So, you’ve heard the buzz about Next.js, and you’re thinking, "What’s the big deal? Another JavaScript framework?" Well, buckle up, because Next.js isn’t just another framework—it’s the VIP pass to building fast, SEO-friendly, and modern web applications. Whether you're a student, a developer, or someone just curious about what this "Next.js tutorial" thing is, you're in for a treat.
In this blog, we'll take you from zero to hero, covering everything from installing Next.js to deploying Next.js like a pro. And don’t worry, I’ll keep it light, fun, and beginner-friendly. Let’s dive in!
What is Next.js, and Why Should You Care?
When it comes to building websites, speed, performance, and user experience are king. Enter Next.js, a powerful framework that ensures your website isn't just a digital placeholder but a high-performing, user-friendly platform that stands out. Let’s break it down so you understand why Next.js is a game-changer.
What is Next.js?
At its core, Next.js is a React-based framework developed by Vercel. Think of React as the toolbox for creating interactive web interfaces. Next.js takes that toolbox and adds superpowers, making it easier to build complex applications without sacrificing performance.
Here’s what makes it special:
-
Server-Side Rendering (SSR):
Normally, when a browser requests a web page, it fetches a blank shell and loads the JavaScript to populate it. With SSR, Next.js pre-renders the page on the server, delivering a complete, ready-to-view page to the browser. This reduces load times significantly. -
Static Site Generation (SSG):
Next.js can pre-generate HTML files at build time. This is perfect for pages that don’t change frequently, like blogs or product catalogs. It combines the speed of static files with the flexibility of dynamic rendering. -
Hybrid Rendering:
Why choose between SSR and SSG when you can have both? Next.js allows you to mix and match, giving you the freedom to decide how each page should be rendered based on your needs.
Why Should You Care?
Let’s face it: Building and maintaining a modern website can feel like juggling flaming torches. Next.js makes it easier by addressing common pain points. Here’s why you should consider adding it to your tech stack:
1. SEO-Friendly
Search engines like Google love fast, pre-rendered pages. With SSR and SSG, Next.js ensures your content is available for indexing right out of the gate. No more worrying about bots getting stuck on JavaScript-heavy pages. Your pages are optimized for search engines, helping you rank higher.
2. Performance Boost
Nobody likes waiting for a website to load. Thanks to features like automatic code splitting (loading only what’s needed for a specific page) and lazy loading (loading images or components only when they appear on-screen), Next.js ensures your site is lightning-fast.
3. Flexibility for Any Project
From personal blogs to large-scale e-commerce platforms, Next.js has tools to cater to every type of project.
- Building a Blog? Use static generation for fast-loading pages.
- Launching an Online Store? Implement SSR for dynamic, frequently updated product pages.
- Corporate Dashboards? Mix static and server-rendered content for optimal performance.
4. Developer-Friendly Features
Next.js is like a developer’s dream come true. It comes with:
- Hot Module Replacement (HMR): See your changes live without refreshing.
- API Routes: Build backend functionality right in the same framework.
- Built-in CSS Support: No need to wrestle with external stylesheets unless you want to.
- File-Based Routing: Create routes just by adding files to the
pages
folder.
5. Strong Community and Ecosystem
Next.js is backed by Vercel, a company deeply invested in the framework’s success. You’ll find extensive documentation, a growing library of plugins, and a vibrant developer community. Whether you’re stuck on a bug or looking for inspiration, help is always just a click away.
Why Prateeksha Web Design Loves Next.js
At Prateeksha Web Design, we’ve worked with countless technologies, and Next.js consistently impresses us. Here’s why it’s one of our go-to frameworks:
- Custom Website Development: Next.js lets us create unique, tailored experiences for our clients, from small businesses to large enterprises.
- Performance Optimization: Whether it’s lazy loading or SSR, we leverage Next.js’s features to deliver blazing-fast websites.
- Scalable Solutions: Our clients often grow, and Next.js provides the flexibility to scale applications without major overhauls.
When we use Next.js for our projects, we know we’re delivering not just a website but a high-performance tool that boosts our clients’ online presence.
Step 1: Installing Next.js Like a Pro
Getting started with Next.js is like setting up your favorite gaming console—easy and exciting. If you’ve installed a Node.js app before, this will feel like a breeze. If not, don’t worry—I’ll guide you step by step.
Let’s set the stage for your Next.js adventure.
What You Need Before We Start
-
Node.js
Next.js relies on Node.js to run. If you don’t have it installed yet, grab the latest stable version (14 or later) from Node.js. Why Node.js? It allows your computer to execute JavaScript code outside of a browser, which is essential for modern web development. -
Code Editor
Writing code in a basic text editor is like drawing with crayons when you could use a professional sketchpad. Download Visual Studio Code (VS Code), a free, versatile, and widely loved code editor.
Installation Steps
Now that you’re armed with the essentials, let’s dive into the actual setup process.
Step 1: Create Your Project Folder
-
Open your terminal (Mac/Linux) or command prompt (Windows).
-
Run the following command:
npx create-next-app@latest my-nextjs-app
What’s happening here?
npx
: A package runner that downloads and executes create-next-app without permanently installing it.create-next-app@latest
: Fetches the latest version of Next.js.my-nextjs-app
: This will be the name of your project folder.
-
Once the setup wizard completes, navigate into your new project folder:
cd my-nextjs-app
-
Quick Checkpoint: Your project folder should now contain files and folders like
pages
,styles
, andpackage.json
.
Step 2: Install Dependencies
After creating the project, the wizard installs the necessary dependencies automatically. If you’re curious, these include React, React DOM, and Next.js.
Want to see your app in action? Type:
npm run dev
This starts a local development server. Open your browser and go to:
http://localhost:3000
You’ll see a default Next.js welcome page with the message: “Welcome to Next.js!” 🎉
Step 3: Customize Your App
Now that your app is live, it’s time to make it yours. Open the project in VS Code and explore the files:
pages
Folder: This is the heart of your app. Each file here represents a route (e.g.,index.js
maps to/
andabout.js
maps to/about
).styles
Folder: Contains global and modular CSS files for styling.
Tip: Change the title in pages/index.js
to see your first edit in action.
Step 2: Building Cool Stuff with Next.js
Congratulations! You’ve successfully installed Next.js. Now, let’s unlock its real potential.
Understanding Pages and Routing
Next.js uses a file-based routing system, which means every file in the pages
folder automatically becomes a route. No need to mess around with react-router
or similar tools—it’s all built-in!
Example 1: Creating a Static Page
Let’s create an About Us page:
- In the
pages
folder, create a new file calledabout.js
. - Add the following code:
export default function About() { return <h1>Welcome to the About Page!</h1>; }
- Save the file and navigate to
http://localhost:3000/about
in your browser.
What Just Happened?
The about.js
file you created was automatically picked up by Next.js and served as the /about
route. Easy, right?
Adding Dynamic Routes
Static routes are great, but what if you want a page for every blog post or product? That’s where dynamic routes come in.
Example 2: Creating a Dynamic Blog Post Page
-
In the
pages
folder, create a new folder calledblog
. Inside it, create a file named[slug].js
. -
Add the following code:
import { useRouter } from 'next/router'; export default function BlogPost() { const router = useRouter(); const { slug } = router.query; return <h1>Blog Post: {slug}</h1>; }
-
Save the file and navigate to a URL like
http://localhost:3000/blog/my-first-post
.
What’s Happening Here?
- The
[slug].js
file acts as a placeholder for dynamic URLs. - The
useRouter
hook retrieves the dynamic part of the URL (slug
). - This approach is perfect for blogs, products, or anything with unique IDs or titles.
Step 3: Styling Your Next.js App
Imagine serving a perfectly baked cupcake—without frosting. It’s functional but lacks the wow factor. Similarly, a Next.js app without styling might work, but it won’t make anyone’s jaw drop. Let’s dive into how you can style your app to make it visually appealing and unique.
Global Styles: A Unified Look Across Your App
Global styles are applied to the entire app, ensuring a consistent look and feel across all pages.
How to Add Global Styles:
-
Open the
styles/globals.css
file in your project. If it doesn’t exist, create one in thestyles
folder. -
Add your global CSS:
body { font-family: Arial, sans-serif; margin: 0; padding: 0; background-color: #f0f0f0; } h1, h2, h3, h4, h5, h6 { color: #333; } a { text-decoration: none; color: #0070f3; } a:hover { text-decoration: underline; }
-
Import the
globals.css
file in yourpages/_app.js
:import '../styles/globals.css'; export default function App({ Component, pageProps }) { return <Component {...pageProps} />; }
Why Use Global Styles?
They’re perfect for resetting default browser styles and applying universal design elements like fonts and colors.
CSS Modules: Component-Specific Styling
CSS Modules let you scope styles to individual components, avoiding conflicts across your app.
How to Use CSS Modules:
-
Create a CSS module file in the
styles
folder. For example,Home.module.css
:.title { color: blue; font-size: 2rem; text-align: center; } .description { color: gray; font-size: 1.2rem; line-height: 1.6; }
-
Import the module in your component:
import styles from '../styles/Home.module.css'; export default function Home() { return ( <div> <h1 className={styles.title}>Welcome to Next.js</h1> <p className={styles.description}> Next.js is awesome for building modern web apps! </p> </div> ); }
Advantages of CSS Modules:
- Scoped styles: No worries about accidentally overwriting styles elsewhere.
- Simplicity: Easy to implement and maintain.
Other Styling Options in Next.js
Next.js supports various styling approaches, giving you the flexibility to choose what works best for your project.
1. Styled JSX:
Styled JSX is built into Next.js, allowing you to write scoped styles directly in your components.
Example:
export default function Home() {
return (
<div>
<h1>Welcome to Next.js</h1>
<style jsx>{`
h1 {
color: green;
text-align: center;
}
`}</style>
</div>
);
}
2. Tailwind CSS:
Want a utility-first CSS framework? Integrate Tailwind CSS into your Next.js app for rapid development.
To install:
npm install tailwindcss postcss autoprefixer
npx tailwindcss init
Then, configure your project to use Tailwind classes.
3. CSS-in-JS Libraries:
Libraries like Emotion and Styled-Components provide a modern, dynamic approach to styling components.
Step 4: Deploying Next.js to the World
Once your app is styled to perfection, it’s time to share it with the world. Deployment might sound intimidating, but with Next.js, it’s a piece of cake.
Deploying on Vercel
Since Vercel is the team behind Next.js, it’s the easiest and most optimized platform for deployment.
Steps to Deploy on Vercel:
-
Install Vercel CLI
Open your terminal and install the Vercel CLI:npm i -g vercel
-
Login to Vercel
Run the login command to connect your account:vercel login
-
Deploy Your App
Inside your project directory, type:vercel
Follow the prompts, and your app will be deployed to a live URL (e.g.,
https://your-app-name.vercel.app
).
Other Hosting Options
If Vercel isn’t your thing, you can deploy your app on other platforms:
1. Netlify
- Perfect for static sites or hybrid apps.
- Install Netlify CLI and run
netlify deploy
.
2. AWS Amplify
- Enterprise-grade hosting for modern apps.
- Offers scalability and integration with AWS services.
3. Self-Hosting
- For those who prefer control.
- Use Docker, Node.js, or a hosting provider like DigitalOcean.
Why Deployment is Important
Deployment isn’t just about making your app live—it’s about scalability, reliability, and performance. With platforms like Vercel, you also get features like:
- Continuous Deployment (CD): Automatically deploy updates from your Git repository.
- Built-in analytics: Monitor app performance effortlessly.
Pro Tips to Level Up Your Next.js Skills
Once you’ve mastered the basics of Next.js, it’s time to kick things up a notch. The beauty of Next.js lies in its ability to cater to both beginners and advanced developers with features that streamline workflows and enhance performance. Here are some pro tips to help you make the most out of your Next.js projects.
1. Use API Routes: Build Serverless APIs Without a Backend
Next.js comes with built-in support for serverless API routes, eliminating the need for a separate backend. You can define APIs directly in the pages/api
folder.
How to Create an API Route:
-
Inside the
pages/api
folder, create a file calledhello.js
. -
Add the following code:
export default function handler(req, res) { res.status(200).json({ message: 'Hello, Next.js API!' }); }
-
Start your server (
npm run dev
) and visithttp://localhost:3000/api/hello
. You’ll see the JSON response:{ "message": "Hello, Next.js API!" }
Why It’s Awesome:
- Perfect for handling form submissions, fetching external data, or building lightweight APIs.
- Scales effortlessly on platforms like Vercel.
2. Explore Image Optimization: Use the <Image>
Component
Images can make or break your app’s performance. Next.js offers a built-in <Image>
component that automatically optimizes images for better loading times and user experience.
How to Use the <Image>
Component:
-
Import the
Image
component:import Image from 'next/image';
-
Add an optimized image to your page:
export default function Home() { return ( <div> <h1>Optimized Images in Next.js</h1> <Image src="/example.jpg" // Replace with your image path alt="Example Image" width={600} height={400} /> </div> ); }
Key Features:
- Automatic Resizing: Serve images in sizes appropriate for different devices.
- Lazy Loading: Load images only when they enter the viewport.
- Built-in Caching: Speeds up repeat visits.
Pro Tip: Use external image domains by configuring next.config.js
:
module.exports = {
images: {
domains: ['your-image-domain.com'],
},
};
3. Play with Middleware: Add Custom Functionality to Requests
Middleware in Next.js lets you execute custom code before a request is processed. This is especially useful for handling authentication, redirects, or localization.
How to Implement Middleware:
- Create a
_middleware.js
file in yourpages
folder or its subdirectories. - Add your custom logic:
import { NextResponse } from 'next/server'; export function middleware(req) { const url = req.nextUrl; // Example: Redirect users not logged in if (!url.searchParams.get('loggedIn')) { return NextResponse.redirect('/login'); } return NextResponse.next(); }
Why Middleware Rocks:
- Flexibility: Intercept and manipulate requests dynamically.
- Performance: Middleware runs on the edge, making it super fast.
- Use Cases: Authentication, feature toggles, A/B testing, and more.
4. Leverage Plugins to Supercharge Your App
Next.js integrates seamlessly with plugins to add advanced features like analytics, SEO, and state management.
Recommended Plugins:
-
Next SEO: Manage meta tags, Open Graph, and structured data.
npm install next-seo
Example usage:
import { NextSeo } from 'next-seo'; export default function Home() { return ( <> <NextSeo title="Next.js Pro Tips" description="Level up your Next.js skills with these expert tips!" /> <h1>Welcome to Pro Tips!</h1> </> ); }
-
@next/font: Add custom fonts to your app with zero layout shift.
Example:import { Roboto } from '@next/font/google'; const roboto = Roboto({ subsets: ['latin'], weight: ['400', '700'], }); export default function Home() { return <h1 style={{ fontFamily: roboto.style.fontFamily }}>Hello, Next.js!</h1>; }
-
Redux Toolkit: For managing state in complex applications.
Install Redux and its Toolkit:npm install @reduxjs/toolkit react-redux
Why These Pro Tips Matter
These features aren’t just bells and whistles—they’re tools that elevate your app to a professional level. Whether you’re building a small portfolio or a large-scale enterprise solution, these tips can:
- Improve performance and scalability.
- Save time by leveraging Next.js’s built-in features.
- Create user-friendly, SEO-optimized, and visually stunning apps.
Wrapping It Up
Congratulations! You’ve just taken your first steps into the Next.js universe. Whether you’re installing Next.js for the first time or deploying Next.js to the world, remember—it’s all about experimenting, learning, and having fun.
At Prateeksha Web Design, we’ve built countless stunning websites with Next.js, blending creativity and technical expertise to deliver outstanding results. If you ever feel stuck, our team is here to guide you every step of the way.
Now, it’s your turn to build something amazing. Go ahead, try it out, and share your creations. The world is waiting for your masterpiece!
Call to Action: Ready to elevate your web development game? Dive deeper into the world of Next.js by exploring our blogs and tutorials. If you need a hand, let Prateeksha Web Design be your trusted partner in bringing your ideas to life. Let’s create something extraordinary together!
About Prateeksha Web Design
Prateeksha Web Design offers comprehensive services tailored for mastering Next.js, including step-by-step installation assistance, intuitive tutorials on core features, and best practices for efficient development. Our expert team provides hands-on guidance for building scalable applications and ensures seamless deployment strategies. We also offer personalized support for troubleshooting and optimization, empowering beginners to confidently navigate their Next.js journey. Enroll in our engaging workshops and access valuable resources to enhance your skills. Transform your web development experience with Prateeksha Web Design today!
Interested in learning more? Contact us today.
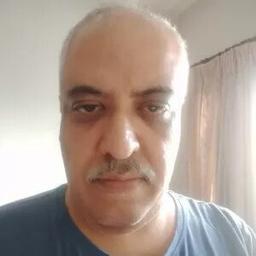