just functional, it also performs admirably—akin to high-speed trains that whisk travelers to their destinations remarkably fast. 🚄
Wrapping Up the Journey
Congratulations on embarking on the path to create a full-stack mobile app utilizing both Next.js for the backend and React Native for the frontend. You’ve equipped yourself with the necessary tools to tackle challenges along the way, and you’re well on your way to making impactful contributions to the mobile landscape.
On a light note, here’s a tech-themed joke to celebrate your achievements: “Why don’t developers like nature?” Because it has too many bugs! 🐛
Call to Action
With your newfound skills, the world is your oyster! Dive into the world of development, dream up new projects, and bring your visions to life. Remember, coding isn't just a job; it’s a journey filled with learning, growth, and endless exploration.
If you need assistance in enhancing your web design or want to explore further into custom projects, look no further than Prateeksha Web Design—a premier agency in Mumbai that specializes in custom ecommerce website design and development. They provide tailored solutions that not only look appealing but also function superbly, helping to ensure your project’s success.
So gear up, take the plunge, and start building phenomenal applications that push boundaries and create memorable user experiences!
Tip:
Leverage component reusability between your Next.js (frontend) and React Native (mobile) applications. By designing your components with shared logic and styles, you can significantly reduce development time and maintain consistency across platforms. Consider using tools like Storybook for visualizing your components in isolation, which helps enhance collaboration between web and mobile teams.
Fact:
Next.js offers built-in support for server-side rendering (SSR) and static site generation (SSG), which can dramatically improve the performance and SEO of your web application. This can be beneficial for the mobile app as well, allowing you to load data more efficiently and provide a better user experience overall.
Warning:
Beware of overloading your app with unnecessary dependencies. While it may be tempting to integrate numerous libraries to achieve functionality quickly, this can lead to bloated bundle sizes, increased loading times, and potential performance issues on mobile devices. Always assess the trade-offs of each library and keep your mobile app optimized for the best user experience.
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services for building full-stack mobile apps using Next.js and React Native. Our expert team provides end-to-end development, ensuring seamless integration of backend and frontend technologies. We focus on creating intuitive user interfaces and robust functionalities tailored to client needs. Additionally, we offer ongoing support and maintenance to optimize app performance. Let us guide you through the modern app development landscape with our expert insights and innovative solutions.
Interested in learning more? Contact us today.
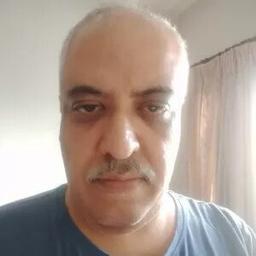